dinic's 算法c++代码
时间: 2024-09-17 22:03:08 浏览: 8
Dinic's算法(也称为增广路径算法)是用于求解网络流问题的一种经典算法,主要用于计算最大流量和最小割。图论中,这个算法用来找到一个无向加权图中,从源节点到汇点的最大流量。在C++中实现时,通常会用到邻接矩阵或邻接表来表示图。
以下是一个简单的Dinic's算法的基本步骤的C++代码示例:
```cpp
#include <iostream>
#include <vector>
#include <queue>
const int MAX_V = 100; // 图的顶点数
const int INF = INT_MAX; // 无穷大
struct Edge {
int to, cap, flow;
Edge(int to, int cap) : to(to), cap(cap), flow(0) {}
};
class Dinic {
private:
std::vector<Edge> adj[MAX_V];
bool visited[MAX_V]; // 记录每个顶点是否已访问过
int dist[MAX_V]; // 最短距离数组
int parent[MAX_V]; // 前驱节点数组
public:
void addEdge(int u, int v, int capacity) {
adj[u].push_back(Edge(v, capacity));
adj[v].push_back(Edge(u, 0)); // 双向边,方向相反并反向容量
}
int maxFlow(int s, int t) {
fill(visited, visited + MAX_V, false);
fill(dist, dist + MAX_V, INF);
dist[s] = 0;
while (true) {
std::priority_queue<std::pair<int, int>, std::vector<std::pair<int, int>>, std::greater<std::pair<int, int>>> pq;
pq.push({0, s});
while (!pq.empty()) {
int d = pq.top().first;
int u = pq.top().second;
pq.pop();
if (d > dist[u]) continue;
if (u == t) break;
for (auto &edge : adj[u]) {
if (edge.flow < edge.cap && dist[edge.to] > d + 1) {
dist[edge.to] = d + 1;
parent[edge.to] = u;
pq.push({dist[edge.to], edge.to});
}
}
}
if (dist[t] == INF) return 0; // 如果没有更短路径了,返回已经找到的最大流量
int flow = INF;
for (int u = t; u != s; u = parent[u])
flow = std::min(flow, adj[parent[u]][u].cap - adj[u][parent[u]].flow);
for (int u = t; u != s; u = parent[u]) {
adj[parent[u]][u].flow += flow;
adj[u][parent[u]].flow -= flow;
}
}
}
};
```
这段代码定义了一个`Dinic`类,其中包含添加边、计算最大流的方法。注意,这只是一个简化版的实现,实际应用中可能还需要处理更复杂的逻辑,比如循环边和更高效的优先队列实现。
相关推荐
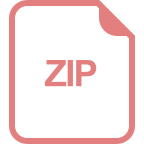
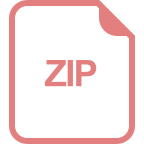









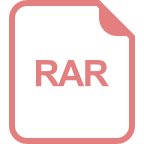
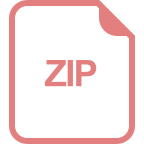
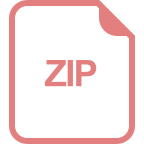
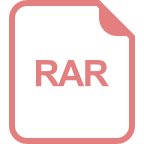
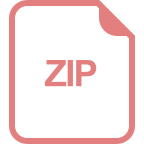