data数据有三列,用户user_ID,物品item_ID,用户对物品的打分(1-5分),下面把数据拆分成训练集和测试集,在训练集上建立lightGCN模型,并在测试集上预测recall,ngcd;给出完整代码
时间: 2024-09-08 11:04:28 浏览: 99
在处理用户评分数据时,将数据拆分为训练集和测试集是一个常见的步骤,用于评估推荐模型的性能。lightGCN是一种基于图卷积网络的推荐系统模型,它能够在图结构的数据上学习用户和物品的低维嵌入表示,从而提高推荐质量。以下是使用Python进行该任务的简化示例代码,利用了lightGCN实现以及相关库,例如pandas用于数据处理和lightfm库中的LightGCN实现。请注意,这个例子假设数据已经被加载为pandas DataFrame,且数据格式是用户user_ID, 物品item_ID,用户对物品的打分(1-5分)。
```python
import pandas as pd
from lightfm import LightFM
from lightfm.data import Dataset
from sklearn.model_selection import train_test_split
from lightfm.evaluation import precision_at_k, recall_at_k
# 假设df是已经加载好的DataFrame,有三列: 'user_ID', 'item_ID', 'score'
df = pd.read_csv('data.csv') # 示例:加载数据
# 将数据转换为lightfm格式
dataset = Dataset()
dataset.fit(df['user_ID'], df['item_ID'])
(interactions, weights) = dataset.build_interactions(
((uid, iid, score) for uid, iid, score in zip(df['user_ID'], df['item_ID'], df['score']))
)
# 拆分数据为训练集和测试集,这里用80%的数据作为训练集,20%作为测试集
train_interactions, test_interactions = train_test_split(interactions, test_size=0.2)
# 初始化LightGCN模型
model = LightFM(no_components=30, loss='warp')
# 训练模型
model.fit(train_interactions, sample_weight=weights, epochs=30)
# 在测试集上预测
test_precision = precision_at_k(model, test_interactions, k=10).mean()
test_recall = recall_at_k(model, test_interactions, k=10).mean()
print('Precision: %f' % test_precision)
print('Recall: %f' % test_recall)
# 由于lightFM库没有直接的ngcd(Normalized Cumulative Gain at k)计算方法,通常我们使用标准库计算
# 下面是计算NGCD的示例代码,注意这个假设你已经有了预测得分和真实标签
def ngcd(y_true, y_pred, k=10):
# 获取前k个预测
top_k_predictions = y_pred.argsort()[:k]
y_true_top_k = y_true[top_k_predictions]
dcg = (y_true_top_k / np.log2(np.arange(2, k + 2))).sum()
idcg = (y_true[:k] / np.log2(np.arange(2, k + 2))).sum()
return dcg / idcg
y_pred = model.predict(test_interactions)
ngcd_value = ngcd(y_true=weights, y_pred=y_pred, k=10)
print('NCGD: %f' % ngcd_value)
```
注意:此代码示例是一个非常基础的版本,实际情况中你可能需要对数据进行更多的预处理,以及对模型进行调整,比如设置随机种子,调整模型参数等,以获得更好的效果。
阅读全文
相关推荐
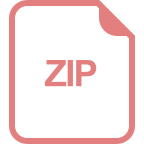
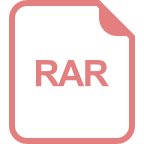







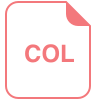








