写一段贪吃蛇代码
时间: 2023-07-10 09:13:16 浏览: 53
好的,以下是一个简单的贪吃蛇游戏的Java代码示例:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.util.*;
public class SnakeGame extends JFrame implements ActionListener {
private Random rand = new Random();
private Timer timer;
private int delay = 100;
private int dotSize = 10;
private int boardWidth = 300;
private int boardHeight = 300;
private int dots = 3;
private int[] x = new int[500];
private int[] y = new int[500];
private int appleX;
private int appleY;
private boolean leftDirection = false;
private boolean rightDirection = true;
private boolean upDirection = false;
private boolean downDirection = false;
private boolean inGame = true;
public SnakeGame() {
setTitle("Snake Game");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(boardWidth, boardHeight);
setResizable(false);
setLocationRelativeTo(null);
initGame();
}
public void initGame() {
for (int i = 0; i < dots; i++) {
x[i] = 50 - i * dotSize;
y[i] = 50;
}
locateApple();
timer = new Timer(delay, this);
timer.start();
}
public void paint(Graphics g) {
super.paint(g);
if (inGame) {
g.setColor(Color.RED);
g.fillOval(appleX, appleY, dotSize, dotSize);
for (int i = 0; i < dots; i++) {
g.setColor(Color.GREEN);
g.fillOval(x[i], y[i], dotSize, dotSize);
}
Toolkit.getDefaultToolkit().sync();
} else {
gameOver(g);
}
}
public void gameOver(Graphics g) {
String message = "Game Over";
Font font = new Font("Helvetica", Font.BOLD, 14);
FontMetrics metrics = getFontMetrics(font);
g.setColor(Color.BLACK);
g.setFont(font);
g.drawString(message, (boardWidth - metrics.stringWidth(message)) / 2, boardHeight / 2);
}
public void checkApple() {
if ((x[0] == appleX) && (y[0] == appleY)) {
dots++;
locateApple();
}
}
public void move() {
for (int i = dots; i > 0; i--) {
x[i] = x[(i - 1)];
y[i] = y[(i - 1)];
}
if (leftDirection) {
x[0] -= dotSize;
}
if (rightDirection) {
x[0] += dotSize;
}
if (upDirection) {
y[0] -= dotSize;
}
if (downDirection) {
y[0] += dotSize;
}
}
public void checkCollision() {
for (int i = dots; i > 0; i--) {
if ((i > 4) && (x[0] == x[i]) && (y[0] == y[i])) {
inGame = false;
}
}
if (y[0] >= boardHeight) {
inGame = false;
}
if (y[0] < 0) {
inGame = false;
}
if (x[0] >= boardWidth) {
inGame = false;
}
if (x[0] < 0) {
inGame = false;
}
if (!inGame) {
timer.stop();
}
}
public void locateApple() {
int r = rand.nextInt((int) (boardWidth / dotSize)) * dotSize;
appleX = r;
r = rand.nextInt((int) (boardHeight / dotSize)) * dotSize;
appleY = r;
}
public void actionPerformed(ActionEvent e) {
if (inGame) {
checkApple();
checkCollision();
move();
}
repaint();
}
public static void main(String[] args) {
SnakeGame game = new SnakeGame();
game.setVisible(true);
}
}
```
这段代码是一个简单的贪吃蛇游戏,使用Java语言编写,采用了Java Swing框架实现图形界面。在代码中,使用了Timer类实现定时器,用于控制贪吃蛇的移动和游戏进程。同时,还实现了贪吃蛇的移动、碰撞检测、苹果的生成等功能。
相关推荐
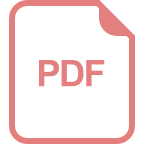
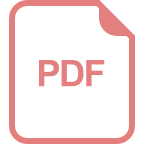
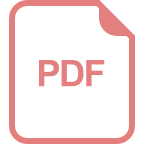












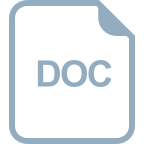
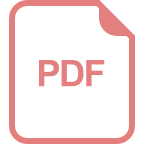
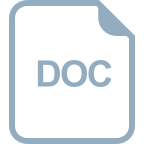