编写程序,对两个一元多项式求和,并输出“和多项式”。
时间: 2023-05-24 14:00:43 浏览: 118
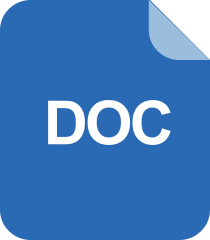
求两个多项式的和

非常感谢您的提问,以下是对两个一元多项式求和的程序:
```
#include <stdio.h>
#include <stdlib.h>
#define MAX_DEGREE 100 // 多项式最大次数
struct polynomial {
int degree; // 多项式次数
float coeff[MAX_DEGREE+1]; // 多项式系数
};
struct polynomial add(struct polynomial p1, struct polynomial p2);
int main() {
struct polynomial p1, p2, result;
int i;
printf("Please input the degree of polynomial 1 (less than %d):\n", MAX_DEGREE);
scanf("%d", &p1.degree);
printf("Please input the coefficients of polynomial 1 (in descending order of degree):\n");
for (i = 0; i <= p1.degree; i++) {
scanf("%f", &p1.coeff[i]);
}
printf("Please input the degree of polynomial 2 (less than %d):\n", MAX_DEGREE);
scanf("%d", &p2.degree);
printf("Please input the coefficients of polynomial 2 (in descending order of degree):\n");
for (i = 0; i <= p2.degree; i++) {
scanf("%f", &p2.coeff[i]);
}
result = add(p1, p2);
printf("The sum polynomial is:\n");
printf("%.2fx^%d", result.coeff[result.degree], result.degree);
for (i = result.degree-1; i >= 0; i--) {
if (result.coeff[i] > 0) {
printf(" + %.2fx^%d", result.coeff[i], i);
} else if (result.coeff[i] < 0) {
printf(" - %.2fx^%d", -result.coeff[i], i);
}
}
printf("\n");
return 0;
}
struct polynomial add(struct polynomial p1, struct polynomial p2) {
struct polynomial result;
int i;
if (p1.degree >= p2.degree) {
result.degree = p1.degree;
} else {
result.degree = p2.degree;
}
for (i = 0; i <= result.degree; i++) {
result.coeff[i] = 0;
if (i <= p1.degree) {
result.coeff[i] += p1.coeff[i];
}
if (i <= p2.degree) {
result.coeff[i] += p2.coeff[i];
}
}
return result;
}
```
该程序会先让用户分别输入两个多项式的次数和系数,并对它们求和,最后输出求和后的多项式。程序中使用了一个结构体 `polynomial` 来表示多项式,同时定义了一个求和函数 `add` 。
阅读全文
相关推荐

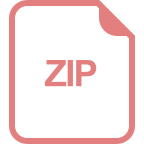
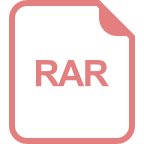
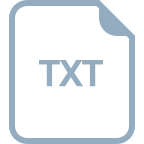
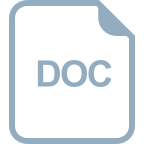








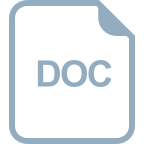
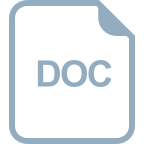
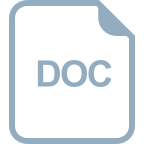