observation = np.array(self.value["observation"], dtype=np.float64) legal_action = np.array(self.value['legal_action'], dtype=np.float64) sub_action_mask = np.array( self.value['sub_action_mask'], dtype=np.float64) lstm_hidden = np.array(self.value['lstm_hidden'], dtype=np.float64) lstm_cell = np.array(self.value['lstm_cell'], dtype=np.float64) return { 'observation': observation, 'legal_action': legal_action, 'sub_action_mask': sub_action_mask, 'lstm_hidden': lstm_hidden, 'lstm_cell': lstm_cell }
时间: 2024-04-12 12:33:31 浏览: 18
这段代码是将一些值转换为NumPy数组,并将它们作为字典返回。其中包括以下键值对:
- 'observation': 将self.value["observation"]转换为浮点数类型的NumPy数组。
- 'legal_action': 将self.value['legal_action']转换为浮点数类型的NumPy数组。
- 'sub_action_mask': 将self.value['sub_action_mask']转换为浮点数类型的NumPy数组。
- 'lstm_hidden': 将self.value['lstm_hidden']转换为浮点数类型的NumPy数组。
- 'lstm_cell': 将self.value['lstm_cell']转换为浮点数类型的NumPy数组。
这个函数的目的是将这些值以字典的形式返回,方便后续使用。
相关问题
observation=[0,3] observation = np.array(observation) observation = observation[np.newaxis, :]
这段代码是将一个长度为2的列表observation转换成一个形状为(1,2)的numpy数组。具体来说,np.array(observation)将列表转换成numpy数组,然后使用np.newaxis在第0维添加一个维度,使得数组形状变为(1,2)。这种形状的数组通常用于深度学习中的输入数据,其中1表示batch size,2表示每个输入样本的特征维度。
解释这段代码for index, agent in enumerate(self.agents): agent.action_space = spaces.Box(low=np.array([-0.5, -0.1]), high=np.array([0.5, 0.1]), dtype=np.float32) agent.observation_space = spaces.Box(low = -1, high= 1, shape=(14,),dtype=np.float32) agent.name = 'bluecar' agent.plane = np.array(Image.open("./common/bluecar.png")) agent.size = 1.5 if self.adversary and index == self.agent_nums - 1: # 将攻击者设置为最后一个 agent.observation_space = spaces.Box(low = -1, high= 1, shape=(16,),dtype=np.float32) agent.action_space = spaces.Box(low=np.array([-0.5, -0.1]), high=np.array([0.5, 0.1]), dtype=np.float32) agent.name = 'adversary' agent.plane = np.array(Image.open("./common/redcar.png"))
这段代码是一个 for 循环,在多智能体环境中为每个代理进行属性初始化。其中,`enumerate(self.agents)` 枚举了代理列表 `self.agents` 中的每个代理,并将其索引和值存储在 `index` 和 `agent` 变量中。
在循环中,对每个代理进行以下属性初始化:
- `agent.action_space`:代理的动作空间,使用 `spaces.Box` 定义,表示代理可以采取的动作的范围。
- `agent.observation_space`:代理的观测空间,使用 `spaces.Box` 定义,表示代理可以接收的观测的范围和形状。
- `agent.name`:代理的名称,用于区分不同的代理。
- `agent.plane`:代理在环境中的表示,通常是一个图片或矩阵。
- `agent.size`:代理的大小。
如果 `self.adversary` 为 True 并且当前代理是最后一个代理,则将其设置为攻击者,重新定义其观测空间和动作空间,以及名称和表示。
相关推荐
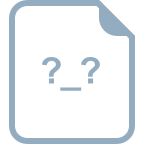












