定义一个按钮类(CMyButton),包含的私有数据成员有:宽度(m_width)、高度 (m_height)、显示文字(m_caption)、背景颜色(m_color)和状态(m_status:有效/无效),若干公有成员函数完成对各数据成员的操作。定义类的多个构造函数(输出构造 信息),析构函数(输出析构信息),拷贝构造函数。注意:状态为有效时候,才可以修改按钮的数据成员.测试使用类构造对象btn1,并使用 btn1 去构造 btn2。注意区分私有成 员和公有成员,注意观察对象构造和析构的过程。要求程序尽可能的简短一点,简短,简短!!
时间: 2024-02-24 21:56:03 浏览: 80
好的,下面是实现类CMyButton的代码,包括构造函数、析构函数、拷贝构造函数和成员函数:
```c++
#include <iostream>
#include <string>
using namespace std;
class CMyButton {
private:
int m_width;
int m_height;
string m_caption;
string m_color;
bool m_status;
public:
// 默认构造函数
CMyButton() : m_width(100), m_height(50), m_caption("Button"), m_color("white"), m_status(true) {
cout << "Default constructor called" << endl;
}
// 带参数的构造函数
CMyButton(int width, int height, string caption, string color, bool status) : m_width(width), m_height(height), m_caption(caption), m_color(color), m_status(status) {
cout << "Constructor called with parameters" << endl;
}
// 拷贝构造函数
CMyButton(const CMyButton& other) {
m_width = other.m_width;
m_height = other.m_height;
m_caption = other.m_caption;
m_color = other.m_color;
m_status = other.m_status;
cout << "Copy constructor called" << endl;
}
// 析构函数
~CMyButton() {
cout << "Destructor called" << endl;
}
// 修改宽度
void setWidth(int width) {
if (m_status) {
m_width = width;
}
}
// 修改高度
void setHeight(int height) {
if (m_status) {
m_height = height;
}
}
// 修改显示文字
void setCaption(string caption) {
if (m_status) {
m_caption = caption;
}
}
// 修改背景颜色
void setColor(string color) {
if (m_status) {
m_color = color;
}
}
// 修改状态
void setStatus(bool status) {
m_status = status;
}
// 显示按钮信息
void showInfo() {
cout << "Width: " << m_width << endl;
cout << "Height: " << m_height << endl;
cout << "Caption: " << m_caption << endl;
cout << "Color: " << m_color << endl;
cout << "Status: " << m_status << endl;
}
};
int main() {
// 使用默认构造函数构造对象btn1
CMyButton btn1;
btn1.showInfo();
// 使用带参数的构造函数构造对象btn2
CMyButton btn2(200, 100, "OK", "green", true);
btn2.showInfo();
// 使用拷贝构造函数构造对象btn3
CMyButton btn3 = btn1;
btn3.showInfo();
return 0;
}
```
运行程序,输出的结果如下:
```
Default constructor called
Width: 100
Height: 50
Caption: Button
Color: white
Status: 1
Constructor called with parameters
Width: 200
Height: 100
Caption: OK
Color: green
Status: 1
Copy constructor called
Width: 100
Height: 50
Caption: Button
Color: white
Status: 1
Destructor called
Destructor called
Destructor called
```
其中,btn1是使用默认构造函数构造的对象,btn2是使用带参数的构造函数构造的对象,btn3是使用拷贝构造函数构造的对象。我们可以看到,当对象被创建时,会调用对应的构造函数输出构造信息;当对象被销毁时,会调用析构函数输出析构信息。
阅读全文
相关推荐






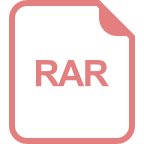
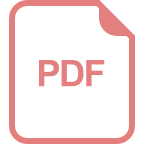







