编写一算法实现在非递减的顺序表上插入数据元素X,并使得顺序表保持有序状态
时间: 2024-09-23 21:14:04 浏览: 70
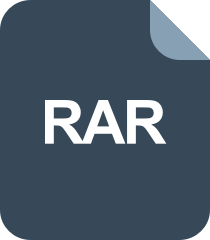
shunxubiao.rar_shunxubiao_输入一组整型元素序列,建立顺序表

在非递减的顺序表(也称为升序列表)上插入数据元素X并保持有序状态,可以采用以下步骤编写简单的插入排序算法:
1. **查找插入位置**:
- 初始化一个变量`insertIndex`为0,表示开始搜索的位置。
- 遍历顺序表从第一个元素开始,如果当前元素小于X,说明X应该在这个元素之后,将`insertIndex`加1。
- 当遍历到某个元素大于等于X时或者到达了列表的末尾,`insertIndex`就是X应插入的位置。
2. **插入新元素**:
- 将顺序表的最后一个元素(如果有)移动到`insertIndex`后面,腾出空间。
- 将数据元素X放置在`insertIndex`位置。
以下是这个过程的伪代码示例:
```python
function insertSortedList(head, X):
// 初始化头指针和插入指针
let head = head
let insertIndex = 0
while (head != null && head.value <= X) {
head = head.next
insertIndex++
}
// 创建一个新的节点
let newNode = new Node(X)
// 插入新节点
if (head == null) {
head = newNode
} else {
newNode.next = head
head = newNode
}
// 如果需要,将后续元素向右移一位
for (let i = insertIndex; i < listLength; i++) {
list[i] = list[i + 1]
}
list[insertIndex] = X
return head
```
阅读全文
相关推荐
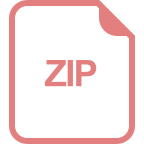
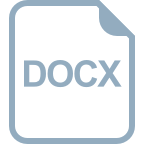








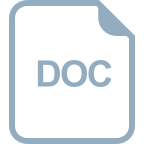






