python提取多个log文件的指定内容并收集结果
时间: 2024-05-04 07:19:26 浏览: 197
可以使用Python标准库中的`re`模块来实现。以下是一个简单的示例代码,可以将多个log文件中所有包含指定关键字的行收集到一个结果文件中:
```python
import re
# 指定需要查找的关键字
pattern = re.compile('error|exception')
# 指定输入和输出文件路径
input_files = ['log1.txt', 'log2.txt', 'log3.txt']
output_file = 'result.txt'
with open(output_file, 'w') as f_out:
for file in input_files:
with open(file, 'r') as f_in:
for line in f_in:
if pattern.search(line):
f_out.write(line)
```
在这个示例代码中,我们首先使用`re.compile`方法创建了一个正则表达式对象`pattern`,用于匹配包含错误或异常信息的行。然后,我们指定了输入文件的路径列表`input_files`和输出文件的路径`output_file`。接下来,我们使用`with open`语句打开输入和输出文件,并逐行读取输入文件,将包含关键字的行写入输出文件中。
你可以根据你的具体需求修改代码中的正则表达式和文件路径等参数。
相关问题
python提取文件夹下面多个.log文件指定内容并收集结果到TXT上面
可以使用Python的内置模块os和re来实现这个任务。
首先,需要导入os和re模块:
```python
import os
import re
```
然后,可以使用os模块的listdir函数列出指定文件夹下的所有文件:
```python
folder_path = "/path/to/folder"
files = os.listdir(folder_path)
```
接着,可以使用re模块来匹配需要提取的内容。假设需要提取的内容是以"error"开头的行,可以使用以下正则表达式:
```python
pattern = "^error.*"
```
接下来,可以遍历所有的文件,逐一读取文件内容并提取需要的内容:
```python
result = []
for file_name in files:
if file_name.endswith(".log"):
file_path = os.path.join(folder_path, file_name)
with open(file_path, "r") as f:
for line in f:
if re.match(pattern, line):
result.append(line)
```
最后,可以将提取到的结果写入到一个TXT文件中:
```python
output_file = "/path/to/output.txt"
with open(output_file, "w") as f:
f.writelines(result)
```
完整代码如下:
```python
import os
import re
folder_path = "/path/to/folder"
output_file = "/path/to/output.txt"
pattern = "^error.*"
files = os.listdir(folder_path)
result = []
for file_name in files:
if file_name.endswith(".log"):
file_path = os.path.join(folder_path, file_name)
with open(file_path, "r") as f:
for line in f:
if re.match(pattern, line):
result.append(line)
with open(output_file, "w") as f:
f.writelines(result)
```
注意,如果需要提取其他的内容,可以修改正则表达式的pattern变量。
python提取多个文件夹下面多个.log文件里面指定内容并收集结果到TXT上面
可以使用Python内置的os和re模块来实现。具体步骤如下:
1. 遍历多个文件夹,找到所有的.log文件;
2. 逐个打开.log文件,使用正则表达式匹配指定内容,并将结果保存到列表中;
3. 将列表中的所有结果写入到一个TXT文件中。
下面是一个示例代码,仅供参考:
```python
import os
import re
# 指定需要匹配的内容
pattern = re.compile(r'Test: (\d+)')
# 遍历多个文件夹,找到所有的.log文件
folders = ['folder1', 'folder2']
log_files = []
for folder in folders:
for file in os.listdir(folder):
if file.endswith('.log'):
log_files.append(os.path.join(folder, file))
# 逐个打开.log文件,匹配指定内容,并将结果保存到列表中
results = []
for file in log_files:
with open(file, 'r') as f:
for line in f:
match = pattern.search(line)
if match:
results.append(match.group(1))
# 将列表中的所有结果写入到一个TXT文件中
with open('results.txt', 'w') as f:
f.write('\n'.join(results))
```
其中,`folders`为需要遍历的文件夹列表,`pattern`为需要匹配的内容,本示例中为匹配形如`Test: 123`的内容。最终结果将保存在`results.txt`文件中。
阅读全文
相关推荐






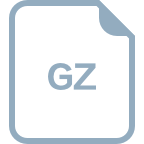


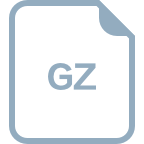






