# 绘制矩形框 def draw_bounding_box(pcd, label): corners = get_box_corners(label) lines = [[0, 1], [1, 2], [2, 3], [3, 0], [4, 5], [5, 6], [6, 7], [7, 4], [0, 4], [1, 5], [2, 6], [3, 7]] colors = [[0, 0, 0] for i in range(len(lines))] line_set = o3d.geometry.LineSet(points=o3d.utility.Vector3dVector(corners), lines=o3d.utility.Vector2iVector(lines)) line_set.colors = o3d.utility.Vector3dVector(colors) pcd += line_set return pcd # 获取矩形框的8个顶点坐标 def get_box_corners(label): h, w, l = label['dimensions'] x, y, z = label['location'] ry = label['rotation_y'] # 3D bounding box corners corners = np.array([ [-l / 2, -h, -w / 2], [l / 2, -h, -w / 2], [l / 2, -h, w / 2], [-l / 2, -h, w / 2], [-l / 2, 0, -w / 2], [l / 2, 0, -w / 2], [l / 2, 0, w / 2], [-l / 2, 0, w / 2] ]) # rotation rot_mat = np.array([ [np.cos(ry), 0, np.sin(ry)], [0, 1, 0], [-np.sin(ry), 0, np.cos(ry)] ]) corners = corners @ rot_mat.T # translation corners += np.array([x, y, z]) return corners将corners中的点把lines中点的信息替换掉
时间: 2023-06-25 21:06:42 浏览: 155
可以尝试使用numpy的函数来替换lines中点的信息。具体做法是,将corners中的点按照lines中的索引顺序进行排列,然后使用numpy的take函数将排列后的点坐标替换掉lines中的索引信息。
修改后的代码如下:
```
import numpy as np
def draw_bounding_box(pcd, label):
corners = get_box_corners(label)
lines = [[0, 1], [1, 2], [2, 3], [3, 0], [4, 5], [5, 6], [6, 7], [7, 4], [0, 4], [1, 5], [2, 6], [3, 7]]
colors = [[0, 0, 0] for i in range(len(lines))]
# 替换lines中的点信息
lines = np.take(corners, lines, axis=0)
line_set = o3d.geometry.LineSet(points=o3d.utility.Vector3dVector(corners), lines=o3d.utility.Vector2iVector(lines))
line_set.colors = o3d.utility.Vector3dVector(colors)
pcd += line_set
return pcd
def get_box_corners(label):
h, w, l = label['dimensions']
x, y, z = label['location']
ry = label['rotation_y']
# 3D bounding box corners
corners = np.array([
[-l / 2, -h, -w / 2],
[l / 2, -h, -w / 2],
[l / 2, -h, w / 2],
[-l / 2, -h, w / 2],
[-l / 2, 0, -w / 2],
[l / 2, 0, -w / 2],
[l / 2, 0, w / 2],
[-l / 2, 0, w / 2]
])
# rotation
rot_mat = np.array([
[np.cos(ry), 0, np.sin(ry)],
[0, 1, 0],
[-np.sin(ry), 0, np.cos(ry)]
])
corners = corners @ rot_mat.T
# translation
corners += np.array([x, y, z])
return corners
```
阅读全文
相关推荐
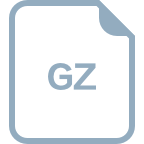

















