计算器实现运算符优先级
时间: 2023-12-08 14:04:30 浏览: 24
计算器可以通过使用栈来实现运算符优先级的计算。具体实现步骤如下:
1. 创建两个栈,一个栈用来存放数字,一个栈用来存放运算符。
2. 从左到右遍历表达式,遇到数字时将其压入数字栈中。
3. 遇到运算符时,将其与运算符栈栈顶的运算符进行比较。
4. 如果当前运算符的优先级小于等于栈顶运算符的优先级,则将数字栈中的两个数字弹出,将栈顶运算符弹出并进行运算,将运算结果压入数字栈中。
5. 如果当前运算符的优先级大于栈顶运算符的优先级,则将当前运算符入栈。
6. 遍历完表达式后,依次弹出运算符栈中的运算符并进行运算,将运算结果压入数字栈中,直到运算符栈为空。
7. 最终数字栈中的唯一元素即为表达式的运算结果。
以下是一个示例代码实现:
```python
def calculate(expression):
num_stack = []
operator_stack = []
operators = {'+': 1, '-': 1, '*': 2, '/': 2, '(': 0}
for char in expression:
if char.isdigit():
num_stack.append(int(char))
elif char in operators:
while operator_stack and operators[char] <= operators[operator_stack[-1]]:
num2 = num_stack.pop()
num1 = num_stack.pop()
operator = operator_stack.pop()
if operator == '+':
num_stack.append(num1 + num2)
elif operator == '-':
num_stack.append(num1 - num2)
elif operator == '*':
num_stack.append(num1 * num2)
elif operator == '/':
num_stack.append(num1 / num2)
if char != ')':
operator_stack.append(char)
elif char == ')':
while operator_stack[-1] != '(':
num2 = num_stack.pop()
num1 = num_stack.pop()
operator = operator_stack.pop()
if operator == '+':
num_stack.append(num1 + num2)
elif operator == '-':
num_stack.append(num1 - num2)
elif operator == '*':
num_stack.append(num1 * num2)
elif operator == '/':
num_stack.append(num1 / num2)
operator_stack.pop()
while operator_stack:
num2 = num_stack.pop()
num1 = num_stack.pop()
operator = operator_stack.pop()
if operator == '+':
num_stack.append(num1 + num2)
elif operator == '-':
num_stack.append(num1 - num2)
elif operator == '*':
num_stack.append(num1 * num2)
elif operator == '/':
num_stack.append(num1 / num2)
return num_stack.pop()
```
这个函数接受一个表达式作为参数,并返回表达式的运算结果。例如,calculate("3+4*5-(6/2)")的返回值为17。
相关推荐
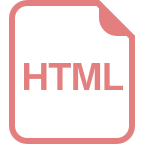
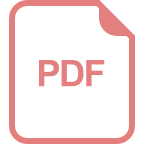












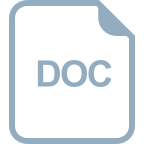
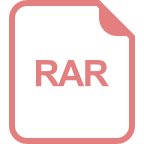
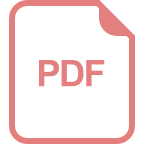