always@(posedge clk or negedge rst_n)begin if(!rst_n) led <= {{(LED - 1){1'b1}},1'b0}; else if(counter == COUNT_TIME - 1) led <= {led[LED - 2:0],led[LED - 1]};//使用位拼接的方法实现LED灯的切换 else led <= led; end
时间: 2024-04-18 16:25:03 浏览: 64
这段代码是一个Verilog的always块,它描述了一个时序逻辑行为。根据时钟的上升沿(posedge clk)以及复位信号的下降沿(negedge rst_n)触发,在每个时钟周期内执行以下逻辑:
1. 如果复位信号(!rst_n)为低电平(即复位激活),则将LED寄存器的值设为 `{{(LED - 1){1'b1}},1'b0}`。这里使用了花括号和重复拷贝操作符来生成一个具有特定位数的二进制数,其中最高位有 (LED - 1) 个1,其余位都为0。
2. 否则,如果计数器(counter)的值等于 COUNT_TIME - 1,将LED寄存器的值更新为 `{led[LED - 2:0],led[LED - 1]}`。这里使用了位切片(bit slicing)操作符来从LED寄存器中取出特定位数的值,并进行位拼接操作。
3. 如果以上条件都不满足,则将LED寄存器的值保持不变。
总体来说,这段代码的作用是实现LED灯的切换逻辑。具体的功能实现还需要根据代码中未给出的部分进行完善。
相关问题
always @(posedge clk or negedge rst_n) if(rst_n) clk_1 <= 1'b0; else clk_1 <= clk_0; always @(posedge clk or negedge rst_n) if(rst_n) clk_2 <= 1'b0; else clk_2 <= clk_1; always @(posedge clk or negedge rst_n) if(rst_n) clk_3 <= 1'b0; else clk_3 <= clk_2;
这段代码展示了三个时钟信号(clk_1, clk_2, clk_3)的递推关系。当复位信号 rst_n 为高电平时,所有时钟信号都被重置为低电平。否则,每个时钟信号都是前一个时钟信号的延迟版本。也就是说,clk_1 是 clk_0 的延迟版本,clk_2 是 clk_1 的延迟版本,以此类推。这种递推关系可以用于时钟延迟和同步电路设计中。请问有什么问题我可以帮助您解答的吗?
module crc8( data_in, clk, rst_n, crc7, crc6, crc5, crc4, crc3, crc2, crc1, crc0 ); input wire data_in; input wire clk; input wire rst_n; output wire crc7; output wire crc6; output wire crc5; output wire crc4; output wire crc3; output wire crc2; output wire crc1; output wire crc0; wire SYNTHESIZED_WIRE_5; reg DFF_inst8; reg DFF_inst; wire SYNTHESIZED_WIRE_2; wire SYNTHESIZED_WIRE_3; reg DFF_inst3; reg DFF_inst4; reg DFF_inst5; reg DFF_inst6; reg DFF_inst7; reg DFF_inst2; assign crc7 = DFF_inst8; assign crc6 = DFF_inst7; assign crc5 = DFF_inst6; assign crc4 = DFF_inst5; assign crc3 = DFF_inst4; assign crc2 = DFF_inst3; assign crc1 = DFF_inst2; assign crc0 = DFF_inst; always@(posedge clk or negedge rst_n) begin if (!rst_n) begin DFF_inst <= 1; end else begin DFF_inst <= SYNTHESIZED_WIRE_5; end end assign SYNTHESIZED_WIRE_5 = data_in ^ DFF_inst8; assign SYNTHESIZED_WIRE_2 = SYNTHESIZED_WIRE_5 ^ DFF_inst; always@(posedge clk or negedge rst_n) begin if (!rst_n) begin DFF_inst2 <= 1; end else begin DFF_inst2 <= SYNTHESIZED_WIRE_2; end end always@(posedge clk or negedge rst_n) begin if (!rst_n) begin DFF_inst3 <= 1; end else begin DFF_inst3 <= SYNTHESIZED_WIRE_3; end end always@(posedge clk or negedge rst_n) begin if (!rst_n) begin DFF_inst4 <= 1; end else begin DFF_inst4 <= DFF_inst3; end end always@(posedge clk or negedge rst_n) begin if (!rst_n) begin DFF_inst5 <= 1; end else begin DFF_inst5 <= DFF_inst4; end end always@(posedge clk or negedge rst_n) begin if (!rst_n) begin DFF_inst6 <= 1; end else begin DFF_inst6 <= DFF_inst5; end end always@(posedge clk or negedge rst_n) begin if (!rst_n) begin DFF_inst7 <= 1; end else begin DFF_inst7 <= DFF_inst6; end end always@(posedge clk or negedge rst_n) begin if (!rst_n) begin DFF_inst8 <= 1; end else begin DFF_inst8 <= DFF_inst7; end end assign SYNTHESIZED_WIRE_3 = SYNTHESIZED_WIRE_5 ^ DFF_inst2; endmodule 把这个代码修改成CRC-12编码器
好的,这里是修改后的CRC-12编码器代码,其中使用的CRC多项式为x^12 + x^11 + x^3 + x^2 + x + 1:
```
module crc12(
data_in,
clk,
rst_n,
crc11,
crc10,
crc9,
crc8,
crc7,
crc6,
crc5,
crc4,
crc3,
crc2,
crc1,
crc0
);
input wire data_in;
input wire clk;
input wire rst_n;
output wire crc11;
output wire crc10;
output wire crc9;
output wire crc8;
output wire crc7;
output wire crc6;
output wire crc5;
output wire crc4;
output wire crc3;
output wire crc2;
output wire crc1;
output wire crc0;
wire [11:0] crc;
wire [11:0] crc_next;
wire [11:0] data;
assign data = {data_in, 6'b0};
always@(posedge clk or negedge rst_n) begin
if (!rst_n) begin
crc <= 12'hFFF;
end
else begin
crc <= crc_next;
end
end
assign crc11 = crc[11];
assign crc10 = crc[10];
assign crc9 = crc[9];
assign crc8 = crc[8];
assign crc7 = crc[7];
assign crc6 = crc[6];
assign crc5 = crc[5];
assign crc4 = crc[4];
assign crc3 = crc[3];
assign crc2 = crc[2];
assign crc1 = crc[1];
assign crc0 = crc[0];
assign crc_next[11:0] = {crc[10:0], 1'b0} ^ (data << 4) ^ (data << 1) ^ data;
endmodule
```
这个代码使用了一个12位的寄存器crc来保存当前的CRC校验值,每次输入一个数据位时,根据CRC多项式进行异或运算得到下一个CRC值,并将其保存到crc_next中。在时钟上升沿时,将crc_next的值赋给crc寄存器,完成一次CRC计算。最后,根据CRC校验位的位置将crc寄存器的值输出到crc11到crc0中,即可得到CRC-12校验码。
阅读全文
相关推荐
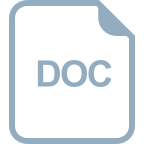
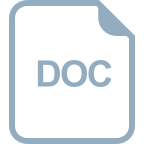
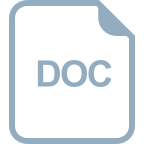













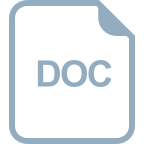