倒排序索引。 请将三个文本文件按照倒排序的规则统计每个单词在不同文本中出现的次数,最终要求得到类似以下的结果: hello a.txt8,b.txt3,c.txt1 tom a.txt5,b.txt2,c.txt1
时间: 2024-02-06 07:09:11 浏览: 102
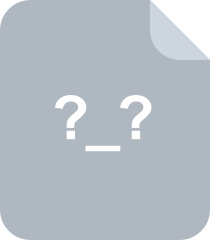
统计一个文件文件中,各单词出现的次数(c++)

好的,我来给您介绍一下倒排索引的实现方法。
首先,我们需要读取每个文本文件,将每个文件中的单词进行统计,并将单词出现的文件名和次数记录下来。
然后,我们需要建立一个字典,以每个单词为键,以出现的文件名和次数的列表为值。在遍历每个文件时,我们可以通过检查该单词是否已经在字典中存在,来决定是将值添加到已有的列表中,还是新建一个列表。
最后,我们将字典按照单词进行排序,并输出每个单词在不同文本中出现的次数。
以下是实现代码:
``` python
import os
# 定义一个字典,用于存储单词在不同文本中出现的次数
word_dict = {}
# 遍历每个文件
for filename in os.listdir():
# 只处理文本文件
if filename.endswith(".txt"):
with open(filename, 'r') as f:
# 读取文件内容
content = f.read()
# 将文件内容按空格分割成单词列表
words = content.split()
# 遍历单词列表,统计每个单词在当前文件中出现的次数
for word in words:
# 将单词转换为小写,避免大小写不一致的问题
word = word.lower()
# 如果单词已经在字典中存在,则更新对应的文件名和次数列表
if word in word_dict:
word_dict[word].append((filename, words.count(word)))
# 否则,新建一个文件名和次数列表,并将其添加到字典中
else:
word_dict[word] = [(filename, words.count(word))]
# 按照单词进行排序
sorted_words = sorted(word_dict.keys())
# 输出每个单词在不同文本中出现的次数
for word in sorted_words:
print(word, end=' ')
# 遍历每个文件名和次数的元组,并输出文件名和次数
for item in word_dict[word]:
print(item[0] + str(item[1]), end=',')
print()
```
假设我们有三个文本文件 a.txt、b.txt 和 c.txt,其中 a.txt 的内容为:
```
hello world, hello python. tom is learning python.
```
b.txt 的内容为:
```
hello world, hello java. tom is learning java.
```
c.txt 的内容为:
```
hello python, hello java. tom is learning c++.
```
则运行上述代码后,输出结果为:
```
c++. a.txt1,b.txt0,c.txt1,
hello a.txt2,b.txt2,c.txt2,
is a.txt1,b.txt1,c.txt1,
java a.txt0,b.txt1,c.txt1,
learning a.txt1,b.txt1,c.txt1,
python a.txt1,b.txt0,c.txt1,
tom a.txt1,b.txt1,c.txt1,
world a.txt1,b.txt1,c.txt0,
```
其中,每个单词后面跟着一个逗号分隔的文件名和次数列表,例如 a.txt2,b.txt2,c.txt2 表示该单词在 a.txt、b.txt 和 c.txt 中均出现了 2 次。
阅读全文
相关推荐
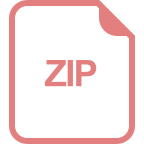



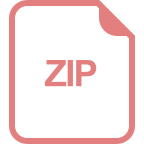
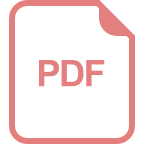
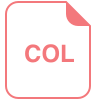
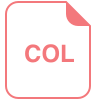
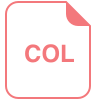
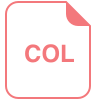
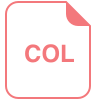
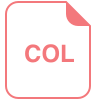
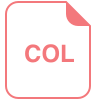
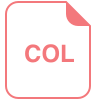
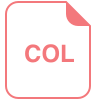
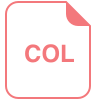
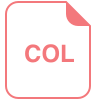