将摄像头画面转换为QPixmap并显示在QLabel上
时间: 2024-05-13 22:15:48 浏览: 7
可以使用OpenCV库将摄像头的画面转换为QPixmap并显示在QLabel上,具体步骤如下:
1. 导入必要的库:
```python
import sys
import cv2
from PyQt5.QtGui import QPixmap
from PyQt5.QtWidgets import QApplication, QLabel
from PyQt5.QtCore import QTimer
```
2. 创建一个QApplication对象和一个QLabel对象:
```python
app = QApplication(sys.argv)
label = QLabel()
```
3. 创建一个定时器,用于定时从摄像头获取画面:
```python
timer = QTimer()
timer.timeout.connect(show_camera)
timer.start(30) # 定时器间隔为30毫秒
```
4. 创建show_camera函数,用于从摄像头获取画面并将其转换为QPixmap并显示在QLabel上:
```python
def show_camera():
cap = cv2.VideoCapture(0) # 打开摄像头
ret, frame = cap.read() # 读取摄像头画面
if ret:
rgb_image = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB) # 将BGR格式转换为RGB格式
h, w, ch = rgb_image.shape # 获取图像尺寸
q_image = QImage(rgb_image.data, w, h, ch * w, QImage.Format_RGB888) # 创建QImage对象
pixmap = QPixmap.fromImage(q_image) # 将QImage对象转换为QPixmap对象
label.setPixmap(pixmap) # 显示QPixmap对象
cap.release() # 释放摄像头
```
5. 启动应用程序:
```python
label.show()
sys.exit(app.exec_())
```
完整代码如下:
```python
import sys
import cv2
from PyQt5.QtGui import QPixmap
from PyQt5.QtWidgets import QApplication, QLabel
from PyQt5.QtCore import QTimer
app = QApplication(sys.argv)
label = QLabel()
def show_camera():
cap = cv2.VideoCapture(0) # 打开摄像头
ret, frame = cap.read() # 读取摄像头画面
if ret:
rgb_image = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB) # 将BGR格式转换为RGB格式
h, w, ch = rgb_image.shape # 获取图像尺寸
q_image = QImage(rgb_image.data, w, h, ch * w, QImage.Format_RGB888) # 创建QImage对象
pixmap = QPixmap.fromImage(q_image) # 将QImage对象转换为QPixmap对象
label.setPixmap(pixmap) # 显示QPixmap对象
cap.release() # 释放摄像头
timer = QTimer()
timer.timeout.connect(show_camera)
timer.start(30)
label.show()
sys.exit(app.exec_())
```
相关推荐
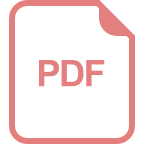
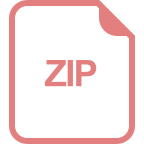
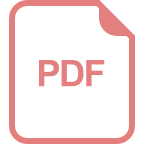














