for start_city, end_cities in around.items(): if start_city in grouped.groups: if start_city not in add_cities: orders_start = grouped.get_group(start_city) add_cities.append(start_city) if end_cities: for end_city in end_cities: if end_city in grouped.groups and end_city not in add_cities: orders_end = grouped.get_group(end_city) add_cities.append(end_city) orders_around = pd.concat([orders_start, orders_end]) result = pd.concat([result, orders_around]) else: if end_cities: for end_city in end_cities: if end_city in grouped.groups and end_city not in add_cities: orders_end = grouped.get_group(end_city) add_cities.append(end_city) result = pd.concat([result, orders_end])这段代码导致result里有重复数据,错误在哪
时间: 2024-02-10 07:08:26 浏览: 36
这段代码中,可能会导致result里有重复数据的原因是在两个地点之间存在多个订单,这些订单被合并到一个DataFrame中后,可能会存在重复的行。为了避免这种情况,可以在合并DataFrame之前,先将其去重。可以使用pandas.DataFrame.drop_duplicates()方法去重,例如在代码中可以像这样添加.drop_duplicates()方法:
```
orders_around = pd.concat([orders_start, orders_end]).drop_duplicates()
result = pd.concat([result, orders_around]).drop_duplicates()
```
这样可以确保result中不会存在重复的行。
相关问题
for start_city, end_cities in around.items(): if start_city in grouped.groups: if start_city not in add_cities: orders_start = grouped.get_group(start_city) add_cities.append(start_city) if end_cities: for end_city in end_cities: if end_city in grouped.groups and end_city not in add_cities: orders_end = grouped.get_group(end_city) add_cities.append(end_city) orders_around = pd.concat([orders_start, orders_end]) result = pd.concat([result, orders_around]) else: if end_cities: for end_city in end_cities: if end_city in grouped.groups and end_city not in add_cities: orders_end = grouped.get_group(end_city) add_cities.append(end_city) result = pd.concat([result, orders_end])这段代码导致result里有重复数据,错误在哪,改正错误,重写代码
这段代码可能会导致result里有重复数据的原因是对于同一对起始地点和终点地点,可能存在多个订单,这些订单被合并到一个DataFrame中后,可能会存在重复的行。为了避免这种情况,可以在合并DataFrame之前,先将其去重。可以使用pandas.DataFrame.drop_duplicates()方法去重。下面是修改后的代码:
```
result = pd.DataFrame()
add_cities = []
for start_city, end_cities in around.items():
if start_city in grouped.groups:
if start_city not in add_cities:
orders_start = grouped.get_group(start_city)
add_cities.append(start_city)
if end_cities:
for end_city in end_cities:
if end_city in grouped.groups and end_city not in add_cities:
orders_end = grouped.get_group(end_city)
add_cities.append(end_city)
orders_around = pd.concat([orders_start, orders_end]).drop_duplicates()
result = pd.concat([result, orders_around]).drop_duplicates()
else:
result = pd.concat([result, orders_start]).drop_duplicates()
else:
if end_cities:
for end_city in end_cities:
if end_city in grouped.groups and end_city not in add_cities:
orders_end = grouped.get_group(end_city)
add_cities.append(end_city)
result = pd.concat([result, orders_end]).drop_duplicates()
```
在处理orders_around和result时,添加了.drop_duplicates()方法以确保没有重复的行。
for group_name, group_newdata in grouped:
这是一个Python中对数据进行分组的语法,其中grouped是一个已经按照某个条件进行分组的数据集,group_name是每个分组的名称,group_newdata是每个分组的数据。这个语法可以用于对数据进行聚合计算,例如对每个分组进行求和、平均值等统计量的计算。在数据分析和可视化工具Tableau中,也有类似的聚合计算功能,可以对数据进行分组并计算每组的统计量。
相关推荐
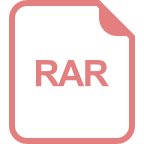
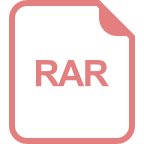














