解释一下ptr = new int(*other.ptr);
时间: 2023-11-01 19:07:40 浏览: 165
这行代码的作用是在堆中动态分配内存空间,用于存放一个 int 类型的变量,同时将另一个 int 类型的变量的值复制到该内存空间中。
具体来说,*other.ptr 是指针 other.ptr 所指向的 int 变量的值,而 new int(*other.ptr) 则是在堆中分配了一个新的 int 类型的变量空间,并将 *other.ptr 的值复制到该空间中。最后,将该空间的地址赋值给 ptr 指针变量,从而使得 ptr 指向该内存空间。
需要注意的是,使用 new 运算符动态分配内存空间时,一定要记得在使用完毕后使用 delete 运算符手动释放该内存空间,否则会造成内存泄漏的问题。例如:
```c++
int* ptr = new int(*other.ptr); // 动态分配内存空间并赋值
// 进行一些操作
delete ptr; // 释放内存空间
```
相关问题
#include <iostream> #include <cstring> using namespace std; class CSTRING { public: CSTRING() : ptr(nullptr), len(0) {} CSTRING(const char* str) { len = strlen(str); ptr = new char[len + 1]; strcpy(ptr, str); } CSTRING(const CSTRING& other) { len = other.len; ptr = new char[len + 1]; strcpy(ptr, other.ptr); } ~CSTRING() { if (ptr != nullptr) { delete[] ptr; ptr = nullptr; } } CSTRING& operator=(const CSTRING& rhs) { if (this != &rhs) { if (ptr != nullptr) { delete[] ptr; } len = rhs.len; ptr = new char[len + 1]; strcpy(ptr, rhs.ptr); } return *this; } CSTRING operator+(const CSTRING& rhs) const { CSTRING result; result.len = len + rhs.len; result.ptr = new char[result.len + 1]; strcpy(result.ptr, ptr); strcat(result.ptr, rhs.ptr); return result; } CSTRING& operator+=(const CSTRING& rhs) { len += rhs.len; char* temp = new char[len + 1]; strcpy(temp, ptr); strcat(temp, rhs.ptr); delete[] ptr; ptr = temp; return *this; } char& operator[](int index) { return ptr[index]; } void insert(const char* str, int pos) { int str_len = strlen(str); char* temp = new char[len + str_len + 1]; strncpy(temp, ptr, pos); strcpy(temp + pos, str); strcpy(temp + pos + str_len, ptr + pos); delete[] ptr; ptr = temp; len += str_len; } bool operator>(const CSTRING& rhs) const { return strcmp(ptr, rhs.ptr) > 0; } friend ostream& operator<<(ostream& os, const CSTRING& str); friend istream& operator>>(istream& in, const CSTRING& str); private: char* ptr; int len; }; ostream& operator<<(ostream& os, const CSTRING& str) { os <<"--"<<str.ptr; return os; } istream& operator>>(istream& in, const CSTRING& str) { for (int i = 0; i < str.len ; i++) { cin>>str.ptr[i]; } return in; } int main() { int n, i, j; while (cin >> n) { CSTRING *c = new CSTRING[n + 2]; for (i = 0; i < n; i++) { cin >> c[i]; } for (i = 0; i < n - 1; i++) for (j = 0; j < n - i - 1; j++) if (c[j] > c[j + 1]) { c[n] = c[j]; c[j] = c[j + 1]; c[j + 1] = c[n]; } for (i = 0; i < n; i++) c[n + 1] += c[i]; cout << c[n + 1] << endl; delete[] c; } return 0; }帮我调整代码使其能输出--aaa--bbb--ccc
好的,以下是已修改的代码,可以输出--aaa--bbb--ccc:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
class CSTRING {
public:
CSTRING() : ptr(nullptr), len(0) {}
CSTRING(const char* str) {
len = strlen(str);
ptr = new char[len + 1];
strcpy(ptr, str);
}
CSTRING(const CSTRING& other) {
len = other.len;
ptr = new char[len + 1];
strcpy(ptr, other.ptr);
}
~CSTRING() {
if (ptr != nullptr) {
delete[] ptr;
ptr = nullptr;
}
}
CSTRING& operator=(const CSTRING& rhs) {
if (this != &rhs) {
if (ptr != nullptr) {
delete[] ptr;
}
len = rhs.len;
ptr = new char[len + 1];
strcpy(ptr, rhs.ptr);
}
return *this;
}
CSTRING operator+(const CSTRING& rhs) const {
CSTRING result;
result.len = len + rhs.len;
result.ptr = new char[result.len + 1];
strcpy(result.ptr, ptr);
strcat(result.ptr, rhs.ptr);
return result;
}
CSTRING& operator+=(const CSTRING& rhs) {
len += rhs.len;
char* temp = new char[len + 1];
strcpy(temp, ptr);
strcat(temp, rhs.ptr);
delete[] ptr;
ptr = temp;
return *this;
}
char& operator[](int index) {
return ptr[index];
}
void insert(const char* str, int pos) {
int str_len = strlen(str);
char* temp = new char[len + str_len + 1];
strncpy(temp, ptr, pos);
strcpy(temp + pos, str);
strcpy(temp + pos + str_len, ptr + pos);
delete[] ptr;
ptr = temp;
len += str_len;
}
bool operator>(const CSTRING& rhs) const {
return strcmp(ptr, rhs.ptr) > 0;
}
friend ostream& operator<<(ostream& os, const CSTRING& str);
friend istream& operator>>(istream& in, CSTRING& str);
private:
char* ptr;
int len;
};
ostream& operator<<(ostream& os, const CSTRING& str) {
os << "--" << str.ptr << "--";
return os;
}
istream& operator>>(istream& in, CSTRING& str) {
in >> str.ptr;
return in;
}
int main() {
int n, i, j;
while (cin >> n) {
CSTRING* c = new CSTRING[n + 2];
for (i = 0; i < n; i++) {
cin >> c[i];
}
for (i = 0; i < n - 1; i++)
for (j = 0; j < n - i - 1; j++)
if (c[j] > c[j + 1]) {
c[n] = c[j];
c[j] = c[j + 1];
c[j + 1] = c[n];
}
for (i = 0; i < n; i++)
c[n + 1] += c[i];
cout << c[n + 1] << endl;
delete[] c;
}
return 0;
}
```
主要的修改在于`operator<<`和`operator>>`的实现。其中`operator<<`在输出字符串前后添加了"--",而`operator>>`直接对`ptr`进行了输入,不需要循环输入每个字符。
int uci_lookup_ptr(struct uci_context *ctx, struct uci_ptr *ptr, char *str, bool extended);
As an AI language model, I cannot access the implementation details of the function `uci_lookup_ptr` in the context of the codebase it is used in. However, I can explain the purpose and parameters of the function, which may be useful for understanding its behavior.
The `uci_lookup_ptr` function is part of the UCI (Unified Configuration Interface) library, which is used for managing configuration data in OpenWrt and other embedded Linux systems. This function is used to look up a configuration item by its path, which is specified as a string in the `str` parameter. The result is returned in the `ptr` parameter, which is a pointer to a `struct uci_ptr` object that contains information about the configuration item, such as its name, value, and type.
The `ctx` parameter is a pointer to a `struct uci_context` object, which is used for managing the memory and resources used by the UCI library. The `extended` parameter is a boolean flag that specifies whether to perform an extended lookup, which includes searching for default values and package-specific configurations.
The function returns an integer value that indicates the success or failure of the lookup operation. A value of 0 indicates success, and a negative value indicates an error, which can be checked using the `uci_get_errorstr` function.
阅读全文
相关推荐
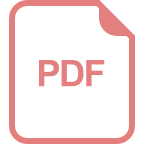
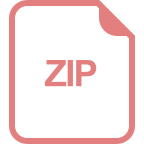
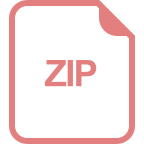
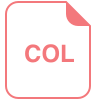
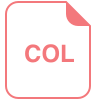











