二叉排序树的类型定义
时间: 2023-12-20 18:32:39 浏览: 80
二叉排序树的类型定义如下所示:
```c
// 定义二叉排序树节点值的类型为学生记录类型
typedef student ElemType;
// 定义二叉排序树的节点类型
typedef struct BSTNode {
ElemType data;
struct BSTNode *left;
struct BSTNode *rchild;
} BSTNode;
```
在上述代码中,`ElemType`是二叉排序树节点值的类型,可以根据实际需求进行定义。`BSTNode`是二叉排序树的节点类型,包含了节点的数据域`data`和左右子节点的指针域`left`和`rchild`。
相关问题
根据二叉排序树的抽象数据类型的定义,使用二叉链表实现一个二叉排序树。 二叉排序树的基本功能: 1、二叉排序树的建立 2、二叉排序树的查找 3、二叉排序树的插入 4、二叉排序树的删除 5、二叉排序树的销毁 6、其他:
好的,根据您的要求,我可以为您提供一个二叉排序树的基本实现。请注意,以下代码仅供参考,需要根据具体需求进行修改和完善。
```c++
#include <iostream>
using namespace std;
// 二叉排序树节点结构体
struct BSTNode {
int data; // 数据
BSTNode* left; // 左子树指针
BSTNode* right; // 右子树指针
};
// 二叉排序树类
class BinarySearchTree {
public:
BinarySearchTree() { root = NULL; } // 构造函数
~BinarySearchTree() { Destroy(root); } // 析构函数
// 基本功能
void Insert(int data); // 插入节点
bool Search(int data) const; // 查找节点
void Delete(int data); // 删除节点
void Destroy(BSTNode* node); // 销毁二叉排序树
// 其他功能
void InOrderTraversal() const; // 中序遍历
int Height() const; // 树的高度
private:
BSTNode* root; // 根节点指针
// 私有函数
void Insert(BSTNode*& node, int data); // 插入节点
bool Search(BSTNode* node, int data) const; // 查找节点
void Delete(BSTNode*& node, int data); // 删除节点
BSTNode* FindMin(BSTNode* node) const; // 查找最小节点
void InOrderTraversal(BSTNode* node) const; // 中序遍历
int Height(BSTNode* node) const; // 树的高度
};
// 插入节点
void BinarySearchTree::Insert(int data) {
Insert(root, data);
}
void BinarySearchTree::Insert(BSTNode*& node, int data) {
if (node == NULL) {
node = new BSTNode;
node->data = data;
node->left = node->right = NULL;
}
else if (data < node->data) {
Insert(node->left, data);
}
else if (data > node->data) {
Insert(node->right, data);
}
}
// 查找节点
bool BinarySearchTree::Search(int data) const {
return Search(root, data);
}
bool BinarySearchTree::Search(BSTNode* node, int data) const {
if (node == NULL) {
return false;
}
else if (data < node->data) {
return Search(node->left, data);
}
else if (data > node->data) {
return Search(node->right, data);
}
else {
return true;
}
}
// 删除节点
void BinarySearchTree::Delete(int data) {
Delete(root, data);
}
void BinarySearchTree::Delete(BSTNode*& node, int data) {
if (node == NULL) {
return;
}
else if (data < node->data) {
Delete(node->left, data);
}
else if (data > node->data) {
Delete(node->right, data);
}
else {
if (node->left == NULL && node->right == NULL) {
delete node;
node = NULL;
}
else if (node->left == NULL) {
BSTNode* temp = node;
node = node->right;
delete temp;
}
else if (node->right == NULL) {
BSTNode* temp = node;
node = node->left;
delete temp;
}
else {
BSTNode* temp = FindMin(node->right);
node->data = temp->data;
Delete(node->right, temp->data);
}
}
}
// 查找最小节点
BSTNode* BinarySearchTree::FindMin(BSTNode* node) const {
if (node == NULL) {
return NULL;
}
else if (node->left == NULL) {
return node;
}
else {
return FindMin(node->left);
}
}
// 销毁二叉排序树
void BinarySearchTree::Destroy(BSTNode* node) {
if (node != NULL) {
Destroy(node->left);
Destroy(node->right);
delete node;
}
}
// 中序遍历
void BinarySearchTree::InOrderTraversal() const {
InOrderTraversal(root);
}
void BinarySearchTree::InOrderTraversal(BSTNode* node) const {
if (node != NULL) {
InOrderTraversal(node->left);
cout << node->data << " ";
InOrderTraversal(node->right);
}
}
// 树的高度
int BinarySearchTree::Height() const {
return Height(root);
}
int BinarySearchTree::Height(BSTNode* node) const {
if (node == NULL) {
return 0;
}
else {
int leftHeight = Height(node->left);
int rightHeight = Height(node->right);
return (leftHeight > rightHeight) ? (leftHeight + 1) : (rightHeight + 1);
}
}
int main() {
BinarySearchTree bst;
bst.Insert(8);
bst.Insert(3);
bst.Insert(10);
bst.Insert(1);
bst.Insert(6);
bst.Insert(9);
bst.Insert(14);
bst.Insert(4);
bst.Insert(7);
bst.Insert(13);
cout << "中序遍历: ";
bst.InOrderTraversal();
cout << endl;
cout << "树的高度: " << bst.Height() << endl;
cout << "查找节点: " << endl;
cout << "3: " << bst.Search(3) << endl;
cout << "5: " << bst.Search(5) << endl;
cout << "删除节点: " << endl;
bst.Delete(1);
bst.Delete(3);
bst.Delete(13);
bst.Delete(8);
bst.Delete(10);
cout << "中序遍历: ";
bst.InOrderTraversal();
cout << endl;
cout << "树的高度: " << bst.Height() << endl;
return 0;
}
```
以上代码实现了二叉排序树的基本功能,包括插入节点、查找节点、删除节点、销毁二叉排序树等。另外,还实现了中序遍历和求树的高度等其他功能。在使用时,可以根据具体需求进行调用。
根据二叉排序树的抽象数据类型的定义,使用二叉链表实现一个二叉排序树。 二叉排序树的基本功能: •二叉排序树的建立 2、二叉排序树的查找 3、二叉排序树的插入 4、 二叉排序树的删除 5、 二叉排序树的销毁 6、其他:自定义操作 编写测试 main()函数测试二叉排序树的正确性。
<<
二叉排序树(Binary Search Tree, BST)是一种特殊的二叉树,其中每个节点的值大于其左子树中所有节点的值,并且小于其右子树中所有节点的值。这使得在二叉排序树中查找、插入和删除元素变得非常高效。
**根据二叉链表实现二叉排序树的步骤:**
1. **二叉排序树的建立** (Insertion):
- 创建一个空链表作为根节点。
- 当接收到新元素时,从根节点开始比较,如果新元素小于当前节点,向左子链表插入;如果新元素大于当前节点,向右子链表插入。递归这个过程直到找到合适的位置。
2. **二叉排序树的查找** (Traversal):
- 从根节点开始,对比目标值与节点值,如果相等则返回该节点,如果不等则根据大小关系继续在左或右子链表中查找。
3. **二叉排序树的插入** (Insertion):
- 删除操作分为三类:删除叶子节点、删除只有一个子节点的非叶子节点和删除有两个子节点的非叶子节点。需考虑替换、旋转等复杂情况。
5. **二叉排序树的销毁** (Deletion of the tree):
- 可以通过中序遍历二叉树,对每个节点执行析构操作,释放内存。
6. **自定义操作** (Custom Operations):
- 可以实现如最小值、最大值、前驱后继节点查找、范围查找等高级操作。
**测试main()函数**:
- 创建一个二叉排序树实例。
- 遍历并打印树中的所有元素,检查是否按顺序排列。
- 插入元素,检查是否成功插入。
- 查找特定元素,确认能否找到。
- 删除元素并再次验证结构的正确性。
- 对树执行自定义操作,验证结果。
测试代码示例:
```c++
// 假设BSTNode是一个包含值、左指针和右指针的链表节点结构体
BSTNode* createBST(BSTNode* root, int value) {
// 插入实现省略
}
BSTNode* searchBST(BSTNode* root, int value) {
// 查找实现省略
}
BSTNode* deleteBST(BSTNode* root, int value) {
// 删除实现省略
}
void destroyBST(BSTNode* root) {
if (root) {
destroyBST(root->left);
destroyBST(root->right);
delete root;
}
}
int main() {
BSTNode* root = nullptr; // 初始化为空
// 创建树、插入元素、查找元素、删除元素
// 执行自定义操作
// 检查main结束时,销毁BST
return 0;
}
阅读全文
相关推荐
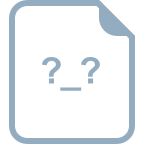
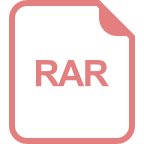





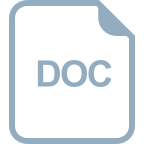






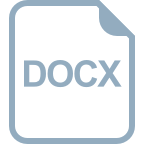