写一个俄罗斯方块的c语言代码
时间: 2023-12-31 08:05:44 浏览: 23
以下是一个简单的俄罗斯方块的C语言代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <conio.h>
#include <windows.h>
#define WIDTH 10 // 方块区域宽度
#define HEIGHT 20 // 方块区域高度
// 方块结构体
typedef struct {
int x; // 方块左上角x坐标
int y; // 方块左上角y坐标
int shape[4][4]; // 方块形状
} Block;
// 方块区域
int board[HEIGHT][WIDTH] = { 0 };
// 方块的七种类型
int block[7][4][4] = {
{ {0,0,0,0}, {1,1,1,1}, {0,0,0,0}, {0,0,0,0} }, // I型
{ {0,0,0,0}, {0,2,2,0}, {0,2,2,0}, {0,0,0,0} }, // O型
{ {0,0,0,0}, {0,3,3,0}, {3,3,0,0}, {0,0,0,0} }, // S型
{ {0,0,0,0}, {4,4,0,0}, {0,4,4,0}, {0,0,0,0} }, // Z型
{ {0,0,0,0}, {5,5,5,0}, {0,0,5,0}, {0,0,0,0} }, // L型
{ {0,0,0,0}, {0,0,5,0}, {5,5,5,0}, {0,0,0,0} }, // J型
{ {0,0,0,0}, {0,0,6,0}, {0,6,6,6}, {0,0,0,0} } // T型
};
// 随机生成一个方块
Block newBlock() {
Block b;
int type = rand() % 7;
b.x = WIDTH / 2 - 2;
b.y = 0;
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
b.shape[i][j] = block[type][i][j];
}
}
return b;
}
// 画出方块区域
void drawBoard() {
system("cls");
for (int i = 0; i < HEIGHT; i++) {
for (int j = 0; j < WIDTH; j++) {
if (board[i][j] == 0) {
printf(" ");
}
else {
printf("[]");
}
}
printf("\n");
}
}
// 画出方块
void drawBlock(Block b) {
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if (b.shape[i][j] != 0) {
printf("[]");
}
}
printf("\n");
}
}
// 检查方块是否可以移动
int checkMove(Block b, int x, int y) {
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if (b.shape[i][j] != 0) {
if (i + y >= HEIGHT || j + x < 0 || j + x >= WIDTH || board[i + y][j + x] != 0) {
return 0;
}
}
}
}
return 1;
}
// 方块下落
void drop(Block b) {
while (checkMove(b, 0, 1)) {
b.y++;
drawBoard();
drawBlock(b);
Sleep(500);
}
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if (b.shape[i][j] != 0) {
board[i + b.y][j + b.x] = b.shape[i][j];
}
}
}
}
// 消除满行
void clearLine() {
for (int i = HEIGHT - 1; i >= 0; i--) {
int flag = 1;
for (int j = 0; j < WIDTH; j++) {
if (board[i][j] == 0) {
flag = 0;
break;
}
}
if (flag) {
for (int k = i; k > 0; k--) {
for (int j = 0; j < WIDTH; j++) {
board[k][j] = board[k - 1][j];
}
}
i++;
drawBoard();
Sleep(500);
}
}
}
int main() {
srand((unsigned)time(NULL));
Block currentBlock = newBlock();
while (1) {
drawBoard();
drawBlock(currentBlock);
if (!checkMove(currentBlock, 0, 1)) {
drop(currentBlock);
clearLine();
currentBlock = newBlock();
if (!checkMove(currentBlock, 0, 0)) {
printf("Game Over!\n");
break;
}
}
if (_kbhit()) {
int key = _getch();
if (key == 75 && checkMove(currentBlock, -1, 0)) { // 左移
currentBlock.x--;
}
else if (key == 77 && checkMove(currentBlock, 1, 0)) { // 右移
currentBlock.x++;
}
else if (key == 80 && checkMove(currentBlock, 0, 1)) { // 下移
currentBlock.y++;
}
else if (key == 32) { // 空格键,直接落地
drop(currentBlock);
clearLine();
currentBlock = newBlock();
if (!checkMove(currentBlock, 0, 0)) {
printf("Game Over!\n");
break;
}
}
}
Sleep(100);
}
return 0;
}
```
该代码使用了Windows API中的`Sleep()`函数,需要在Windows环境下编译运行。
相关推荐
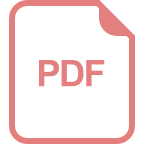













