C++调用glew绘制全向天线水平波瓣图代码
时间: 2023-07-11 07:27:28 浏览: 184
以下是C++调用glew库绘制全向天线水平波瓣图的代码示例:
```c++
#include <GL/glew.h>
#include <GLFW/glfw3.h>
#include <iostream>
#include <cmath>
// 定义窗口大小
const int WIDTH = 800;
const int HEIGHT = 800;
// 定义绘制圆的分辨率
const int RESOLUTION = 100;
// 定义天线的辐射功率模型
double powerModel(double theta) {
// 这里使用简单的cos(theta)的模型,实际中需要根据具体情况进行定义
return std::cos(theta);
}
// 绘制全向天线水平波瓣图
void drawAntenna() {
glBegin(GL_LINE_STRIP);
glColor3f(1.0f, 1.0f, 1.0f);
for (int i = 0; i <= RESOLUTION; i++) {
double theta = i * 2 * M_PI / RESOLUTION;
double power = powerModel(theta);
double x = power * std::cos(theta);
double y = power * std::sin(theta);
glVertex2d(x, y);
}
glEnd();
}
// 窗口大小变化回调函数
void resizeCallback(GLFWwindow* window, int width, int height) {
glViewport(0, 0, width, height);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
double aspectRatio = static_cast<double>(width) / static_cast<double>(height);
if (width >= height) {
glOrtho(-1.0 * aspectRatio, 1.0 * aspectRatio, -1.0, 1.0, -1.0, 1.0);
} else {
glOrtho(-1.0, 1.0, -1.0 / aspectRatio, 1.0 / aspectRatio, -1.0, 1.0);
}
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
}
// 主函数
int main() {
// 初始化GLFW
if (!glfwInit()) {
std::cerr << "Failed to initialize GLFW" << std::endl;
return -1;
}
// 创建窗口
GLFWwindow* window = glfwCreateWindow(WIDTH, HEIGHT, "Antenna Pattern", nullptr, nullptr);
if (!window) {
std::cerr << "Failed to create window" << std::endl;
glfwTerminate();
return -1;
}
// 设置窗口大小变化回调函数
glfwSetWindowSizeCallback(window, resizeCallback);
// 设置当前上下文
glfwMakeContextCurrent(window);
// 初始化GLEW
if (glewInit() != GLEW_OK) {
std::cerr << "Failed to initialize GLEW" << std::endl;
glfwTerminate();
return -1;
}
// 设置视口
glViewport(0, 0, WIDTH, HEIGHT);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
glOrtho(-1.0, 1.0, -1.0, 1.0, -1.0, 1.0);
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
// 渲染循环
while (!glfwWindowShouldClose(window)) {
// 清空缓冲区
glClear(GL_COLOR_BUFFER_BIT);
// 绘制天线
drawAntenna();
// 交换缓冲区
glfwSwapBuffers(window);
// 处理事件
glfwPollEvents();
}
// 清理并退出
glfwTerminate();
return 0;
}
```
这段代码使用OpenGL库绘制全向天线水平波瓣图,使用了GLFW和GLEW库来初始化窗口和OpenGL环境,并使用glOrtho函数设置了正交投影矩阵。在绘制天线时,使用了powerModel函数来计算在不同方向上的辐射功率,并使用glBegin和glEnd函数绘制出天线的形状。最后,使用glfwSwapBuffers函数交换缓冲区来显示绘制结果,并在渲染循环中不断更新窗口。
阅读全文
相关推荐
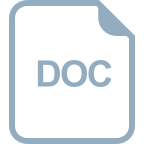
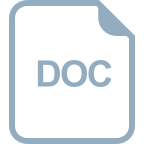
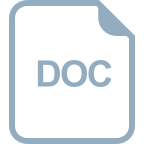






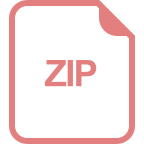
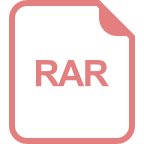
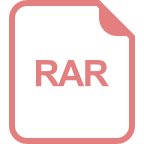
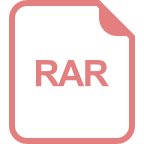
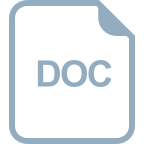
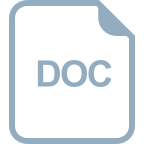



