micropython 实现多线程代码示例
时间: 2023-05-21 09:02:01 浏览: 153
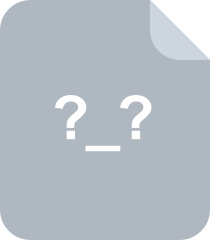
Python 多线程编程实例

以下是一个简单的 Micropython 多线程代码示例:
```python
import _thread
def thread_func(thread_name):
print("Thread %s started" % thread_name)
# 在这里编写线程的代码
print("Thread %s finished" % thread_name)
try:
_thread.start_new_thread(thread_func, ("Thread 1",))
_thread.start_new_thread(thread_func, ("Thread 2",))
except:
print("Error: 无法启动线程")
# 主线程继续执行
print("Main thread finished")
```
这个示例中,我们使用 `_thread` 模块来创建两个线程,每个线程都执行 `thread_func` 函数。在 `thread_func` 函数中,我们可以编写线程的代码。在这个示例中,我们只是简单地打印一些信息。
注意,这个示例只是一个简单的演示,实际的多线程代码可能会更加复杂。在编写多线程代码时,请务必小心处理共享资源和同步问题。
阅读全文
相关推荐




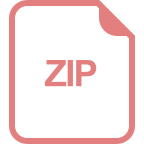








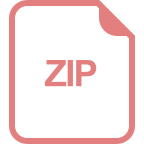
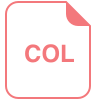
