定义一个三维向量类,并定义相应的特殊方法实现:1)两个该类对象的加、减运算(要求支持运算符+、-);2)实现该类对象与标量的乘、除运算(要求支持运算符*、/,乘法时包括标量在前和后两种情况);3)以及向量的print方法; 向量的长度的定义;向量的点积以及叉积的定义; 定义一个类属性,表征已经实例化的对象的个数。2)创建1000个随机三维向量,要求x轴的范围在0~100,y的范围在-100~0;z的范围在-50~50,并用matplotlib把1000个向量的x坐标、y坐标和z坐标分别用三条2D曲线绘制出来(一个plot),然后给出Axis grid、 xlabel、legend、line线形等config。
时间: 2023-10-27 08:30:57 浏览: 102
好的,我会根据你的要求来回答你的问题。
首先,我们定义一个三维向量类:
```python
class Vector3D:
count = 0
def __init__(self, x=0, y=0, z=0):
self.x = x
self.y = y
self.z = z
Vector3D.count += 1
def __add__(self, other):
return Vector3D(self.x + other.x, self.y + other.y, self.z + other.z)
def __sub__(self, other):
return Vector3D(self.x - other.x, self.y - other.y, self.z - other.z)
def __mul__(self, other):
if isinstance(other, (int, float)):
return Vector3D(self.x * other, self.y * other, self.z * other)
elif isinstance(other, Vector3D):
return self.x * other.x + self.y * other.y + self.z * other.z
def __rmul__(self, other):
return Vector3D(self.x * other, self.y * other, self.z * other)
def __truediv__(self, other):
return Vector3D(self.x / other, self.y / other, self.z / other)
def print(self):
print(f"({self.x}, {self.y}, {self.z})")
def length(self):
return (self.x ** 2 + self.y ** 2 + self.z ** 2) ** 0.5
def dot_product(self, other):
return self.x * other.x + self.y * other.y + self.z * other.z
def cross_product(self, other):
return Vector3D(self.y * other.z - self.z * other.y,
self.z * other.x - self.x * other.z,
self.x * other.y - self.y * other.x)
```
这个类中实现了向量的加、减、乘、除、长度、点积以及叉积的运算,同时还有一个print方法用于打印向量的值。此外,我们还添加了一个类属性count,用于记录已经实例化的对象的个数。
接下来,我们创建1000个随机三维向量,并用matplotlib将它们的x坐标、y坐标和z坐标分别用三条2D曲线绘制出来:
```python
import random
import matplotlib.pyplot as plt
vectors = [Vector3D(random.randint(0, 100), random.randint(-100, 0), random.randint(-50, 50)) for _ in range(1000)]
xs = [v.x for v in vectors]
ys = [v.y for v in vectors]
zs = [v.z for v in vectors]
fig = plt.figure()
ax = fig.add_subplot(111)
ax.plot(xs, label='x')
ax.plot(ys, label='y')
ax.plot(zs, label='z')
ax.set_xlabel('Index')
ax.set_ylabel('Value')
ax.grid(True)
ax.legend()
plt.show()
```
这里我们使用了Python的random模块来生成随机的三维向量。然后,我们分别将这些向量的x、y、z坐标提取出来,存储在xs、ys、zs列表中。最后,我们使用matplotlib的plot函数将这些列表的值绘制成曲线,并对图形进行了一些设置,包括添加Axis grid、xlabel、legend等。
运行上述代码,即可得到1000个随机三维向量的图形:

阅读全文
相关推荐
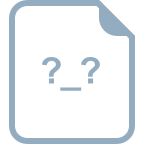
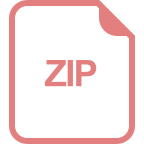













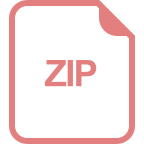
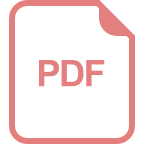