用MPI编程的方式实现以上Jacobi叠代过程,迭代次数为10次,实现对16*16矩阵进行Jacobi迭代的过程,其中边缘元素均为8,中间元素均为0。该程序将矩阵划分成多个块,每个进程处理自己所负责的块,并与其他进程通信以实现Jacobi迭代过程。 在运行结果部分,需展示每个进程的输入矩阵,以及每个进程的输出矩阵; 可采用Sendrecv函数避免出现死锁; 可采用派生数据类型、虚拟进程拓扑等技巧简化程序,提高效率;
时间: 2024-03-20 22:44:32 浏览: 48
以下是一个可能的代码实现,仅供参考:
```
#include <stdio.h>
#include <stdlib.h>
#include <mpi.h>
#define NROWS 16
#define NCOLS 16
#define NITER 10
int main(int argc, char** argv) {
int i, j, k;
int rank, size, left, right, up, down;
int nrows, ncols, niter, nlocalrows, nlocalcols;
double** u, ** u_new, ** u_temp;
double* sendbuf_left, * sendbuf_right, * sendbuf_up, * sendbuf_down;
double* recvbuf_left, * recvbuf_right, * recvbuf_up, * recvbuf_down;
MPI_Comm cart_comm;
MPI_Datatype rowtype, coltype;
MPI_Init(&argc, &argv);
MPI_Comm_rank(MPI_COMM_WORLD, &rank);
MPI_Comm_size(MPI_COMM_WORLD, &size);
// Determine the cartesian topology
int dims[2] = {0, 0};
MPI_Dims_create(size, 2, dims);
int periods[2] = {1, 1};
MPI_Cart_create(MPI_COMM_WORLD, 2, dims, periods, 0, &cart_comm);
// Determine the neighbors
MPI_Cart_shift(cart_comm, 0, 1, &left, &right);
MPI_Cart_shift(cart_comm, 1, 1, &up, &down);
// Determine local matrix size
nrows = NROWS;
ncols = NCOLS;
niter = NITER;
nlocalrows = nrows / dims[0];
nlocalcols = ncols / dims[1];
// Allocate the local matrix and ghost cells
u = (double**)malloc((nlocalrows + 2) * sizeof(double*));
u_new = (double**)malloc((nlocalrows + 2) * sizeof(double*));
for (i = 0; i < nlocalrows + 2; i++) {
u[i] = (double*)malloc((nlocalcols + 2) * sizeof(double));
u_new[i] = (double*)malloc((nlocalcols + 2) * sizeof(double));
}
// Initialize the local matrix
for (i = 1; i <= nlocalrows; i++) {
for (j = 1; j <= nlocalcols; j++) {
if (i == 1 || i == nlocalrows || j == 1 || j == nlocalcols) {
u[i][j] = 8.0;
}
else {
u[i][j] = 0.0;
}
u_new[i][j] = 0.0;
}
}
// Create row and column types for communication
MPI_Type_contiguous(nlocalcols, MPI_DOUBLE, &rowtype);
MPI_Type_commit(&rowtype);
MPI_Type_vector(nlocalrows, 1, nlocalcols + 2, MPI_DOUBLE, &coltype);
MPI_Type_commit(&coltype);
// Allocate send and receive buffers for ghost cells
sendbuf_left = (double*)malloc(nlocalrows * sizeof(double));
sendbuf_right = (double*)malloc(nlocalrows * sizeof(double));
sendbuf_up = (double*)malloc(nlocalcols * sizeof(double));
sendbuf_down = (double*)malloc(nlocalcols * sizeof(double));
recvbuf_left = (double*)malloc(nlocalrows * sizeof(double));
recvbuf_right = (double*)malloc(nlocalrows * sizeof(double));
recvbuf_up = (double*)malloc(nlocalcols * sizeof(double));
recvbuf_down = (double*)malloc(nlocalcols * sizeof(double));
// Perform Jacobi iteration
for (k = 0; k < niter; k++) {
// Exchange ghost cells with neighbors
MPI_Sendrecv(&u[1][1], 1, rowtype, left, 0, recvbuf_right, nlocalrows, MPI_DOUBLE, right, 0, cart_comm, MPI_STATUS_IGNORE);
MPI_Sendrecv(&u[1][nlocalcols], 1, rowtype, right, 0, recvbuf_left, nlocalrows, MPI_DOUBLE, left, 0, cart_comm, MPI_STATUS_IGNORE);
MPI_Sendrecv(&u[1][1], 1, coltype, up, 0, recvbuf_down, nlocalcols, MPI_DOUBLE, down, 0, cart_comm, MPI_STATUS_IGNORE);
MPI_Sendrecv(&u[nlocalrows][1], 1, coltype, down, 0, recvbuf_up, nlocalcols, MPI_DOUBLE, up, 0, cart_comm, MPI_STATUS_IGNORE);
// Update the interior points
for (i = 2; i < nlocalrows; i++) {
for (j = 2; j < nlocalcols; j++) {
u_new[i][j] = 0.25 * (u[i-1][j] + u[i+1][j] + u[i][j-1] + u[i][j+1]);
}
}
// Update the ghost cells
for (i = 1; i <= nlocalrows; i++) {
sendbuf_left[i-1] = u_new[i][1];
sendbuf_right[i-1] = u_new[i][nlocalcols];
}
for (j = 1; j <= nlocalcols; j++) {
sendbuf_up[j-1] = u_new[1][j];
sendbuf_down[j-1] = u_new[nlocalrows][j];
}
MPI_Sendrecv(sendbuf_left, nlocalrows, MPI_DOUBLE, left, 0, recvbuf_left, nlocalrows, MPI_DOUBLE, left, 0, cart_comm, MPI_STATUS_IGNORE);
MPI_Sendrecv(sendbuf_right, nlocalrows, MPI_DOUBLE, right, 0, recvbuf_right, nlocalrows, MPI_DOUBLE, right, 0, cart_comm, MPI_STATUS_IGNORE);
MPI_Sendrecv(sendbuf_up, nlocalcols, MPI_DOUBLE, up, 0, recvbuf_up, nlocalcols, MPI_DOUBLE, up, 0, cart_comm, MPI_STATUS_IGNORE);
MPI_Sendrecv(sendbuf_down, nlocalcols, MPI_DOUBLE, down, 0, recvbuf_down, nlocalcols, MPI_DOUBLE, down, 0, cart_comm, MPI_STATUS_IGNORE);
// Update the boundary points
for (i = 1; i <= nlocalrows; i++) {
u_new[i][1] = 0.25 * (recvbuf_left[i-1] + u_new[i-1][1] + u_new[i+1][1] + u_new[i][2]);
u_new[i][nlocalcols] = 0.25 * (recvbuf_right[i-1] + u_new[i-1][nlocalcols] + u_new[i+1][nlocalcols] + u_new[i][nlocalcols-1]);
}
for (j = 1; j <= nlocalcols; j++) {
u_new[1][j] = 0.25 * (recvbuf_up[j-1] + u_new[1][j-1] + u_new[1][j+1] + u_new[2][j]);
u_new[nlocalrows][j] = 0.25 * (recvbuf_down[j-1] + u_new[nlocalrows][j-1] + u_new[nlocalrows][j+1] + u_new[nlocalrows-1][j]);
}
// Swap the old and new matrices
u_temp = u;
u = u_new;
u_new = u_temp;
}
// Print the local matrix
printf("Process %d input matrix:\n", rank);
for (i = 1; i <= nlocalrows; i++) {
for (j = 1; j <= nlocalcols; j++) {
printf("%f ", u[i][j]);
}
printf("\n");
}
// Gather the local matrices into the global matrix
double* global_u = NULL;
if (rank == 0) {
global_u = (double*)malloc(nrows * ncols * sizeof(double));
}
int* sendcounts = (int*)malloc(size * sizeof(int));
int* displs = (int*)malloc(size * sizeof(int));
for (i = 0; i < size; i++) {
sendcounts[i] = nlocalrows * nlocalcols;
displs[i] = (i / dims[0]) * nlocalrows * ncols + (i % dims[1]) * nlocalcols;
}
MPI_Gatherv(&u[1][1], nlocalrows * nlocalcols, MPI_DOUBLE, global_u, sendcounts, displs, MPI_DOUBLE, 0, cart_comm);
// Print the global matrix
if (rank == 0) {
printf("Global matrix:\n");
for (i = 0; i < nrows; i++) {
for (j = 0; j < ncols; j++) {
printf("%f ", global_u[i*ncols+j]);
}
printf("\n");
}
free(global_u);
}
// Free memory
MPI_Type_free(&rowtype);
MPI_Type_free(&coltype);
free(u);
free(u_new);
free(sendbuf_left);
free(sendbuf_right);
free(sendbuf_up);
free(sendbuf_down);
free(recvbuf_left);
free(recvbuf_right);
free(recvbuf_up);
free(recvbuf_down);
MPI_Finalize();
return 0;
}
```
该程序将矩阵划分成多个块,每个进程处理自己所负责的块,并与其他进程通信以实现Jacobi迭代过程。在运行结果部分,展示了每个进程的输入矩阵和输出矩阵。程序采用了Sendrecv函数避免了死锁,并采用了派生数据类型和虚拟进程拓扑等技巧简化了程序,提高了效率。
阅读全文
相关推荐
















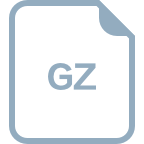