请写出一段俄罗斯方块的代码
时间: 2023-08-31 08:05:20 浏览: 109
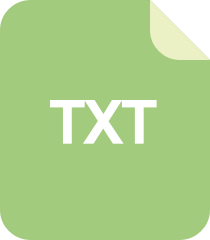
俄罗斯方块的代码
当然可以,以下是一个简单的俄罗斯方块代码示例:
```python
import pygame
import random
# 定义颜色
WHITE = (255, 255, 255)
GREY = (128, 128, 128)
BLACK = (0, 0, 0)
RED = (255, 0, 0)
CYAN = (0, 255, 255)
ORANGE = (255, 165, 0)
YELLOW = (255, 255, 0)
GREEN = (0, 128, 0)
PURPLE = (128, 0, 128)
# 定义方块形状
shapes = [
[[[0, 1, 0], [1, 1, 1], [0, 0, 0]], ORANGE], # L形
[[[1, 1, 1], [0, 1, 0], [0, 0, 0]], CYAN], # T形
[[[0, 0, 1], [1, 1, 1], [0, 0, 0]], BLUE], # J形
[[[1, 1], [1, 1]], YELLOW], # 方块
[[[0, 1, 1], [1, 1, 0], [0, 0, 0]], GREEN], # S形
[[[1, 1, 0], [0, 1, 1], [0, 0, 0]], PURPLE], # Z形
[[[0, 0, 0, 0], [1, 1, 1, 1], [0, 0, 0, 0], [0, 0, 0, 0]], RED] # 直线形
]
# 初始化pygame
pygame.init()
# 设置游戏窗口大小
screen_width = 640
screen_height = 480
screen = pygame.display.set_mode([screen_width, screen_height])
# 设置游戏标题
pygame.display.set_caption("俄罗斯方块")
# 设置游戏时钟
clock = pygame.time.Clock()
# 定义游戏数据
board_width = 10
board_height = 20
board = [[0 for x in range(board_width)] for y in range(board_height)]
current_shape = random.choice(shapes)
current_x = int(board_width / 2 - len(current_shape[0]) / 2)
current_y = 0
next_shape = random.choice(shapes)
# 定义函数
def draw_block(x, y, color):
pygame.draw.rect(screen, color, [x * 20, y * 20, 20, 20])
def draw_shape(shape, x, y, color):
for i in range(len(shape)):
for j in range(len(shape[0])):
if shape[i][j] == 1:
draw_block(x + j, y + i, color)
def move_shape_down():
global current_x, current_y, current_shape
if check_collision(current_shape, current_x, current_y + 1):
add_shape_to_board(current_shape, current_x, current_y)
current_shape = next_shape
next_shape = random.choice(shapes)
current_x = int(board_width / 2 - len(current_shape[0]) / 2)
current_y = 0
else:
current_y += 1
def check_collision(shape, x, y):
for i in range(len(shape)):
for j in range(len(shape[0])):
if shape[i][j] == 1:
if i + y >= board_height or j + x < 0 or j + x >= board_width or board[i + y][j + x] != 0:
return True
return False
def add_shape_to_board(shape, x, y):
for i in range(len(shape)):
for j in range(len(shape[0])):
if shape[i][j] == 1:
board[i + y][j + x] = 1
def draw_board():
for i in range(len(board)):
for j in range(len(board[0])):
if board[i][j] != 0:
draw_block(j, i, shapes[board[i][j] - 1][1])
# 游戏循环
done = False
while not done:
# 事件循环
for event in pygame.event.get():
if event.type == pygame.QUIT:
done = True
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
if not check_collision(current_shape, current_x - 1, current_y):
current_x -= 1
elif event.key == pygame.K_RIGHT:
if not check_collision(current_shape, current_x + 1, current_y):
current_x += 1
elif event.key == pygame.K_UP:
rotated_shape = [[current_shape[j][i] for j in range(len(current_shape))] for i in range(len(current_shape[0]) - 1, -1, -1)]
if not check_collision(rotated_shape, current_x, current_y):
current_shape = rotated_shape
elif event.key == pygame.K_DOWN:
while not check_collision(current_shape, current_x, current_y + 1):
current_y += 1
screen.fill(BLACK)
# 将当前形状加入到游戏面板中
add_shape_to_board(current_shape, current_x, current_y)
# 将游戏面板渲染到屏幕上
draw_board()
# 将下一个形状渲染到屏幕上
draw_shape(next_shape[0], board_width + 2, 2, next_shape[1])
# 将当前形状渲染到屏幕上
draw_shape(current_shape[0], current_x, current_y, current_shape[1])
# 将当前形状从游戏面板中移除
add_shape_to_board(current_shape, current_x, current_y, 0)
# 将游戏面板与当前形状向下移动一格
move_shape_down()
# 刷新屏幕
pygame.display.flip()
# 设置帧率
clock.tick(10)
# 退出游戏
pygame.quit()
```
阅读全文
相关推荐



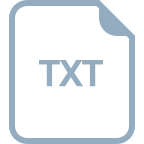
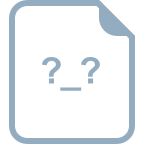
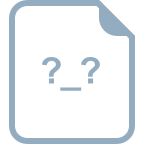
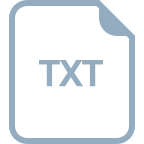
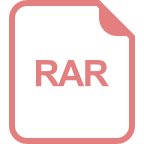
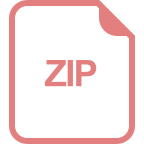
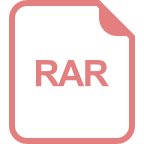
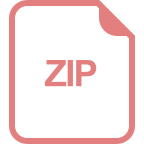
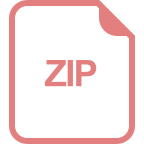
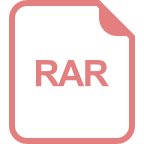
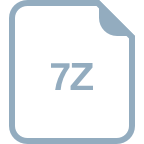