给我一段c++代码,完成五子棋游戏,要求包含三种游戏模式:人人对战,人机对战(机器先下),人机对战(人先下))
时间: 2024-02-02 10:03:19 浏览: 17
以下是一个简单的五子棋游戏的 C++ 代码,包含了三种游戏模式:
```c++
#include<iostream>
#include<cstring>
#include<cstdlib>
using namespace std;
const int MAXN = 20;
const int INF = 1e9;
int n;
char mp[MAXN][MAXN];
int val[MAXN][MAXN];
int dx[4] = {1, 0, -1, 1};
int dy[4] = {0, 1, 1, 1};
void init(){
for(int i = 0; i < n; i++){
for(int j = 0; j < n; j++){
mp[i][j] = ' ';
val[i][j] = 0;
}
}
}
void draw_map(){
for(int i = 0; i < n; i++){
cout << i+1 << " ";
for(int j = 0; j < n; j++){
cout << mp[i][j] << " ";
}
cout << endl;
}
cout << " ";
for(int i = 1; i <= n; i++){
cout << i << " ";
}
cout << endl;
}
inline bool check(int x, int y){
if(x < 0 || x >= n || y < 0 || y >= n) return false;
if(mp[x][y] != ' ') return false;
return true;
}
int evaluation(){
int res = 0;
for(int i = 0; i < n; i++){
for(int j = 0; j < n; j++){
if(mp[i][j] == 'O'){
for(int k = 0; k < 4; k++){
int cnt = 1;
int nx = i + dx[k], ny = j + dy[k];
while(check(nx, ny) && mp[nx][ny] == 'O'){
cnt++; nx += dx[k]; ny += dy[k];
}
if(cnt >= 5) return INF;
res += val[cnt][0];
}
}
if(mp[i][j] == 'X'){
for(int k = 0; k < 4; k++){
int cnt = 1;
int nx = i + dx[k], ny = j + dy[k];
while(check(nx, ny) && mp[nx][ny] == 'X'){
cnt++; nx += dx[k]; ny += dy[k];
}
if(cnt >= 5) return -INF;
res -= val[cnt][0];
}
}
}
}
return res;
}
int alpha_beta(int dep, int alpha, int beta, bool flag){
if(dep == 0) return evaluation();
int res = flag ? -INF : INF;
for(int i = 0; i < n; i++){
for(int j = 0; j < n; j++){
if(mp[i][j] == ' '){
if(flag){
mp[i][j] = 'O';
res = max(res, alpha_beta(dep-1, alpha, beta, false));
alpha = max(alpha, res);
}
else{
mp[i][j] = 'X';
res = min(res, alpha_beta(dep-1, alpha, beta, true));
beta = min(beta, res);
}
mp[i][j] = ' ';
if(alpha >= beta) return res;
}
}
}
return res;
}
void player_vs_player(){
cout << "Player 1 is O, Player 2 is X." << endl;
int cnt = 0;
while(cnt < n*n){
int x, y;
if(cnt%2 == 0) cout << "Player 1's turn: ";
else cout << "Player 2's turn: ";
cin >> x >> y;
x--; y--;
if(check(x, y)){
if(cnt%2 == 0) mp[x][y] = 'O';
else mp[x][y] = 'X';
draw_map();
int res = evaluation();
if(res == INF){
cout << "Player 1 wins!" << endl;
return;
}
if(res == -INF){
cout << "Player 2 wins!" << endl;
return;
}
cnt++;
}
else{
cout << "Invalid input, please try again." << endl;
}
}
cout << "It's a tie!" << endl;
}
void player_vs_computer(bool flag){
cout << (flag ? "You are O, computer is X." : "You are X, computer is O.") << endl;
int cnt = 0;
while(cnt < n*n){
int x, y;
if(cnt%2 == flag){
cout << "Your turn: ";
cin >> x >> y;
x--; y--;
if(check(x, y)){
mp[x][y] = flag ? 'O' : 'X';
draw_map();
int res = evaluation();
if(res == INF){
cout << "You win!" << endl;
return;
}
if(res == -INF){
cout << "Computer wins!" << endl;
return;
}
cnt++;
}
else{
cout << "Invalid input, please try again." << endl;
}
}
else{
int maxv = -INF;
for(int i = 0; i < n; i++){
for(int j = 0; j < n; j++){
if(mp[i][j] == ' '){
mp[i][j] = flag ? 'X' : 'O';
int tmp = alpha_beta(5, -INF, INF, !flag);
mp[i][j] = ' ';
if(tmp > maxv){
maxv = tmp;
x = i; y = j;
}
}
}
}
cout << "Computer's turn: " << x+1 << " " << y+1 << endl;
mp[x][y] = flag ? 'X' : 'O';
draw_map();
int res = evaluation();
if(res == INF){
cout << "You win!" << endl;
return;
}
if(res == -INF){
cout << "Computer wins!" << endl;
return;
}
cnt++;
}
}
cout << "It's a tie!" << endl;
}
void computer_vs_player(bool flag){
cout << (flag ? "Computer is O, you are X." : "Computer is X, you are O.") << endl;
int cnt = 0;
while(cnt < n*n){
int x, y;
if(cnt%2 == flag){
int maxv = -INF;
for(int i = 0; i < n; i++){
for(int j = 0; j < n; j++){
if(mp[i][j] == ' '){
mp[i][j] = flag ? 'X' : 'O';
int tmp = alpha_beta(5, -INF, INF, !flag);
mp[i][j] = ' ';
if(tmp > maxv){
maxv = tmp;
x = i; y = j;
}
}
}
}
cout << "Computer's turn: " << x+1 << " " << y+1 << endl;
mp[x][y] = flag ? 'O' : 'X';
draw_map();
int res = evaluation();
if(res == INF){
cout << "Computer wins!" << endl;
return;
}
if(res == -INF){
cout << "You win!" << endl;
return;
}
cnt++;
}
else{
cout << "Your turn: ";
cin >> x >> y;
x--; y--;
if(check(x, y)){
mp[x][y] = flag ? 'X' : 'O';
draw_map();
int res = evaluation();
if(res == INF){
cout << "Computer wins!" << endl;
return;
}
if(res == -INF){
cout << "You win!" << endl;
return;
}
cnt++;
}
else{
cout << "Invalid input, please try again." << endl;
}
}
}
cout << "It's a tie!" << endl;
}
int main(){
cout << "Please input the size of the chessboard (maximum 20): ";
cin >> n;
init();
draw_map();
while(true){
cout << "Please choose the game mode: " << endl;
cout << "1. Player vs Player" << endl;
cout << "2. Player vs Computer (computer goes first)" << endl;
cout << "3. Player vs Computer (player goes first)" << endl;
int mode;
cin >> mode;
if(mode == 1){
player_vs_player();
}
else if(mode == 2){
player_vs_computer(true);
}
else if(mode == 3){
computer_vs_player(true);
}
else{
cout << "Invalid input, please try again." << endl;
}
cout << "Would you like to play again? (y/n)" << endl;
char c;
cin >> c;
if(c != 'y' && c != 'Y') break;
init();
draw_map();
}
return 0;
}
```
在该代码中,我们使用了 alpha-beta 剪枝算法来实现了简单的 AI。注意到这个 AI 的效率并不高,对于较大的棋盘或深度,可能需要更高效的算法进行优化。
相关推荐
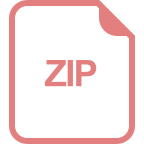
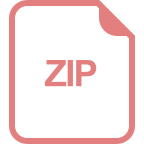














