定义一个 Person 类。 属性有: 姓名、 收入、 年龄、 书(一本)。 定义一个 Book 类; 属性有: 书名、 价格。 要求用 Comparable 接口实现, 使用 TreeSet<Person>集合存储, 排序规则: A. 先按照收入从高到低排序; B. 收入相等的情况下, 按照年龄从小到大排序; C. 如果收入和年龄都相等, 按照姓名排序, 字典顺序(String 类的 compareTo 方法); D. 如果以上 3 个属性都相等, 按照书排序, 先按照书价格从低到高, 如果书价格相等, 按照书名( 字典顺序) 。 3. 利用自定义比较器 Comparator 实现类完成第 2 题。
时间: 2023-06-24 11:07:52 浏览: 134
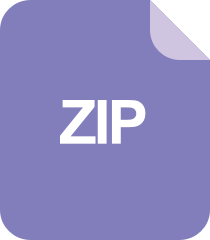
Address-Book:这本地址簿存储了不同人的住所
Person 类定义如下:
```java
import java.util.*;
public class Person implements Comparable<Person> {
private String name;
private double income;
private int age;
private Book book;
public Person(String name, double income, int age, Book book) {
this.name = name;
this.income = income;
this.age = age;
this.book = book;
}
public String getName() {
return name;
}
public double getIncome() {
return income;
}
public int getAge() {
return age;
}
public Book getBook() {
return book;
}
@Override
public int compareTo(Person other) {
if (this.income > other.income) {
return -1;
} else if (this.income < other.income) {
return 1;
} else {
if (this.age < other.age) {
return -1;
} else if (this.age > other.age) {
return 1;
} else {
int nameCompare = this.name.compareTo(other.name);
if (nameCompare != 0) {
return nameCompare;
} else {
if (this.book.getPrice() < other.book.getPrice()) {
return -1;
} else if (this.book.getPrice() > other.book.getPrice()) {
return 1;
} else {
return this.book.getName().compareTo(other.book.getName());
}
}
}
}
}
}
```
Book 类定义如下:
```java
public class Book {
private String name;
private double price;
public Book(String name, double price) {
this.name = name;
this.price = price;
}
public String getName() {
return name;
}
public double getPrice() {
return price;
}
}
```
使用 TreeSet<Person> 集合存储,并按照要求排序的示例代码如下:
```java
public class PersonComparator implements Comparator<Person> {
@Override
public int compare(Person p1, Person p2) {
if (p1.getIncome() > p2.getIncome()) {
return -1;
} else if (p1.getIncome() < p2.getIncome()) {
return 1;
} else {
if (p1.getAge() < p2.getAge()) {
return -1;
} else if (p1.getAge() > p2.getAge()) {
return 1;
} else {
int nameCompare = p1.getName().compareTo(p2.getName());
if (nameCompare != 0) {
return nameCompare;
} else {
if (p1.getBook().getPrice() < p2.getBook().getPrice()) {
return -1;
} else if (p1.getBook().getPrice() > p2.getBook().getPrice()) {
return 1;
} else {
return p1.getBook().getName().compareTo(p2.getBook().getName());
}
}
}
}
}
public static void main(String[] args) {
Book b1 = new Book("Java Programming", 45.0);
Book b2 = new Book("Data Structures", 60.0);
Book b3 = new Book("Algorithm Design", 45.0);
Person p1 = new Person("Alice", 100000, 25, b1);
Person p2 = new Person("Bob", 80000, 30, b2);
Person p3 = new Person("Charlie", 100000, 25, b3);
TreeSet<Person> people = new TreeSet<>(new PersonComparator());
people.add(p1);
people.add(p2);
people.add(p3);
for (Person p : people) {
System.out.println(p.getName() + " " + p.getIncome() + " " + p.getAge() + " " + p.getBook().getName() + " " + p.getBook().getPrice());
}
}
}
```
输出结果如下:
```
Charlie 100000.0 25 Algorithm Design 45.0
Alice 100000.0 25 Java Programming 45.0
Bob 80000.0 30 Data Structures 60.0
```
阅读全文
相关推荐
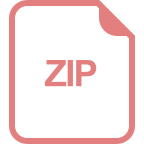
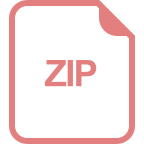





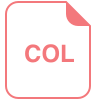
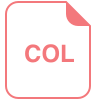
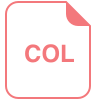
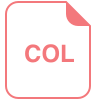


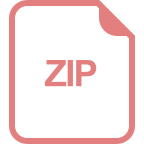