svm hog数字分类 python
时间: 2023-09-28 14:10:20 浏览: 263
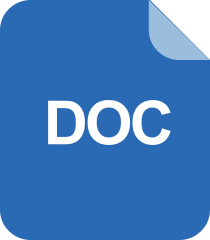
基于 SVM 分类器和 HOG 的模式识别系统的设计与实现代码大全.doc
SVM(Support Vector Machine)是一种常见的分类算法,HOG(Histogram of Oriented Gradients)是一种常用的特征提取方法,可以用于数字分类。
下面是一个使用SVM和HOG进行数字分类的Python实例代码:
```python
import cv2
import numpy as np
from sklearn.svm import SVC
from sklearn.metrics import accuracy_score
from sklearn.model_selection import train_test_split
from skimage.feature import hog
# 加载手写数字数据集
digits = cv2.imread('digits.png', 0)
# 分割手写数字数据集
images = [np.hsplit(row, 100) for row in np.vsplit(digits, 50)]
images = np.array(images, dtype=np.float32)
# 提取HOG特征
hog_features = []
for image in images:
for row in image:
hog_features.append(hog(row, orientations=9, pixels_per_cell=(8, 8), cells_per_block=(2, 2)))
hog_features = np.array(hog_features, dtype=np.float32)
# 创建标签
labels = np.repeat(np.arange(10), 500)
# 划分数据集
X_train, X_test, y_train, y_test = train_test_split(hog_features, labels, test_size=0.2)
# 训练SVM模型
svm_model = SVC(kernel='linear', C=1.0)
svm_model.fit(X_train, y_train)
# 预测
y_pred = svm_model.predict(X_test)
print("Accuracy: {:.2f}%".format(accuracy_score(y_test, y_pred) * 100))
```
需要注意的是,这个实例代码假定手写数字数据集已经准备好,并且保存在名为“digits.png”的文件中。如果没有这个数据集,可以使用`sklearn.datasets.load_digits()`函数加载手写数字数据集。
这个实例代码的步骤如下:
1. 加载手写数字数据集,并分割成1000个数字图像。
2. 对每个数字图像进行HOG特征提取。
3. 创建标签,每个数字有500个样本。
4. 划分数据集为训练集和测试集。
5. 训练SVM模型。
6. 预测测试集的分类结果,并计算准确率。
该实例代码中的准确率可能不是很高,可以通过调整HOG参数、SVM参数、数据预处理等方法来提高分类准确率。
阅读全文
相关推荐
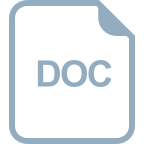
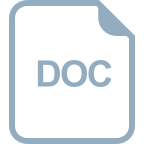
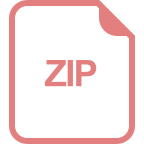
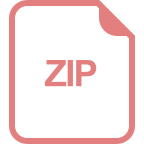
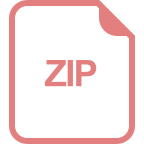
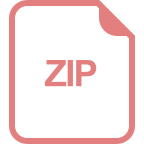
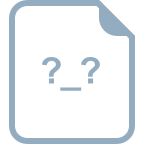
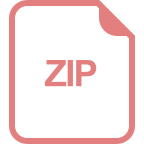









