用数组实现队列,java
时间: 2024-10-19 15:12:22 浏览: 36
在Java中,可以使用数组作为基础数据结构来实现一个简单的队列。数组队列通常有两种操作:入队(enqueue)和出队(dequeue)。由于数组索引是从0开始的,我们可以使用数组的前半部分存储队列元素,后半部分作为临时空间。
**队列类的简单实现:**
```java
public class ArrayQueue {
private int[] queue;
private int front; // 队首指针
private int rear; // 队尾指针
private int capacity;
public ArrayQueue(int size) {
capacity = size;
queue = new int[capacity];
front = -1;
rear = -1;
}
// 入队
public void enqueue(int item) {
if ((rear + 1) % capacity == front) { // 当队满时
throw new IllegalStateException("Queue is full");
}
if (front == -1) { // 初始化队列
front = 0;
} else {
rear = (rear + 1) % capacity;
}
queue[rear] = item;
}
// 出队
public int dequeue() {
if (front == -1 || front == rear) { // 队空或只有一个元素
throw new IllegalStateException("Queue is empty");
}
int removedItem = queue[front];
if (front == rear) { // 队列只剩一个元素
front = rear = -1;
} else {
front = (front + 1) % capacity;
}
return removedItem;
}
// 查看队列是否为空
public boolean isEmpty() {
return front == -1;
}
// 查看队列是否已满
public boolean isFull() {
return (rear + 1) % capacity == front;
}
}
```
**
阅读全文
相关推荐
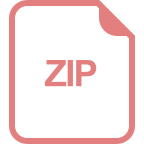
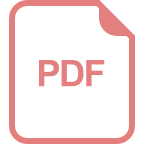
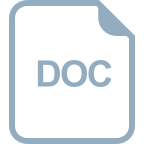
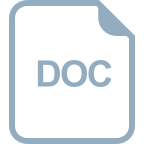




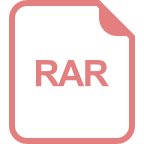
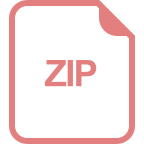
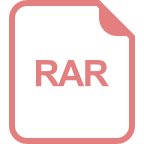
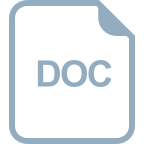




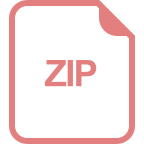