使用队列实现BFS
时间: 2023-07-11 07:13:47 浏览: 92
好的,使用队列实现BFS(广度优先搜索)的基本步骤如下:
1. 将起始节点放入队列中。
2. 从队列中取出一个节点,将该节点标记为已访问。
3. 遍历该节点的所有邻居节点,将未访问的邻居节点放入队列中。
4. 重复步骤 2 和步骤 3 直到队列为空。
下面是一个 Python 代码示例:
```python
from queue import Queue
def bfs(start_node):
visited = set()
q = Queue()
q.put(start_node)
while not q.empty():
node = q.get()
visited.add(node)
for neighbor in node.neighbors:
if neighbor not in visited:
q.put(neighbor)
return visited
```
其中,start_node 是起始节点,visited 是已访问过的节点的集合,q 是队列。在每次从队列中取出一个节点时,都将该节点加入 visited 集合中,以确保不会重复访问。遍历每个节点的邻居节点时,只将未访问过的邻居节点放入队列中。最终返回 visited 集合即可。
相关问题
基于队列实现bfs算法c语言
以下是基于队列实现BFS算法的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_QUEUE_SIZE 1000
typedef struct {
int x;
int y;
} Point;
typedef struct {
Point data[MAX_QUEUE_SIZE];
int front;
int rear;
} Queue;
void initQueue(Queue *q) {
q->front = -1;
q->rear = -1;
}
int isEmpty(Queue *q) {
return (q->front == -1 && q->rear == -1);
}
int isFull(Queue *q) {
return (q->rear + 1) % MAX_QUEUE_SIZE == q->front;
}
void enqueue(Queue *q, Point p) {
if (isFull(q)) {
printf("Error: Queue is full!\n");
exit(EXIT_FAILURE);
}
if (isEmpty(q)) {
q->front = q->rear = 0;
} else {
q->rear = (q->rear + 1) % MAX_QUEUE_SIZE;
}
q->data[q->rear] = p;
}
Point dequeue(Queue *q) {
if (isEmpty(q)) {
printf("Error: Queue is empty!\n");
exit(EXIT_FAILURE);
}
Point p = q->data[q->front];
if (q->front == q->rear) {
q->front = q->rear = -1;
} else {
q->front = (q->front + 1) % MAX_QUEUE_SIZE;
}
return p;
}
void bfs(int **graph, int n, int start) {
Queue q;
initQueue(&q);
int visited[n];
for (int i = 0; i < n; i++) {
visited[i] = 0;
}
Point p = {start, 0};
visited[start] = 1;
enqueue(&q, p);
while (!isEmpty(&q)) {
Point curr = dequeue(&q);
printf("%d ", curr.x);
for (int i = 0; i < n; i++) {
if (graph[curr.x][i] == 1 && visited[i] == 0) {
Point next = {i, curr.y + 1};
visited[i] = 1;
enqueue(&q, next);
}
}
}
}
int main() {
int n = 8;
int **graph = (int **)malloc(n * sizeof(int *));
for (int i = 0; i < n; i++) {
graph[i] = (int *)malloc(n * sizeof(int));
}
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
graph[i][j] = 0;
}
}
graph[0][1] = graph[1][0] = 1;
graph[0][2] = graph[2][0] = 1;
graph[1][3] = graph[3][1] = 1;
graph[1][4] = graph[4][1] = 1;
graph[2][5] = graph[5][2] = 1;
graph[2][6] = graph[6][2] = 1;
graph[4][7] = graph[7][4] = 1;
bfs(graph, n, 0);
return 0;
}
```
该代码是一个简单的BFS实现,图的邻接矩阵存储在一个二维数组中,函数 `bfs` 接受该二维数组、顶点数和起点编号作为参数,并输出遍历结果。在函数中使用了一个队列来辅助实现BFS。具体实现可参考代码注释。
优先队列 + bfs
优先队列与BFS(广度优先搜索)是两个不同的概念。优先队列是一种数据结构,类似于队列,但是它的元素按照一定的优先级进行排序,每次出队时,都会选择优先级最高的元素出队。而BFS是一种图遍历算法,它从起始节点开始,逐层向外扩展,直到找到目标节点或者遍历完所有可达节点。
在上面的引用中,优先队列和BFS通常结合使用,以实现修改后的BFS算法。通过使用优先队列存储待扩展的节点,并根据节点的某个属性(如时间)进行排序,可以确保每次扩展的都是时间最短的节点,从而提高算法的效率。
阅读全文
相关推荐
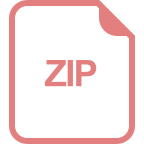
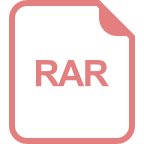
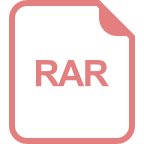












