可以提供一个C语言OpenGL编写的贪吃蛇代码吗
时间: 2024-02-06 16:10:14 浏览: 24
当然可以,以下是一个简单的基于OpenGL和C语言的贪吃蛇游戏代码:
```c
#include <GL/glut.h>
#include <stdlib.h>
#include <time.h>
#define ROWS 25
#define COLS 25
#define FPS 10
int snake_length = 1;
int snake_pos_x[ROWS * COLS] = {0};
int snake_pos_y[ROWS * COLS] = {0};
int food_x = 0, food_y = 0;
int direction = GLUT_KEY_RIGHT;
int game_over = 0;
int score = 0;
void init(void)
{
glClearColor(1.0, 1.0, 1.0, 0.0);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
gluOrtho2D(0, COLS, 0, ROWS);
srand(time(NULL));
food_x = rand() % COLS;
food_y = rand() % ROWS;
}
void draw_snake(void)
{
glColor3f(0.0, 0.0, 1.0); // Snake color is blue
for (int i = 0; i < snake_length; i++) {
glRecti(snake_pos_x[i], snake_pos_y[i], snake_pos_x[i]+1, snake_pos_y[i]+1);
}
}
void draw_food(void)
{
glColor3f(1.0, 0.0, 0.0); // Food color is red
glRecti(food_x, food_y, food_x+1, food_y+1);
}
void display(void)
{
glClear(GL_COLOR_BUFFER_BIT);
draw_snake();
draw_food();
glutSwapBuffers();
}
void update(void)
{
if (game_over) {
return;
}
// Move the snake
for (int i = snake_length - 1; i > 0; i--) {
snake_pos_x[i] = snake_pos_x[i-1];
snake_pos_y[i] = snake_pos_y[i-1];
}
switch(direction) {
case GLUT_KEY_UP:
snake_pos_y[0]++;
break;
case GLUT_KEY_DOWN:
snake_pos_y[0]--;
break;
case GLUT_KEY_LEFT:
snake_pos_x[0]--;
break;
case GLUT_KEY_RIGHT:
snake_pos_x[0]++;
break;
}
// Check if snake collides with food
if (snake_pos_x[0] == food_x && snake_pos_y[0] == food_y) {
snake_length++;
score++;
food_x = rand() % COLS;
food_y = rand() % ROWS;
}
// Check if snake collides with itself
for (int i = 1; i < snake_length; i++) {
if (snake_pos_x[0] == snake_pos_x[i] &&
snake_pos_y[0] == snake_pos_y[i]) {
game_over = 1;
break;
}
}
// Check if snake collides with walls
if (snake_pos_x[0] < 0 || snake_pos_x[0] >= COLS ||
snake_pos_y[0] < 0 || snake_pos_y[0] >= ROWS) {
game_over = 1;
}
glutPostRedisplay();
}
void keyboard(int key, int x, int y)
{
switch(key) {
case GLUT_KEY_UP:
case GLUT_KEY_DOWN:
case GLUT_KEY_LEFT:
case GLUT_KEY_RIGHT:
if (abs(direction - key) != 2) {
direction = key;
}
break;
}
}
int main(int argc, char* argv[])
{
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_RGB | GLUT_DOUBLE);
glutInitWindowSize(COLS * 20, ROWS * 20);
glutCreateWindow("Snake Game");
glutDisplayFunc(display);
glutIdleFunc(update);
glutSpecialFunc(keyboard);
init();
glutMainLoop();
return 0;
}
```
这段代码使用了OpenGL和GLUT库来实现图形界面,通过键盘控制蛇的移动。同时,它也包含了游戏结束、得分统计、食物生成、蛇的移动等游戏逻辑。同时,代码中也考虑了蛇与边界和自身的碰撞检测。
相关推荐
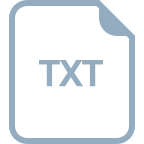












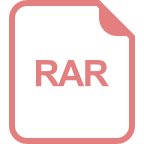