pytorch BiLSTM
时间: 2023-10-29 10:00:09 浏览: 112
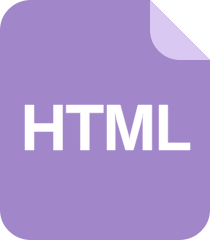
基于Pytorch实现LSTM
PyTorch中的BiLSTM是一种双向长短期记忆(Bi-directional Long Short-Term Memory)模型,可以用于序列数据的建模和处理。BiLSTM结合了正向和反向两个LSTM网络,其中正向LSTM按照时间顺序处理输入序列,而反向LSTM按照时间逆序处理输入序列。这种结构允许模型同时利用过去和未来的上下文信息。
在PyTorch中,可以使用`torch.nn.LSTM`和`torch.nn.LSTMCell`来实现BiLSTM模型。`torch.nn.LSTM`是一个多层LSTM模型的封装,而`torch.nn.LSTMCell`是一个单层LSTM模型的封装。
以下是一个使用BiLSTM模型进行文本分类的示例代码:
```python
import torch
import torch.nn as nn
class BiLSTM(nn.Module):
def __init__(self, input_dim, hidden_dim, output_dim):
super(BiLSTM, self).__init__()
self.hidden_dim = hidden_dim
self.lstm = nn.LSTM(input_dim, hidden_dim, bidirectional=True)
self.fc = nn.Linear(hidden_dim*2, output_dim)
def forward(self, input):
output, _ = self.lstm(input)
output = self.fc(output[:, -1, :])
return output
# 定义模型的输入维度、隐藏层维度和输出维度
input_dim = 100
hidden_dim = 50
output_dim = 10
# 创建BiLSTM模型实例
model = BiLSTM(input_dim, hidden_dim, output_dim)
# 构造输入数据
input = torch.randn(5, 3, input_dim) # 输入维度为(序列长度, 批次大小, 输入维度)
# 运行模型
output = model(input)
print(output.size()) # 输出维度为(序列长度, 批次大小, 输出维度)
```
这段代码定义了一个BiLSTM模型类`BiLSTM`,模型的输入维度为`input_dim`,隐藏层维度为`hidden_dim`,输出维度为`output_dim`。在`forward`方法中,首先使用`nn.LSTM`进行双向LSTM计算,然后通过线性层`nn.Linear`将输出转换为最终的预测结果。最后,可以通过输入数据调用模型来进行预测。
阅读全文
相关推荐




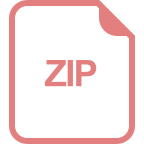
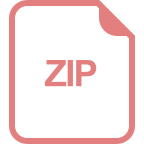
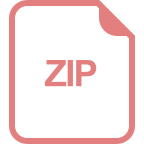
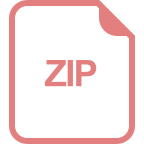
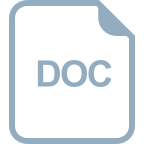






