用数组实现队列java
时间: 2024-10-25 22:11:38 浏览: 20
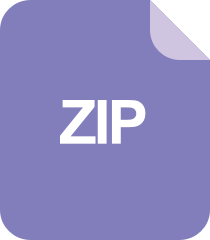
用数组实现的优先队列(JAVA)
在Java中,可以使用数组来实现一个基本的队列数据结构,通常采用数组的后进先出(LIFO,Last In First Out)特性来模拟队列的操作。这里是一个简单的基于数组实现的队列:
```java
public class ArrayQueue {
private int capacity;
private int front; // 队头指针
private int rear; // 队尾指针
// 初始化队列,默认容量为5
public ArrayQueue() {
this.capacity = 5;
this.front = -1;
this.rear = -1;
}
// 如果队列已满,抛出异常
public boolean isFull() {
return rear == (front + capacity - 1) % capacity;
}
// 如果队列为空,抛出异常
public boolean isEmpty() {
return front == rear;
}
// 入队操作
public void enqueue(int item) {
if (isFull()) {
throw new RuntimeException("Queue is full");
}
rear = (rear + 1) % capacity; // 向后移动队尾指针
data[rear] = item; // 插入元素
if (isEmpty()) front = rear; // 队头等于队尾,更新队头
}
// 出队操作
public int dequeue() {
if (isEmpty()) {
throw new RuntimeException("Queue is empty");
}
int removedItem = data[front]; // 获取并移除队头元素
front = (front + 1) % capacity; // 后移队头指针
if (front == rear) rear = -1; // 当只剩下一个元素时,重置队尾
return removedItem;
}
// 返回队列当前大小
public int size() {
return (rear >= front) ? rear - front : capacity - front + rear;
}
// 队列内部存储的数据,为了示例,假设长度为10
private int[] data = new int[capacity];
}
```
阅读全文
相关推荐
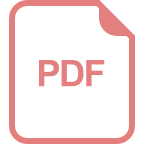

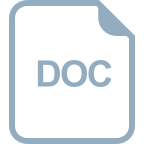
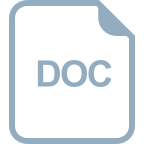




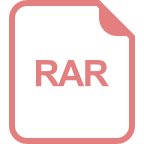
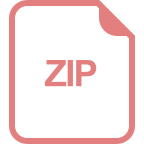
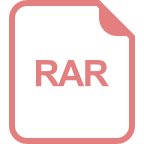
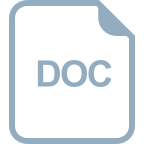



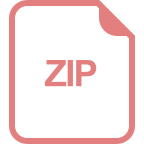