void print(long n) { if (n==0) return ; else{ print(n/10); printf("%d", n%10); return ; } }n=5378时的结果
时间: 2024-02-02 12:03:29 浏览: 37
当n=5378时,调用print函数后,会首先执行print(n/10),即print(537),然后继续执行print(n/10),即print(53),接着执行print(n/10),即print(5),此时n/10的值已经变成0,于是执行return语句返回上一层递归,即回到n=53时的情况,接着执行printf("%d", n%10),即输出3,然后执行return语句返回上一层递归,即回到n=537时的情况,接着执行printf("%d", n%10),即输出8,最后函数结束。
因此,函数print(5378)的结果是8537。
相关问题
//动态链表 #include "stdio.h" #include "stdlib.h" #define LEN sizeof(struct Student) struct Student{ long num; float score; struct Student *next; }; int n; //全局变量 struct Student*creat(void) //建立链表 { //此函数无需放入形参 struct Student *head ,*p1,*p2; n=0; p1=p2=(struct Student *)malloc(LEN); scanf("%ld%f",&p1->num,&p1->score); while(p1->num!=0) //此处判断遇 0 代表结束 { n=n+1; if(n==1) head=p1; else p2->next=p1; p2=p1; p1=(struct Student *) malloc(LEN); scanf("%ld%f",&p1->num,&p1->score); } p2->next=NULL; return head; //返回值是链表头 } void del(struct Student *head) //删除结点 { struct Student *p1,*p2,*p; int k,a=n; printf("请输入要删除的结点:\n"); scanf("%d",&k); p1=head; while(n==a&&p1->next!=NULL) { if(head->num==k) //删除的是头结点 { p1=head; // 原头结点 p2= p1->next; // 新的头结点 free(p1); // 释放原头结点内存空间 head =p2; // 头指针向后移一位 n-=1; } p2=p1; p1=p1->next; if(p1->num==k) { p=p1->next; p2->next=p; n-=1; } } } void print(struct Student *head)//输出链表 { struct Student *p; p=head; printf("\nNow,These %d reconds are:\n",n); if(head!=NULL) do { printf("%ld %5.1f\n",p->num,p->score); p=p->next; }while(p!=NULL); } int main() { struct Student *pt; pt=creat(); del(pt); print(pt); return 0; }哪里有错误
代码中存在一个潜在的错误。当删除的是最后一个结点时,程序会陷入死循环,无法退出。具体来说,是由于在while循环中,当p1指向最后一个结点时,p1->next为NULL,但是判断条件却是p1->next!=NULL,导致循环无法结束。应该将判断条件改为p1!=NULL,这样当p1指向最后一个结点时,循环可以正常退出。修改后的代码如下:
```
void del(struct Student *head) //删除结点
{
struct Student *p1,*p2,*p;
int k,a=n;
printf("请输入要删除的结点:\n");
scanf("%d",&k);
p1=head;
while(n==a&&p1!=NULL)
{
if(head->num==k) //删除的是头结点
{
p1=head; // 原头结点
p2= p1->next; // 新的头结点
free(p1); // 释放原头结点内存空间
head =p2; // 头指针向后移一位
n-=1;
}
p2=p1;
p1=p1->next;
if(p1!=NULL && p1->num==k)
{
p=p1->next;
p2->next=p;
n-=1;
}
}
}
```
补全下面代码:#include "stdio.h" #include "iostream.h" #include "stdlib.h" #include "time.h" const int size=1000; typedef long RecType; typedef struct { RecType rec[size+1]; int n; } SqTable; //顺序查找 void SeqSearch(SqTable R,RecType k,int &i,int &j) { } void print(SqTable R,int n) { } //排序 void sort(SqTable &R,int n) { } //折半查找 int BinSearch(SqTable R,RecType k,int &j) { } void main() { SqTable R,A; RecType x; int i,j,n; int k; do { printf("\n\n\n\n"); printf("\t\t 查找子系统\n"); printf("\t\t*****************************\n"); printf("\t\t* 1----产生数据 *\n"); printf("\t\t* 2----顺序查找 *\n"); printf("\t\t* 3----二分查找 *\n"); printf("\t\t* 4----打印数据 *\n"); printf("\t\t* 0----返 回 *\n"); printf("\t\t*****************************\n"); printf("\t\t 请选择菜单项(0-4):"); scanf("%d",&k); switch(k) { case 1://随机产生数据 printf("请输入要产生随机数的个数(n<=%d)n= ",size); scanf("%d",&n); srand((unsigned)time( NULL )); for (i=1;i<=n;i++) A.rec[i]=R.rec[i]=rand(); A.n=R.n=n; break; case 2://顺序查找 printf("请输入要查找的关键字:"); scanf("%ld",&x); SeqSearch(R,x,i,j); if (i==0) printf("没有此数据!!!"); else printf("在 %d 个下标,第 %d 次找到。",i,j); break; case 3://二分查找 sort(A,n); print(A,n); printf("请输入要查找的关键字:"); scanf("%ld",&x); j=0; i=BinSearch(A,x,j); if (i==0) printf("没有此数据!!!"); else printf("在 %d 个下标,第 %d 次找到。",i,j); break; case 4: print(A,n); } }while (k!=0); }
这是一个简单的顺序查找和折半查找的程序,补全代码如下:
```c
#include "stdio.h"
#include "iostream.h"
#include "stdlib.h"
#include "time.h"
const int size = 1000;
typedef long RecType;
typedef struct {
RecType rec[size+1];
int n;
} SqTable;
//顺序查找
void SeqSearch(SqTable R, RecType k, int &i, int &j) {
i = 1;
while (i <= R.n && R.rec[i] != k) {
i++;
j++;
}
if (i <= R.n) {
j++;
} else {
i = 0;
}
}
void print(SqTable R, int n) {
for (int i = 1; i <= n; i++) {
printf("%d ", R.rec[i]);
}
printf("\n");
}
//排序
void sort(SqTable &R, int n) {
int i, j;
RecType temp;
for (i = 1; i < n; i++) {
for (j = n; j >= i+1; j--) {
if (R.rec[j] < R.rec[j-1]) {
temp = R.rec[j];
R.rec[j] = R.rec[j-1];
R.rec[j-1] = temp;
}
}
}
}
//折半查找
int BinSearch(SqTable R, RecType k, int &j) {
int low = 1, high = R.n, mid;
while (low <= high) {
mid = (low + high) / 2;
j++;
if (R.rec[mid] == k) {
return mid;
} else if (R.rec[mid] > k) {
high = mid - 1;
} else {
low = mid + 1;
}
}
return 0;
}
void main() {
SqTable R, A;
RecType x;
int i, j, n;
int k;
do {
printf("\n\n\n\n");
printf("\t\t 查找子系统\n");
printf("\t\t*****************************\n");
printf("\t\t* 1----产生数据 *\n");
printf("\t\t* 2----顺序查找 *\n");
printf("\t\t* 3----二分查找 *\n");
printf("\t\t* 4----打印数据 *\n");
printf("\t\t* 0----返 回 *\n");
printf("\t\t*****************************\n");
printf("\t\t 请选择菜单项(0-4):");
scanf("%d", &k);
switch(k) {
case 1://随机产生数据
printf("请输入要产生随机数的个数(n<=%d)n= ",size);
scanf("%d",&n);
srand((unsigned)time(NULL));
for (i = 1; i <= n; i++) {
A.rec[i] = R.rec[i] = rand();
}
A.n = R.n = n;
break;
case 2://顺序查找
printf("请输入要查找的关键字:");
scanf("%ld",&x);
SeqSearch(R,x,i,j);
if (i == 0) {
printf("没有此数据!!!");
} else {
printf("在 %d 个下标,第 %d 次找到。", i, j);
}
break;
case 3://二分查找
sort(A,n);
print(A,n);
printf("请输入要查找的关键字:");
scanf("%ld",&x);
j = 0;
i = BinSearch(A,x,j);
if (i == 0) {
printf("没有此数据!!!");
} else {
printf("在 %d 个下标,第 %d 次找到。", i, j);
}
break;
case 4:
print(A,n);
}
} while (k != 0);
}
```
阅读全文
相关推荐
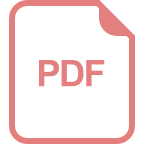
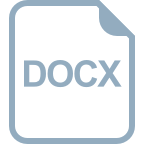
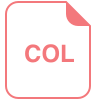












