js如何对两个json对象进行深度合并和替换
时间: 2024-03-03 11:52:08 浏览: 176
在JavaScript中,可以使用递归实现两个JSON对象的深度合并和替换。具体步骤如下:
1. 遍历第一个JSON对象的所有属性,如果属性在第二个JSON对象中不存在,则将该属性添加到第二个JSON对象中。
2. 如果属性在第二个JSON对象中也存在,则需要进行合并或替换操作:
a. 如果属性是基本数据类型,直接替换第二个JSON对象中对应属性的值;
b. 如果属性是数组类型,则将第二个JSON对象中对应属性的数组与第一个JSON对象中对应属性的数组进行合并或替换操作,合并或替换后的数组替换第二个JSON对象中对应属性的值;
c. 如果属性是对象类型,则需要递归调用深度合并函数,将第一个JSON对象中对应属性的值与第二个JSON对象中对应属性的值进行合并或替换操作,合并或替换后的对象替换第二个JSON对象中对应属性的值。
3. 最后返回合并或替换后的第二个JSON对象。
以下是一个示例代码,实现两个JSON对象的深度合并和替换:
```javascript
function mergeJSON(json1, json2) {
for (let key in json1) {
if (json1.hasOwnProperty(key)) {
if (json2.hasOwnProperty(key)) {
if (typeof json1[key] === 'object' && typeof json2[key] === 'object') {
json2[key] = mergeJSON(json1[key], json2[key]);
} else {
json2[key] = json1[key];
}
} else {
json2[key] = json1[key];
}
}
}
return json2;
}
```
可以通过以下方式调用该函数:
```javascript
let json1 = {"name": "John", "age": 30, "hobbies": ["reading", "swimming"], "address": {"city": "Beijing", "country": "China"}};
let json2 = {"name": "Peter", "age": 25, "hobbies": ["singing", "dancing"], "address": {"city": "Shanghai"}};
let mergedJSON = mergeJSON(json1, json2);
console.log(JSON.stringify(mergedJSON, null, 2));
```
输出结果如下:
```json
{
"name": "Peter",
"age": 25,
"hobbies": [
"singing",
"dancing",
"reading",
"swimming"
],
"address": {
"city": "Shanghai",
"country": "China"
}
}
```
在上面的示例中,我们对第二个JSON对象进行了深度合并和替换操作,将第一个JSON对象的属性合并或替换到第二个JSON对象中,最终得到了合并或替换后的第二个JSON对象。
阅读全文
相关推荐
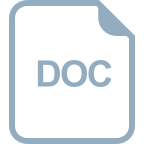
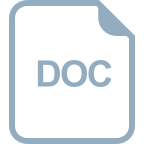
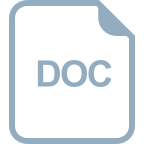
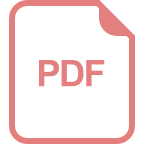
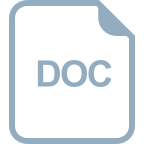
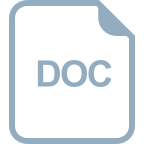
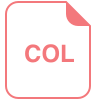
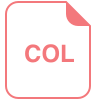
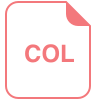
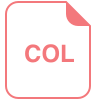
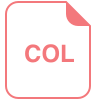
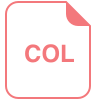
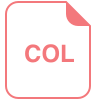
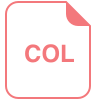
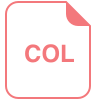
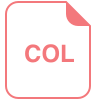
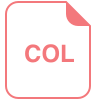
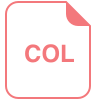
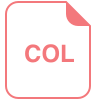