编写一个程序,要求用户输入一段英文文本,统计文本中每个单词出现的次数,并输出统计
时间: 2024-09-23 15:08:50 浏览: 81
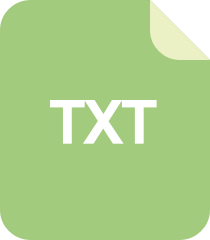
main.txt
编写一个简单的Python程序来实现这个功能:
```python
def word_count(text):
# 将文本转换为小写并分割成单词列表
words = text.lower().split()
# 创建一个空字典存储单词及其计数
word_dict = {}
# 遍历单词列表,更新字典中的计数
for word in words:
if word in word_dict:
word_dict[word] += 1
else:
word_dict[word] = 1
return word_dict
# 请求用户输入文本
user_text = input("请输入一段英文文本: ")
# 统计并打印结果
word_counts = word_count(user_text)
for word, count in word_counts.items():
print(f"{word}: {count} 次")
阅读全文
相关推荐
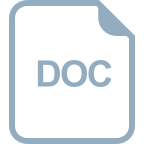
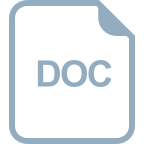






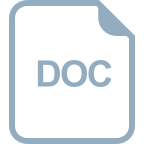








