用C语言写一个俄罗斯方块代码
时间: 2023-12-29 20:17:47 浏览: 79
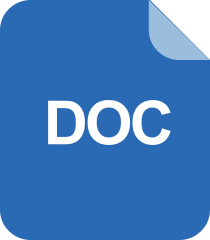
俄罗斯方块C语言代码.doc
以下是一个简单的俄罗斯方块游戏的C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <conio.h>
#include <windows.h>
#define WIDTH 10 // 游戏区域宽度
#define HEIGHT 20 // 游戏区域高度
// 方块种类及形状
int block[7][4][4] = {
{ { 0, 0, 0, 0 }, { 0, 0, 0, 0 }, { 1, 1, 1, 1 }, { 0, 0, 0, 0 } },
{ { 0, 0, 0, 0 }, { 0, 1, 1, 0 }, { 0, 1, 1, 0 }, { 0, 0, 0, 0 } },
{ { 0, 0, 0, 0 }, { 1, 1, 0, 0 }, { 0, 1, 1, 0 }, { 0, 0, 0, 0 } },
{ { 0, 0, 0, 0 }, { 0, 1, 1, 0 }, { 1, 1, 0, 0 }, { 0, 0, 0, 0 } },
{ { 0, 0, 0, 0 }, { 1, 1, 1, 0 }, { 0, 0, 1, 0 }, { 0, 0, 0, 0 } },
{ { 0, 0, 0, 0 }, { 0, 0, 1, 0 }, { 1, 1, 1, 0 }, { 0, 0, 0, 0 } },
{ { 0, 0, 0, 0 }, { 1, 1, 1, 0 }, { 1, 0, 0, 0 }, { 0, 0, 0, 0 } },
};
// 游戏区域
int board[HEIGHT][WIDTH] = { 0 };
// 当前方块的位置和类型
int cur_x, cur_y, cur_type;
// 下一个方块的类型
int next_type;
// 分数和级别
int score, level;
// 游戏是否结束
int gameover = 0;
// 随机生成下一个方块
void generate_next_block()
{
next_type = rand() % 7;
}
// 在游戏区域中画出方块
void draw_block(int x, int y, int type)
{
int i, j;
for (i = 0; i < 4; i++)
{
for (j = 0; j < 4; j++)
{
if (block[type][i][j] == 1)
{
board[y + i][x + j] = type + 1;
}
}
}
}
// 擦除游戏区域中的方块
void erase_block(int x, int y, int type)
{
int i, j;
for (i = 0; i < 4; i++)
{
for (j = 0; j < 4; j++)
{
if (block[type][i][j] == 1)
{
board[y + i][x + j] = 0;
}
}
}
}
// 判断当前位置是否合法
int is_valid_position(int x, int y, int type)
{
int i, j;
for (i = 0; i < 4; i++)
{
for (j = 0; j < 4; j++)
{
if (block[type][i][j] == 1)
{
if (x + j < 0 || x + j >= WIDTH || y + i >= HEIGHT)
{
return 0;
}
if (y + i >= 0 && board[y + i][x + j] != 0)
{
return 0;
}
}
}
}
return 1;
}
// 将当前方块固定到游戏区域中
void fix_block()
{
int i, j;
for (i = 0; i < 4; i++)
{
for (j = 0; j < 4; j++)
{
if (block[cur_type][i][j] == 1)
{
board[cur_y + i][cur_x + j] = cur_type + 1;
}
}
}
}
// 消除满行
void clear_full_rows()
{
int i, j, k;
for (i = HEIGHT - 1; i >= 0; i--)
{
int full = 1;
for (j = 0; j < WIDTH; j++)
{
if (board[i][j] == 0)
{
full = 0;
break;
}
}
if (full)
{
for (k = i; k > 0; k--)
{
for (j = 0; j < WIDTH; j++)
{
board[k][j] = board[k - 1][j];
}
}
score += 100;
i++;
}
}
}
// 显示游戏区域
void display_board()
{
int i, j;
system("cls");
printf("Score: %d, Level: %d\n", score, level);
for (i = 0; i < HEIGHT; i++)
{
for (j = 0; j < WIDTH; j++)
{
if (board[i][j] == 0)
{
printf(".");
}
else
{
printf("*");
}
}
printf("\n");
}
}
// 初始化游戏
void init_game()
{
int i, j;
score = 0;
level = 1;
gameover = 0;
for (i = 0; i < HEIGHT; i++)
{
for (j = 0; j < WIDTH; j++)
{
board[i][j] = 0;
}
}
generate_next_block();
}
// 主函数
int main()
{
int ch, i, j;
srand(time(NULL));
init_game();
while (!gameover)
{
cur_x = WIDTH / 2 - 2;
cur_y = 0;
cur_type = next_type;
generate_next_block();
if (!is_valid_position(cur_x, cur_y, cur_type))
{
gameover = 1;
break;
}
while (1)
{
display_board();
draw_block(cur_x, cur_y, cur_type);
if (kbhit())
{
ch = getch();
if (ch == 'a' || ch == 'A')
{
if (is_valid_position(cur_x - 1, cur_y, cur_type))
{
erase_block(cur_x, cur_y, cur_type);
cur_x--;
}
}
else if (ch == 'd' || ch == 'D')
{
if (is_valid_position(cur_x + 1, cur_y, cur_type))
{
erase_block(cur_x, cur_y, cur_type);
cur_x++;
}
}
else if (ch == 'w' || ch == 'W')
{
int new_type = (cur_type + 1) % 4;
if (is_valid_position(cur_x, cur_y, new_type))
{
erase_block(cur_x, cur_y, cur_type);
cur_type = new_type;
}
}
else if (ch == 's' || ch == 'S')
{
while (is_valid_position(cur_x, cur_y + 1, cur_type))
{
erase_block(cur_x, cur_y, cur_type);
cur_y++;
}
}
}
if (is_valid_position(cur_x, cur_y + 1, cur_type))
{
erase_block(cur_x, cur_y, cur_type);
cur_y++;
}
else
{
fix_block();
clear_full_rows();
break;
}
Sleep(500);
}
}
display_board();
printf("Game Over!\n");
return 0;
}
```
运行后,可以用键盘上的 A、D、W、S 键控制方块的左右移动、旋转和加速下落,直到游戏结束。
阅读全文
相关推荐
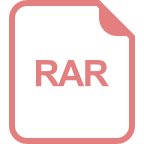














