torch如何使用softmax
时间: 2024-06-05 10:03:02 浏览: 15
在PyTorch中,可以使用torch.nn.functional模块中的softmax函数来实现softmax操作。softmax函数将一个向量映射为一个概率分布,使得向量中的每个元素都在0到1之间,并且所有元素的和为1。
下面是使用softmax函数的示例代码:
```python
import torch
import torch.nn.functional as F
# 定义一个输入向量
input_vector = torch.tensor([1.0, 2.0, 3.0])
# 使用softmax函数进行操作
output_vector = F.softmax(input_vector, dim=0)
print(output_vector)
```
在上述代码中,我们首先导入了torch和torch.nn.functional模块。然后,我们定义了一个输入向量input_vector,其中包含了三个元素。接下来,我们使用F.softmax函数对input_vector进行softmax操作,并将结果保存在output_vector中。最后,我们打印输出output_vector。
请注意,softmax函数的第二个参数dim指定了在哪个维度上进行softmax操作。在上述示例中,我们将dim设置为0,表示对输入向量的第一个维度进行softmax操作。
相关问题
torch.softmax
torch.softmax 是 PyTorch 中的一个函数,用于计算 softmax 函数。softmax 函数是一种常见的激活函数,通常用于多分类问题中,将输出的原始分数转换为概率分布。
其函数定义为:`torch.softmax(input, dim=None, _stacklevel=3, dtype=None)`
其中,`input` 是输入的张量;`dim` 是沿着哪个维度进行计算 softmax 函数,默认为最后一个维度;`_stacklevel` 和 `dtype` 是可选参数。
举个例子,假设有一个大小为 (3, 4) 的张量 `x`,我们要对最后一个维度进行 softmax 操作,可以使用以下代码:
```
import torch
x = torch.randn(3, 4)
y = torch.softmax(x, dim=-1)
print(y)
```
输出结果为:
```
tensor([[0.3021, 0.2271, 0.0792, 0.3916],
[0.1253, 0.0705, 0.1157, 0.6885],
[0.1546, 0.2040, 0.1178, 0.5236]])
```
可以看到,输出的张量 `y` 沿着最后一个维度进行了 softmax 操作,每一行的和都等于 1。
torch中softmax
在PyTorch中,softmax函数是一个常用的操作,它将一个向量转换为概率分布。PyTorch提供了torch.nn.functional.softmax函数来实现softmax操作。
你可以使用以下代码示例来计算一个向量的softmax:
```python
import torch
import torch.nn.functional as F
# 输入向量
x = torch.tensor([1.0, 2.0, 3.0])
# 计算softmax
output = F.softmax(x, dim=0)
print(output)
```
在上述代码中,我们首先导入了torch和torch.nn.functional模块。然后,我们定义了一个输入向量x,
相关推荐
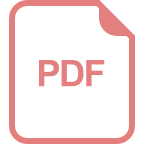
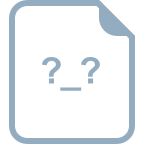
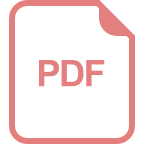












