写一个bootstrap中的分页示例, 要求使用双向绑定数据
时间: 2024-04-30 09:18:57 浏览: 10
以下是一个使用Bootstrap中的分页示例,配合Vue.js实现双向绑定数据:
HTML代码:
```
<div id="app">
<table class="table table-striped table-bordered">
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Age</th>
</tr>
</thead>
<tbody>
<tr v-for="item in pagedItems">
<td>{{ item.id }}</td>
<td>{{ item.name }}</td>
<td>{{ item.age }}</td>
</tr>
</tbody>
</table>
<nav aria-label="Page navigation example">
<ul class="pagination">
<li class="page-item" :class="{ 'disabled': currentPage <= 1 }">
<a class="page-link" href="#" aria-label="Previous" @click.prevent="changePage(currentPage - 1)">
<span aria-hidden="true">«</span>
</a>
</li>
<li class="page-item" v-for="pageNumber in pages" :key="pageNumber" :class="{ 'active': currentPage === pageNumber }">
<a class="page-link" href="#" @click.prevent="changePage(pageNumber)">{{ pageNumber }}</a>
</li>
<li class="page-item" :class="{ 'disabled': currentPage >= totalPages }">
<a class="page-link" href="#" aria-label="Next" @click.prevent="changePage(currentPage + 1)">
<span aria-hidden="true">»</span>
</a>
</li>
</ul>
</nav>
</div>
```
JavaScript代码:
```
new Vue({
el: '#app',
data: {
items: [
{ id: 1, name: 'Alice', age: 20 },
{ id: 2, name: 'Bob', age: 25 },
{ id: 3, name: 'Charlie', age: 30 },
{ id: 4, name: 'David', age: 35 },
{ id: 5, name: 'Eva', age: 40 },
{ id: 6, name: 'Frank', age: 45 },
{ id: 7, name: 'Grace', age: 50 },
{ id: 8, name: 'Henry', age: 55 },
{ id: 9, name: 'Ivy', age: 60 },
{ id: 10, name: 'John', age: 65 }
],
pageSize: 3,
currentPage: 1
},
computed: {
totalPages() {
return Math.ceil(this.items.length / this.pageSize);
},
pages() {
const range = (start, end) => {
const length = end - start + 1;
return Array.from({ length }, (_, i) => start + i);
};
if (this.totalPages <= 9) {
return range(1, this.totalPages);
}
let startPage = Math.max(1, this.currentPage - 4);
let endPage = Math.min(this.totalPages, this.currentPage + 4);
if (startPage === 1) {
return range(1, 9);
}
if (endPage === this.totalPages) {
return range(this.totalPages - 8, this.totalPages);
}
return range(startPage, endPage);
},
pagedItems() {
const startIndex = (this.currentPage - 1) * this.pageSize;
return this.items.slice(startIndex, startIndex + this.pageSize);
}
},
methods: {
changePage(pageNumber) {
this.currentPage = pageNumber;
}
}
});
```
在这个示例中,我们使用了`v-for`指令来遍历数据,使用`computed`属性来计算页码和分页后的数据,使用`methods`方法来处理分页切换。同时,我们使用了Bootstrap中的分页组件来显示分页导航。通过Vue.js的双向绑定数据,我们可以很方便地实现分页功能。
相关推荐
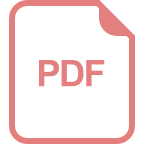














