The size of tensor a (16) must match the size of tensor b (15) at non-singleton dimension 3
时间: 2023-12-13 09:31:06 浏览: 33
这个错误提示表明在进行张量运算时,两个张量的维度不匹配。具体来说,张量a在第3个维度上的大小为16,而张量b在第3个维度上的大小为15,因此无法进行运算。解决这个问题的方法是调整张量的大小,使得它们在所有维度上的大小都匹配。
解决这个问题的方法有很多种,具体取决于你的应用场景和数据结构。以下是一些可能有用的方法:
1. 检查张量的维度和大小,确保它们符合你的预期。
2. 使用torch.reshape()或torch.view()函数调整张量的大小,使得它们在所有维度上的大小都匹配。
3. 使用torch.unsqueeze()函数在张量中添加一个新的维度,使得张量的大小匹配。
4. 检查你的代码,确保你没有在张量运算中使用了错误的维度。
相关问题
The size of tensor a (10) must match the size of tensor b (3) at non-singleton dimension 1
The error message "The size of tensor a (10) must match the size of tensor b (3) at non-singleton dimension 1" indicates that the dimensions of tensor a and tensor b do not match at dimension 1, which prevents the operation from being completed. It seems that the number of elements in tensor a at dimension 1 is 10, while the number of elements in tensor b at dimension 1 is 3.
To fix this issue, you can either resize one of the tensors to match the other tensor's dimension at dimension 1, or reshape one of the tensors to have a different number of dimensions.
Here are some possible solutions:
1. Reshape tensor a: You can reshape tensor a to match the number of elements in tensor b at dimension 1. For example, if tensor a has a shape of (10, 5) and tensor b has a shape of (3, 5), you can reshape tensor a to have a shape of (3, 2, 5) using the reshape() function.
2. Resize tensor b: Alternatively, you can resize tensor b to match the number of elements in tensor a at dimension 1. For example, if tensor a has a shape of (10, 5) and tensor b has a shape of (3, 5), you can resize tensor b to have a shape of (10, 5) using the resize() function.
3. Verify the input data: Double-check the input data for tensor a and tensor b to ensure that they have the correct dimensions and sizes.
The size of tensor a (3) must match the size of tensor b (0) at non-singleton dimension 3
根据提供的引用内容,您遇到的错误是"RuntimeError: The size of tensor a (3) must match the size of tensor b (0) at non-singleton dimension 3"。这个错误通常是由于张量的维度不匹配导致的。为了解决这个问题,您可以尝试以下方法:
1. 检查张量的维度:确保张量a和张量b在非单例维度3上的大小相同。您可以使用`size()`函数来检查张量的维度和大小。
2. 调整张量的大小:如果张量a和张量b的大小不匹配,您可以使用PyTorch的`view()`函数来调整张量的大小,以确保它们在非单例维度3上的大小相同。
3. 检查数据加载:如果您正在使用数据加载器加载数据,并且遇到了这个错误,那么可能是因为数据加载器返回的张量大小不一致。请确保您的数据加载器正确地处理数据,并返回具有相同大小的张量。
4. 检查模型结构:如果您正在使用模型进行训练,并且遇到了这个错误,那么可能是因为模型的输入和输出张量大小不匹配。请检查模型的结构,并确保输入和输出张量的大小匹配。
5. 检查损失函数:如果您正在使用损失函数计算损失,并且遇到了这个错误,那么可能是因为损失函数期望的输入张量大小与实际的张量大小不匹配。请检查损失函数的文档,并确保输入张量的大小与其期望的大小匹配。
请根据您的具体情况尝试上述方法来解决问题。如果问题仍然存在,请提供更多的上下文信息,以便我能够更好地帮助您解决问题。
相关推荐
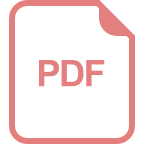
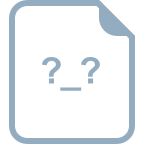
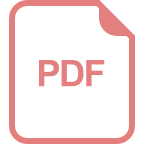












