请用C++设计一个算法Insert(int i, T e):在单链表中插入e作为第i个元素。
时间: 2024-10-24 07:14:31 浏览: 25
在C++中,我们可以创建一个插入功能的函数`Insert`,首先需要确保链表结构支持动态插入。下面是一个基本的单链表节点定义,然后给出插入函数的实现:
```cpp
#include <iostream>
// 定义单链表节点
template <typename T>
struct ListNode {
T val;
ListNode<T>* next;
ListNode(T x) : val(x), next(nullptr) {}
};
template <typename T>
void Insert(ListNode<T>** head, int i, const T& e) {
if (i <= 0) {
std::cout << "Invalid index! Index must be a positive number.\n";
return;
}
ListNode<T>* newNode = new ListNode<T>(e); // 创建新节点
if (*head == nullptr || i == 1) { // 如果链表为空或者要插入的位置是第一个节点
newNode->next = *head;
*head = newNode;
} else {
ListNode<T>* current = *head;
for (int j = 1; j < i - 1 && current != nullptr; ++j) {
current = current->next;
}
if (current == nullptr) {
std::cout << "Index out of range!\n";
delete newNode;
return;
}
newNode->next = current->next;
current->next = newNode;
}
}
```
这个函数首先检查输入的索引是否有效,如果无效则返回错误信息并终止。接着,根据索引位置的不同情况,分别处理空链表、只有一个节点以及插入中间节点的情况。
阅读全文
相关推荐
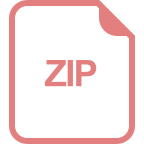
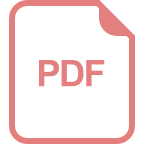
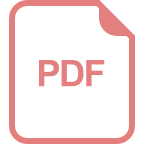









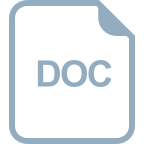
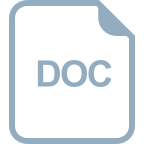




