AttributeError: module 'tensorflow._api.v2.image' has no attribute
时间: 2023-12-06 19:37:37 浏览: 255
这个错误通常是因为在导入tensorflow时,使用了错误的模块或属性名称。在这种情况下,错误的模块或属性名称是“image”。这可能是因为您使用的是较旧版本的tensorflow,或者您的代码中存在拼写错误。
要解决此问题,您可以尝试以下几个步骤:
1.检查您的代码中是否存在拼写错误,并确保正确导入了tensorflow模块。
2.如果您使用的是较旧版本的tensorflow,请尝试升级到最新版本。
3.如果您使用的是较新版本的tensorflow,请检查您的代码是否使用了已弃用的模块或属性名称。在这种情况下,您可以尝试使用新的模块或属性名称来解决问题。
以下是一个例子,演示如何使用tensorflow的Image模块来加载和处理图像:
```python
import tensorflow as tf
from tensorflow.keras.preprocessing.image import load_img
# 加载图像
img = load_img('image.jpg')
# 显示图像
img.show()
# 将图像转换为numpy数组
img_array = tf.keras.preprocessing.image.img_to_array(img)
# 显示图像数组的形状
print(img_array.shape)
```
相关问题
AttributeError: module 'tensorflow_core._api.v2.train' has no attribute 'Optimizer'
AttributeError: module 'tensorflow_core._api.v2.train' has no attribute 'Optimizer' 是一个错误提示,意味着在tensorflow_core._api.v2.train模块中没有名为'Optimizer'的属性。
这个错误通常发生在使用旧版本的TensorFlow代码时,因为在较新的TensorFlow版本中,Optimizer类已经被移动到了tensorflow.keras.optimizers模块中。
要解决这个问题,你可以尝试以下几种方法:
1. 检查你的TensorFlow版本是否较旧。如果是,请升级到最新版本,以确保你使用的是最新的API。
2. 如果你无法升级TensorFlow版本,可以尝试将代码中的'tensorflow_core._api.v2.train.Optimizer'替换为'tensorflow.keras.optimizers.Optimizer'。
3. 如果你的代码中没有直接使用Optimizer类,而是通过其他方式引用了tensorflow_core._api.v2.train模块,那么你可能需要检查你的代码逻辑,并确保正确导入了所需的模块。
希望以上信息对你有帮助!如果还有其他问题,请继续提问。
AttributeError: module 'tensorflow._api.v2.lookup' has no attribute 'staticHashTable'
AttributeError: module 'tensorflow._api.v2.lookup' has no attribute 'staticHashTable' 是一个Python错误,当你尝试在TensorFlow 2.x版本中访问`staticHashTable`这个属性,但该模块实际上并没有提供这个属性时会出现这种错误。`tensorflow._api.v2.lookup` 是TensorFlow中的一个子模块,可能在你引用的版本中已经被重构或移除。
通常,这表明你的代码试图使用的API已经过时,或者你可能需要更新你的TensorFlow库到最新版本,或者检查文档以确认正确的API调用方式。如果你确实需要`staticHashTable`这个功能,可能你需要查阅TensorFlow的最新文档,看看是否有替代的函数或模块来完成相同任务。
阅读全文
相关推荐
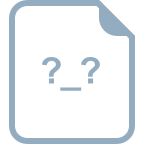
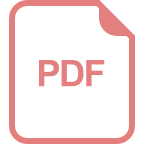
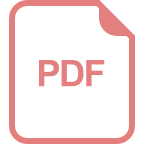













