在同一图形窗口绘制sinx和cosx的曲线,要求用不同的颜色、线型、数据点标记字符,x轴的取值范围是[0,2π],y轴的取值范围是[-2,2],并加注解说明‘sinx’和‘cosx’加以区分。
时间: 2024-02-16 11:04:26 浏览: 63
这是一个比较简单的数据可视化问题,可以使用Python的matplotlib库来实现。以下是一个可能的实现示例,供参考:
``` python
import numpy as np
import matplotlib.pyplot as plt
# 生成x轴的数据,取值范围是[0, 2π],共100个点
x = np.linspace(0, 2*np.pi, 100)
# 计算sin(x)和cos(x)的函数值
y1 = np.sin(x)
y2 = np.cos(x)
# 绘制sin(x)的曲线,使用红色实线和圆形数据点标记
plt.plot(x, y1, 'ro-', label='sin(x)')
# 绘制cos(x)的曲线,使用蓝色虚线和三角形数据点标记
plt.plot(x, y2, 'bs--', label='cos(x)')
# 设置x轴和y轴的取值范围
plt.xlim([0, 2*np.pi])
plt.ylim([-2, 2])
# 添加x轴和y轴的标签
plt.xlabel('x')
plt.ylabel('y')
# 添加图例
plt.legend()
# 显示图形
plt.show()
```
这段代码会生成一个图形窗口,其中包含sin(x)和cos(x)的曲线,使用不同的颜色、线型和数据点标记来区分。x轴的取值范围是[0,2π],y轴的取值范围是[-2,2],并添加了注解来说明曲线的含义。
相关问题
在同一坐标系上绘制sinx与cosx的matlab代码
### 回答1:
以下是绘制sinx与cosx的matlab代码:
x = linspace(, 2*pi, 100);
y1 = sin(x);
y2 = cos(x);
plot(x, y1, 'r', x, y2, 'b');
xlabel('x');
ylabel('y');
legend('sin(x)', 'cos(x)');
### 回答2:
在MATLAB中,可以使用以下代码来绘制sinx与cosx的图形,并将它们绘制在同一坐标系上:
```matlab
% 定义x的范围
x = 0:0.01:2*pi;
% 计算sinx和cosx的值
y1 = sin(x);
y2 = cos(x);
% 创建一个图形窗口
figure;
% 绘制sinx的曲线
subplot(2,1,1);
plot(x, y1, 'r'); % 使用红色绘制sinx
xlabel('x');
ylabel('sin(x)');
title('sin(x)');
% 绘制cosx的曲线
subplot(2,1,2);
plot(x, y2, 'b'); % 使用蓝色绘制cosx
xlabel('x');
ylabel('cos(x)');
title('cos(x)');
% 在同一坐标系上绘制
figure;
plot(x, y1, 'r', x, y2, 'b');
xlabel('x');
ylabel('y');
title('sin(x) and cos(x)');
legend('sin(x)', 'cos(x)');
```
上述代码中,首先定义了x的范围,即0到2π之间以0.01为间隔的一组数值。然后利用这些数值计算出sinx和cosx的值。接下来,创建一个图形窗口,并使用subplot函数创建两个子图来分别绘制sinx和cosx的曲线。subplot(2,1,1)指定第一个子图,subplot(2,1,2)指定第二个子图。在每个子图中,使用plot函数绘制sinx和cosx的曲线,同时设置不同的颜色来区分它们。最后,使用plot函数在同一个坐标系上绘制sinx和cosx的曲线,并设置合适的图例以显示每条曲线的含义。
### 回答3:
在Matlab中,我们可以使用plot函数来绘制sinx和cosx的曲线。
首先,我们需要定义x轴的范围。假设我们希望在[-2π, 2π]的范围内绘制,可以使用linspace函数生成一个包含足够多点的数组。代码如下:
``` matlab
x = linspace(-2*pi, 2*pi, 100);
```
接下来,我们可以计算sinx和cosx的值。在Matlab中,可以直接使用sin和cos函数进行计算,这两个函数默认接受弧度作为参数。代码如下:
``` matlab
y_sin = sin(x);
y_cos = cos(x);
```
最后,我们可以使用plot函数将两个函数的曲线绘制在同一坐标系上。可以使用hold on命令来保持坐标系不变,以便在同一图上绘制多个曲线。同时,我们可以通过设置线条的样式、颜色等参数来美化图形。代码如下:
``` matlab
plot(x, y_sin, 'r-', 'LineWidth', 2); % 计算并绘制sinx的曲线
hold on; % 保持坐标系不变
plot(x, y_cos, 'b--', 'LineWidth', 2); % 计算并绘制cosx的曲线
hold off; % 还原坐标系
```
最后,我们可以通过添加标题、坐标轴标签等来完善图形。代码如下:
``` matlab
title('Sin(x) and Cos(x) Curve'); % 添加标题
xlabel('x'); % 添加x轴标签
ylabel('y'); % 添加y轴标签
legend('Sin(x)', 'Cos(x)'); % 添加图例
```
综合起来,完整的Matlab代码如下:
``` matlab
x = linspace(-2*pi, 2*pi, 100);
y_sin = sin(x);
y_cos = cos(x);
plot(x, y_sin, 'r-', 'LineWidth', 2); % 计算并绘制sinx的曲线
hold on; % 保持坐标系不变
plot(x, y_cos, 'b--', 'LineWidth', 2); % 计算并绘制cosx的曲线
hold off; % 还原坐标系
title('Sin(x) and Cos(x) Curve'); % 添加标题
xlabel('x'); % 添加x轴标签
ylabel('y'); % 添加y轴标签
legend('Sin(x)', 'Cos(x)'); % 添加图例
```
以上代码运行后,将在同一坐标系上绘制sinx和cosx的曲线。
编写matlab代码,示出sinx和cosx的函数图形。 要求:在同一个图形窗口中绘制两条曲线,正弦曲线用实线表示,余弦曲线用虚线表示,并用不同的颜色表示,用图例表示。使用网格。将X的范围设置为[-2pi,2pi]。
好的,以下是Matlab代码:
```matlab
x = linspace(-2*pi, 2*pi); % 生成X的取值范围
y1 = sin(x); % 计算sinx的值
y2 = cos(x); % 计算cosx的值
% 绘制图形
plot(x, y1, 'b-', x, y2, 'r--'); % 蓝色实线表示sinx,红色虚线表示cosx
xlabel('X'); % X轴标签
ylabel('Y'); % Y轴标签
title('sinx and cosx'); % 图形标题
grid on; % 显示网格
legend('sinx', 'cosx'); % 图例
```
运行以上代码,即可得到两条曲线在同一张图上的效果图。
相关推荐
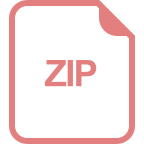












