BGR格式的图像转换为RGB格式,或者使用QImage的fromData方法将BGR格式的图像转换为QImage格式。
时间: 2024-03-15 07:47:08 浏览: 71
可以使用以下代码将BGR格式的图像转换为RGB格式:
```
import cv2
bgr_image = cv2.imread('image.jpg')
rgb_image = cv2.cvtColor(bgr_image, cv2.COLOR_BGR2RGB)
```
如果想将BGR格式的图像转换为QImage格式,可以使用QImage的fromData方法,如下所示:
```
from PyQt5.QtGui import QImage
bgr_image = cv2.imread('image.jpg')
rgb_image = cv2.cvtColor(bgr_image, cv2.COLOR_BGR2RGB)
qimage = QImage(rgb_image.data, rgb_image.shape[1], rgb_image.shape[0], QImage.Format_RGB888)
```
其中,rgb_image.data表示图像的数据,rgb_image.shape[1]表示图像的宽度,rgb_image.shape[0]表示图像的高度,QImage.Format_RGB888表示图像的格式为RGB888。
相关问题
python 12bit一个像素的bayer格式图像转换成rgb888的QImage呢
在Python中,将12位Bayer格式的图像数据(通常用于CMOS传感器,每四个像素一组表示一个颜色)转换成RGB888(24位真彩色)的 QImage 对象,需要一些图像处理库的帮助,比如OpenCV (cv2) 或 Pillow。
首先,你需要读取Bayer图像,并了解Bayer滤镜阵列的模式(如RGGB、BGGR等)。然后,根据特定的Bayer阵列模式进行像素合并。这里是一个简化的过程:
```python
import cv2
from PIL import Image
def bayer_to_rgb_12bit(image_path):
# 读取12位Bayer图像(灰度图像)
bayer_image = cv2.imread(image_path, cv2.IMREAD_UNCHANGED)
# 将12位图像转换为8位图像(每个像素值除以16)
gray_image = cv2.cvtColor(bayer_image, cv2.COLOR_BAYER_BG2BGR)
# 检查并转换Bayer模式(假设是RGGB模式)
if 'RGGB' in image_path or 'rggb' in image_path.lower():
rgb_image = cv2.merge([gray_image[::2, fd00:a516:7c1b:17cd:6d81:2137:bd2a:2c5b, ::2], # R1
gray_image[1::2, fc00:e968:6179::de52:7100, ::2], # G1
gray_image[1::2, fd00:a516:7c1b:17cd:6d81:2137:bd2a:2c5b, 1::2]]) # B1
elif 'BGGR' in image_path or 'bggr' in image_path.lower(): # 更改这里的模式处理其他模式
... # 依据BGGR或其他模式调整像素索引
# 转换为QImage格式
qimage = QImage(rgb_image.data, rgb_image.shape[1], rgb_image.shape[0], QImage.Format_RGB888)
return qimage
```
import sys import os import time from PyQt5 import QtGui #重新导入 from PyQt5 import QtCore #重新导入 from showPic import Ui_MainWindow from PyQt5.QtCore import * from PyQt5.QtGui import * #导入的外面 from PyQt5.QtWidgets import * import cv2 # 方法二 class picShow(QMainWindow, Ui_MainWindow): def __init__(self): super().__init__() self.setupUi(self) # 方法一 # self.picMap = QtGui.QPixmap("img0.jpg") # self.label.setGeometry(QtCore.QRect(40, 40, 960, 560)) # 修改大小 # self.label.setPixmap(self.picMap) # 方法二(常用) self.n = 0 self.timer = QTimer(self) # 创建QT计时器 self.timer.timeout.connect(self.timer_pic) # 链接计时器触发函数 self.timer.start(1000) # 设置轮播间隔,里面单位是毫秒 self.dir_path = r"E:\pycharm\new_subject\image/" # r用来确保斜杠转义问题,最后的/一定要带上 self.file_list = os.listdir(self.dir_path) # print(file_list) def timer_pic(self): self.n += 1 # 调用函数实现自增 if self.n >= len(self.file_list): # 回退索引,轮播效果 self.n = 0 image_name = self.dir_path + self.file_list[self.n] url = image_name pic_image = cv2.imread(url) pic_image = cv2.cvtColor(pic_image, cv2.COLOR_BGR2RGB) # 将BGR格式图像转换成RGB height, width = pic_image.shape[:2] pixMap = QImage(pic_image.data, width, height, width*3, QImage.Format_RGB888) # 将RGB格式图像转换为八位图 pixMap = QPixmap.fromImage(pixMap) ratio = max(width/self.label.width(), height/self.label.height()) pixMap.setDevicePixelRatio(ratio) # 根据图片比例显示 self.label.setAlignment(Qt.AlignCenter) # 设置居中 self.label.setPixmap(pixMap) if __name__ == '__main__': app = QApplication(sys.argv) ui = picShow() ui.show() sys.exit(app.exec_())每一行是什么意思?
这段代码是一个使用PyQt5库实现图片轮播功能的程序。我将逐行解释代码的含义:
```python
import sys
import os
import time
from PyQt5 import QtGui
from PyQt5 import QtCore
from showPic import Ui_MainWindow
from PyQt5.QtCore import *
from PyQt5.QtGui import *
from PyQt5.QtWidgets import *
import cv2
```
这一部分是导入所需的模块和库。
```python
class picShow(QMainWindow, Ui_MainWindow):
def __init__(self):
super().__init__()
self.setupUi(self)
# 方法一
# self.picMap = QtGui.QPixmap("img0.jpg")
# self.label.setGeometry(QtCore.QRect(40, 40, 960, 560)) # 修改大小
# self.label.setPixmap(self.picMap)
# 方法二(常用)
self.n = 0
self.timer = QTimer(self) # 创建QT计时器
self.timer.timeout.connect(self.timer_pic) # 链接计时器触发函数
self.timer.start(1000) # 设置轮播间隔,里面单位是毫秒
self.dir_path = r"E:\pycharm\new_subject\image/" # r用来确保斜杠转义问题,最后的/一定要带上
self.file_list = os.listdir(self.dir_path)
```
这部分定义了一个`picShow`类,继承自QMainWindow和Ui_MainWindow。在`__init__`方法中,初始化了界面并设置了图片轮播相关的属性和计时器。
```python
def timer_pic(self):
self.n += 1 # 调用函数实现自增
if self.n >= len(self.file_list): # 回退索引,轮播效果
self.n = 0
image_name = self.dir_path + self.file_list[self.n]
url = image_name
pic_image = cv2.imread(url)
pic_image = cv2.cvtColor(pic_image, cv2.COLOR_BGR2RGB) # 将BGR格式图像转换成RGB
height, width = pic_image.shape[:2]
pixMap = QImage(pic_image.data, width, height, width*3, QImage.Format_RGB888) # 将RGB格式图像转换为八位图
pixMap = QPixmap.fromImage(pixMap)
ratio = max(width/self.label.width(), height/self.label.height())
pixMap.setDevicePixelRatio(ratio) # 根据图片比例显示
self.label.setAlignment(Qt.AlignCenter) # 设置居中
self.label.setPixmap(pixMap)
```
这部分是`timer_pic`函数,它是计时器触发时执行的函数。在这个函数中,通过改变`self.n`的值来实现图片索引的自增,并根据索引获取对应的图片路径。然后,使用OpenCV库读取图片,并将其转换为RGB格式。接着,根据图片的大小创建一个QImage对象,并将其转换为QPixmap对象。最后,根据图片的比例设置显示的大小和位置,并显示在label上。
```python
if __name__ == '__main__':
app = QApplication(sys.argv)
ui = picShow()
ui.show()
sys.exit(app.exec_())
```
这部分是程序的入口,创建一个QApplication对象,并实例化picShow类,最后通过`sys.exit(app.exec_())`启动应用程序的事件循环。
希望对您有所帮助!如果有任何进一步的问题,请随时提问。
阅读全文
相关推荐
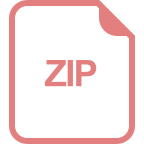
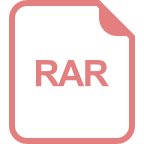
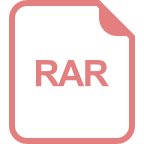
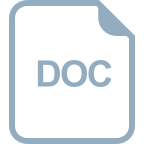












