生成JAVA实现中国象棋代码
时间: 2023-11-12 09:04:56 浏览: 50
以下是一个简单的JAVA实现中国象棋的代码示例:
```java
//定义棋子类
class ChessPiece {
String name; // 棋子名称
int x, y; // 棋子坐标
public ChessPiece(String name, int x, int y) {
this.name = name;
this.x = x;
this.y = y;
}
public String toString() {
return name + " (" + x + "," + y + ")";
}
}
//定义棋盘类
class ChessBoard {
ChessPiece[][] board;
public ChessBoard() {
board = new ChessPiece[10][9];
}
// 初始化棋盘
public void init() {
// 初始化黑方
board[0][0] = new ChessPiece("車", 0, 0);
board[0][1] = new ChessPiece("馬", 0, 1);
board[0][2] = new ChessPiece("象", 0, 2);
board[0][3] = new ChessPiece("士", 0, 3);
board[0][4] = new ChessPiece("將", 0, 4);
board[0][5] = new ChessPiece("士", 0, 5);
board[0][6] = new ChessPiece("象", 0, 6);
board[0][7] = new ChessPiece("馬", 0, 7);
board[0][8] = new ChessPiece("車", 0, 8);
board[2][1] = new ChessPiece("炮", 2, 1);
board[2][7] = new ChessPiece("炮", 2, 7);
board[3][0] = new ChessPiece("卒", 3, 0);
board[3][2] = new ChessPiece("卒", 3, 2);
board[3][4] = new ChessPiece("卒", 3, 4);
board[3][6] = new ChessPiece("卒", 3, 6);
board[3][8] = new ChessPiece("卒", 3, 8);
// 初始化红方
board[9][0] = new ChessPiece("車", 9, 0);
board[9][1] = new ChessPiece("馬", 9, 1);
board[9][2] = new ChessPiece("象", 9, 2);
board[9][3] = new ChessPiece("士", 9, 3);
board[9][4] = new ChessPiece("帥", 9, 4);
board[9][5] = new ChessPiece("士", 9, 5);
board[9][6] = new ChessPiece("象", 9, 6);
board[9][7] = new ChessPiece("馬", 9, 7);
board[9][8] = new ChessPiece("車", 9, 8);
board[7][1] = new ChessPiece("炮", 7, 1);
board[7][7] = new ChessPiece("炮", 7, 7);
board[6][0] = new ChessPiece("兵", 6, 0);
board[6][2] = new ChessPiece("兵", 6, 2);
board[6][4] = new ChessPiece("兵", 6, 4);
board[6][6] = new ChessPiece("兵", 6, 6);
board[6][8] = new ChessPiece("兵", 6, 8);
}
// 打印棋盘
public void print() {
for (int i = 0; i < board.length; i++) {
for (int j = 0; j < board[i].length; j++) {
if (board[i][j] != null) {
System.out.print(board[i][j] + "\t");
} else {
System.out.print("\t");
}
}
System.out.println("");
}
}
// 移动棋子
public void move(int startX, int startY, int endX, int endY) {
ChessPiece piece = board[startX][startY];
board[startX][startY] = null;
board[endX][endY] = piece;
piece.x = endX;
piece.y = endY;
}
}
//测试类
public class ChessTest {
public static void main(String[] args) {
ChessBoard board = new ChessBoard();
board.init();
board.print();
board.move(3, 0, 4, 0);
board.print();
}
}
```
以上代码是一个简单的实现,具体规则和完整实现可以根据需求进行扩展。
相关推荐
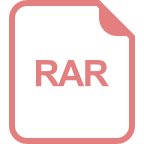
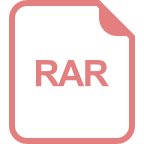
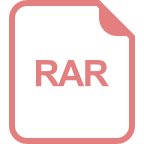














