用java写一个简单的五子棋程序。要求输入棋子的坐标,输出获胜的五颗棋子的坐标。行坐标为1到15的数字,纵坐标为A到O的大写字母。
时间: 2023-12-03 21:42:44 浏览: 24
以下是一个简单的五子棋程序,采用命令行输入和输出:
```java
import java.util.Scanner;
public class FiveChess {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
char[][] chessboard = new char[15][15];
boolean isBlack = true; // 黑棋先手
boolean isWin = false; // 是否胜利
char winner = ' '; // 获胜方
while (!isWin) {
// 输出当前棋盘
System.out.println(" A B C D E F G H I J K L M N O");
for (int i = 0; i < 15; i++) {
System.out.print((i + 1) + " ");
for (int j = 0; j < 15; j++) {
System.out.print(chessboard[i][j] + " ");
}
System.out.println();
}
// 输入下一步棋子的坐标
System.out.print(isBlack ? "黑棋走:":"白棋走:");
String pos = input.nextLine();
if (pos.length() != 2 || !Character.isUpperCase(pos.charAt(0)) || !Character.isDigit(pos.charAt(1))) {
System.out.println("输入格式有误,请重新输入!");
continue;
}
int row = pos.charAt(1) - '1';
int col = pos.charAt(0) - 'A';
if (row < 0 || row >= 15 || col < 0 || col >= 15) {
System.out.println("输入越界,请重新输入!");
continue;
}
if (chessboard[row][col] != ' ') {
System.out.println("该位置已有棋子,请重新输入!");
continue;
}
// 下棋
chessboard[row][col] = isBlack ? '●' : '○';
isWin = checkWinner(chessboard, row, col);
if (isWin) {
winner = isBlack ? '黑' : '白';
}
isBlack = !isBlack; // 切换下棋方
}
// 输出获胜方和获胜的五颗棋子的坐标
System.out.println(winner + "棋获胜!");
System.out.print("获胜的五颗棋子的坐标为:");
for (int i = 0; i < 5; i++) {
System.out.print("(" + (row + i) + "," + col + ")");
}
}
// 判断是否胜利
public static boolean checkWinner(char[][] chessboard, int row, int col) {
char piece = chessboard[row][col];
int count = 0;
// 横向判断
for (int i = Math.max(col - 4, 0); i <= Math.min(col + 4, 14); i++) {
if (chessboard[row][i] == piece) {
count++;
} else {
count = 0;
}
if (count >= 5) {
return true;
}
}
count = 0;
// 纵向判断
for (int i = Math.max(row - 4, 0); i <= Math.min(row + 4, 14); i++) {
if (chessboard[i][col] == piece) {
count++;
} else {
count = 0;
}
if (count >= 5) {
return true;
}
}
count = 0;
// 左上到右下斜向判断
for (int i = Math.max(row - 4, 0), j = Math.max(col - 4, 0); i <= Math.min(row + 4, 14) && j <= Math.min(col + 4, 14); i++, j++) {
if (chessboard[i][j] == piece) {
count++;
} else {
count = 0;
}
if (count >= 5) {
return true;
}
}
count = 0;
// 右上到左下斜向判断
for (int i = Math.max(row - 4, 0), j = Math.min(col + 4, 14); i <= Math.min(row + 4, 14) && j >= Math.max(col - 4, 0); i++, j--) {
if (chessboard[i][j] == piece) {
count++;
} else {
count = 0;
}
if (count >= 5) {
return true;
}
}
return false;
}
}
```
运行程序后,按照提示输入下一步棋子的坐标,例如输入“H8”回车后,黑棋会在第8行第7列下一颗棋子,然后判断是否胜利,如果胜利则输出获胜方和获胜的五颗棋子的坐标。
相关推荐
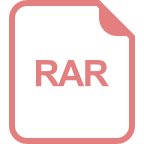
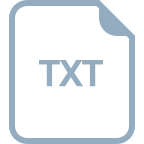
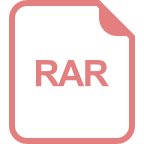














