pthread_cond_timewait用例
时间: 2023-11-04 19:53:18 浏览: 64
pthread_cond_timedwait函数是一个等待特定条件变量的函数,它允许线程在指定时间内等待条件变量的满足。函数原型如下:
```c
int pthread_cond_timedwait(pthread_cond_t *restrict cond, pthread_mutex_t *restrict mutex, const struct timespec *restrict abstime);
```
参数说明:
- cond:指向条件变量的指针。
- mutex:指向互斥锁的指针。在调用该函数前,必须已经持有这个互斥锁。
- abstime:指向保存等待时间的timespec结构体的指针,表示等待的绝对时间。
函数返回值为0表示成功,非零值表示失败。
以下是一个使用pthread_cond_timedwait的示例代码:
```c
#include <stdio.h>
#include <pthread.h>
#include <time.h>
pthread_mutex_t mutex;
pthread_cond_t cond;
void* thread_func(void* arg) {
printf("Child thread waiting for condition...\n");
struct timespec wait_time;
clock_gettime(CLOCK_REALTIME, &wait_time);
wait_time.tv_sec += 5; // 等待5秒钟
pthread_mutex_lock(&mutex);
int ret = pthread_cond_timedwait(&cond, &mutex, &wait_time);
if (ret == 0) {
printf("Condition signaled!\n");
} else if (ret == ETIMEDOUT) {
printf("Timeout occurred!\n");
} else {
printf("Error occurred while waiting for condition\n");
}
pthread_mutex_unlock(&mutex);
return NULL;
}
int main() {
pthread_t tid;
pthread_mutex_init(&mutex, NULL);
pthread_cond_init(&cond, NULL);
pthread_create(&tid, NULL, thread_func, NULL);
sleep(3); // 保证子线程在等待之前先运行
pthread_mutex_lock(&mutex);
printf("Main thread signaling condition...\n");
pthread_cond_signal(&cond);
pthread_mutex_unlock(&mutex);
pthread_join(tid, NULL);
pthread_cond_destroy(&cond);
pthread_mutex_destroy(&mutex);
return 0;
}
```
上述代码中,主线程创建了一个子线程,子线程调用pthread_cond_timedwait函数等待条件变量的满足。主线程在等待3秒后发出信号,并通知子线程条件已满足。如果在等待时间内收到信号,则打印"Condition signaled!",否则打印"Timeout occurred!"。
相关推荐
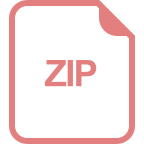












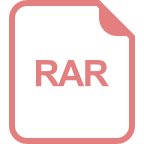
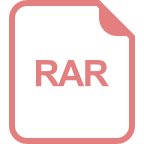