def get_box_corners(label): h, w, l = label['dimensions'] x, y, z = label['location'] ry = label['rotation_y'] # 3D bounding box corners corners = np.array([ [-l / 2, -h, -w / 2], [l / 2, -h, -w / 2], [l / 2, -h, w / 2], [-l / 2, -h, w / 2], [-l / 2, 0, -w / 2], [l / 2, 0, -w / 2], [l / 2, 0, w / 2], [-l / 2, 0, w / 2] ]) # rotation rot_mat = np.array([ [np.cos(ry), 0, np.sin(ry)], [0, 1, 0], [-np.sin(ry), 0, np.cos(ry)] ]) corners = corners @ rot_mat.T # translation corners += np.array([x, y, z]) return corners
时间: 2023-06-25 11:07:18 浏览: 142
这段代码是用来获取物体的 3D 边界框(bounding box)的八个顶点坐标。输入参数 label 是包含物体尺寸、位置和旋转角度等信息的字典。函数首先计算出物体的尺寸和位置信息,然后定义八个顶点坐标,接着通过旋转矩阵将顶点坐标旋转到正确的方向,最后加上平移向量,得到最终的顶点坐标。返回的 corners 是一个 8x3 的二维数组,其中每一行表示一个顶点的 x、y、z 坐标。
相关问题
# 绘制矩形框 def draw_bounding_box(pcd, label): corners = get_box_corners(label) lines = [[0, 1], [1, 2], [2, 3], [3, 0], [4, 5], [5, 6], [6, 7], [7, 4], [0, 4], [1, 5], [2, 6], [3, 7]] colors = [[0, 0, 0] for i in range(len(lines))] line_set = o3d.geometry.LineSet(points=o3d.utility.Vector3dVector(corners), lines=o3d.utility.Vector2iVector(lines)) line_set.colors = o3d.utility.Vector3dVector(colors) pcd += line_set return pcd # 获取矩形框的8个顶点坐标 def get_box_corners(label): h, w, l = label['dimensions'] x, y, z = label['location'] ry = label['rotation_y'] # 3D bounding box corners corners = np.array([ [-l / 2, -h, -w / 2], [l / 2, -h, -w / 2], [l / 2, -h, w / 2], [-l / 2, -h, w / 2], [-l / 2, 0, -w / 2], [l / 2, 0, -w / 2], [l / 2, 0, w / 2], [-l / 2, 0, w / 2] ]) # rotation rot_mat = np.array([ [np.cos(ry), 0, np.sin(ry)], [0, 1, 0], [-np.sin(ry), 0, np.cos(ry)] ]) corners = corners @ rot_mat.T # translation corners += np.array([x, y, z]) return corners # 主函数 if __name__ == '__main__': pcd_file = '/home/lang/Documents/orr-small/lidar_4d/1547120787645464.bin' label_file = '/home/lang/Documents/orr-small/label_3d/1547120787645464.txt' pcd = read_bin(pcd_file) labels = read_label(label_file) for label in labels: if label['type'] == 'car': pcd = draw_bounding_box(pcd, label) o3d.visualization.draw_geometries([pcd])得到的图像没有矩形框
可能是因为你的代码存在一些问题,可以逐步排查。首先,检查读取的点云数据和标签文件是否正确,可以打印出来进行检查。其次,检查获取矩形框顶点坐标的函数是否正确,可以单独调用该函数并打印输出结果进行检查。最后,检查绘制矩形框的函数是否正确,可以在绘制完矩形框后打印输出点云数据进行检查。如果还存在问题,可以将代码和数据文件一起发给我,我可以帮你进行调试。
def draw_bounding_box(pcd, label): corners = get_box_corners(label) lines = [[0, 1], [1, 2], [2, 3], [3, 0], [4, 5], [5, 6], [6, 7], [7, 4], [0, 4], [1, 5], [2, 6], [3, 7]]
这段代码的作用是在点云数据中绘制一个包围盒(bounding box)来表示目标物体的位置和大小。其中,pcd是点云数据,label是目标物体的标签信息。函数中调用了get_box_corners函数来获取包围盒的8个顶点坐标,然后通过lines数组来定义包围盒的12条边线段,最后可以使用绘图库如Matplotlib或Open3D来将这些线段绘制出来。
阅读全文
相关推荐
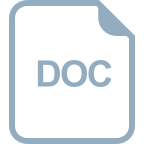
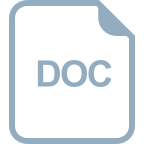
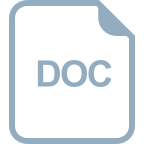












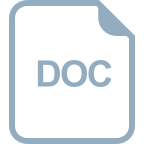