C++调用glew绘制天线水平方向图(在极坐标上)代码
时间: 2024-03-04 08:52:59 浏览: 25
以下是一个基于 GLEW 的 C++ 示例代码,用于在极坐标上绘制天线水平方向图:
```c++
#include <iostream>
#include <cmath>
#include <GL/glew.h>
#include <GLFW/glfw3.h>
const int WIDTH = 800;
const int HEIGHT = 600;
const float PI = 3.14159265358979323846f;
void error_callback(int error, const char* description)
{
std::cerr << "Error: " << description << std::endl;
}
void key_callback(GLFWwindow* window, int key, int scancode, int action, int mods)
{
if (key == GLFW_KEY_ESCAPE && action == GLFW_PRESS)
glfwSetWindowShouldClose(window, GLFW_TRUE);
}
void drawPolarLine(float r, float theta)
{
float x = r * std::cos(theta);
float y = r * std::sin(theta);
glVertex2f(x, y);
}
void render()
{
glClear(GL_COLOR_BUFFER_BIT);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
glOrtho(-1.2f, 1.2f, -1.2f, 1.2f, -1.0f, 1.0f);
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
glColor3f(1.0f, 0.0f, 0.0f);
glBegin(GL_LINE_STRIP);
float r = 1.0f;
for (float theta = 0.0f; theta <= 2 * PI; theta += PI / 180.0f) {
drawPolarLine(r, theta);
}
glEnd();
glfwSwapBuffers(glfwGetCurrentContext());
}
int main()
{
glfwSetErrorCallback(error_callback);
if (!glfwInit())
return EXIT_FAILURE;
GLFWwindow* window = glfwCreateWindow(WIDTH, HEIGHT, "Antenna Pattern", NULL, NULL);
if (!window)
{
glfwTerminate();
return EXIT_FAILURE;
}
glfwSetKeyCallback(window, key_callback);
glfwMakeContextCurrent(window);
if (glewInit() != GLEW_OK)
return EXIT_FAILURE;
glEnable(GL_LINE_SMOOTH);
glHint(GL_LINE_SMOOTH_HINT, GL_NICEST);
glLineWidth(2.0f);
while (!glfwWindowShouldClose(window))
{
render();
glfwPollEvents();
}
glfwDestroyWindow(window);
glfwTerminate();
return EXIT_SUCCESS;
}
```
该示例代码使用 GLFW 和 GLEW 库进行窗口管理和 OpenGL 渲染,绘制了一个红色的圆形线条,表示天线的水平方向图。在函数 `drawPolarLine` 中,我们将极坐标 `(r, theta)` 转换为直角坐标 `(x, y)`,然后调用 `glVertex2f` 函数绘制线条。在主循环中,我们不断调用 `render` 函数进行渲染,直到用户关闭窗口。
请注意,这只是一个简单的示例,实际的天线模拟需要更复杂的计算和绘制过程。
相关推荐
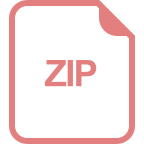
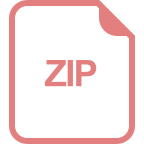
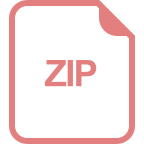














