def net(x): x = x.reshape((-1, num_inputs)) H = relu(x@w1 + b1) # 这⾥“@”代表矩阵乘法 return (H@w2 + b2)
时间: 2023-06-14 14:06:41 浏览: 264
这是一个神经网络的前向传播函数,其中包含两个隐藏层和一个输出层。具体来说,输入`x`通过一个线性变换 `x@w1 + b1`,然后通过 ReLU 非线性激活函数,得到隐藏层的输出`H`。隐藏层的输出再经过一个线性变换 `H@w2 + b2`,得到最终的输出。其中,`w1`、`w2`、`b1`、`b2`分别是网络的参数,`num_inputs`是输入的特征数。这个前向传播函数可以用来对输入数据进行预测。
相关问题
def net(X): X = X.reshape((-1, num_inputs)) H = relu(X@W1 + b1) # 这里“@”代表矩阵乘法 return (H@W2 + b2)
这是一个定义了一个简单的神经网络的函数 net(X)。该函数接受一个输入张量 X,并按照一定的计算规则进行前向传播。
首先,通过调用 X.reshape((-1, num_inputs)),将输入张量 X 重新形状为一个二维矩阵,其中维度为 -1 的部分会根据其他维度的大小自动计算得到。
接下来,通过 X@W1 + b1 进行线性变换,其中 @ 表示矩阵乘法,W1 是一个权重矩阵,b1 是一个偏置向量。然后将线性变换的结果通过 relu() 函数进行激活函数操作,得到激活后的输出 H。
最后,将激活后的输出 H 通过 H@W2 + b2 进行另一次线性变换和激活操作,得到最终的输出结果。
整个函数 net(X) 定义了一个两层的全连接神经网络,并且没有经过任何训练,可以用于进行预测。
def call(self, x): batch_size, _, _ = x.shape x = self.embeddings(x) # similar to embedding, expand feature dimensions to embedding_size m x = tf.transpose(x, perm=[0, 2, 1]) h_matrix = tf.zeros([batch_size, self.embedding_size, self.obs_len]) for i in tf.range(self.embedding_size): m = tf.reshape(x[:, i, :], shape=[batch_size, 1, -1]) h_m = self.lstm(m)[:, -1, :] for j in tf.range(batch_size): # update h_matrix h_matrix = tf.tensor_scatter_nd_update(h_matrix, [[j, i]], tf.reshape(h_m[j], shape=[1, -1])) h_matrix = LeakyReLU()(h_matrix) ht = tf.reshape(h_matrix[:, :, -1], shape=[batch_size, self.embedding_size, 1]) h_matrix = h_matrix[:, :, :-1] # reshape hidden states h_matrix to a shape like an image (n, h, w, c) h_matrix = tf.reshape(h_matrix, shape=[-1, self.embedding_size, self.obs_len - 1, 1]) vt = self.tpa(h_matrix, ht) ht_concat = tf.concat([vt, ht], axis=1) prediction = self.linear_final(tf.transpose(ht_concat, perm=[0, 2, 1])) return prediction
这段代码是在之前的基础上进行了一些额外的操作。
首先,通过LeakyReLU激活函数对h_matrix进行了激活操作,该函数可以增强模型的非线性特性。
然后,通过对h_matrix进行形状重塑操作,将其转换为类似图像的形式,即(n, h, w, c),其中n表示批次大小,h表示嵌入维度,w表示观测长度减1,c表示通道数(此处为1)。
接下来,通过调用self.tpa函数对h_matrix和ht进行处理,得到一个新的张量vt。
然后,通过在嵌入维度上将vt和ht进行拼接,得到ht_concat。
最后,通过对ht_concat进行转置操作,并将其输入到linear_final层中,得到最终的预测结果prediction。
整个过程可以看作是对隐藏状态序列h_matrix的进一步处理和转换,以生成最终的预测结果。
阅读全文
相关推荐














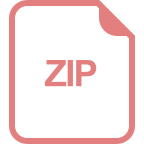