c++,实现对一个长方体进行体积、表面积的计算
时间: 2024-09-27 22:09:24 浏览: 58
在C++中,计算长方体的体积和表面积通常涉及到结构体或者类来存储长方体的相关尺寸,如长度(length)、宽度(width)和高度(height)。下面是一个简单的例子,首先定义一个`Cube`类,包含体积和表面积的成员函数:
```cpp
#include <iostream>
// 定义一个立方体类
class Cube {
private:
double length; // 长度
double width; // 宽度
double height; // 高度
public:
// 构造函数
Cube(double l, double w, double h) : length(l), width(w), height(h) {}
// 计算体积
double volume() const {
return length * width * height;
}
// 计算表面积
double surfaceArea() const {
return 2 * (length * width + length * height + width * height);
}
// 显示信息
void displayProperties() const {
std::cout << "Volume: " << volume() << "\n";
std::cout << "Surface Area: " << surfaceArea() << "\n";
}
};
int main() {
// 创建一个立方体实例并设置尺寸
Cube myCube(5.0, 4.0, 3.0);
// 计算并显示属性
myCube.displayProperties();
return 0;
}
```
在这个例子中,用户可以创建`Cube`对象,并通过调用`volume()`和`surfaceArea()`方法获取体积和表面积。如果你有其他需求,比如输入用户的尺寸或者处理更复杂的形状,只需稍作修改即可。
阅读全文
相关推荐
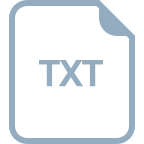
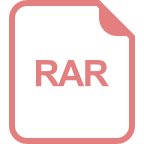





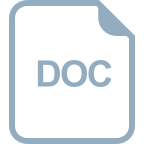
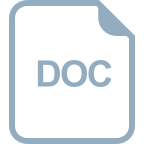








