用Java语言写一个五子棋游戏
时间: 2023-07-20 21:12:49 浏览: 103
好的,以下是一个简单的五子棋游戏Java代码示例,供您参考:
```
import java.util.Scanner;
public class FiveInRow {
Scanner scanner = new Scanner(System.in);
char[][] board = new char[15][15];
char player = 'X';
public void initializeBoard() {
for (int i = 0; i < 15; i++) {
for (int j = 0; j < 15; j++) {
board[i][j] = '-';
}
}
}
public void displayBoard() {
System.out.println(" 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15");
for (int i = 0; i < 15; i++) {
System.out.print((i + 1) + " ");
for (int j = 0; j < 15; j++) {
System.out.print(board[i][j] + " ");
}
System.out.println();
}
}
public void play() {
initializeBoard();
displayBoard();
while (true) {
System.out.println("Player " + player + " please enter your move (row[1-15] column[1-15]):");
int row = scanner.nextInt() - 1;
int col = scanner.nextInt() - 1;
if (row < 0 || row > 14 || col < 0 || col > 14) {
System.out.println("Invalid move, please try again.");
continue;
}
if (board[row][col] != '-') {
System.out.println("This place is already taken, please try again.");
continue;
}
board[row][col] = player;
displayBoard();
if (checkWin(row, col)) {
System.out.println("Player " + player + " wins!");
break;
}
if (isDraw()) {
System.out.println("Draw!");
break;
}
player = (player == 'X') ? 'O' : 'X';
}
scanner.close();
}
public boolean checkWin(int row, int col) {
// check row
int count = 0;
for (int i = 0; i < 15; i++) {
if (board[row][i] == player) {
count++;
} else {
count = 0;
}
if (count == 5) {
return true;
}
}
// check column
count = 0;
for (int i = 0; i < 15; i++) {
if (board[i][col] == player) {
count++;
} else {
count = 0;
}
if (count == 5) {
return true;
}
}
// check diagonal
count = 0;
for (int i = -4; i <= 4; i++) {
int r = row + i;
int c = col + i;
if (r < 0 || r > 14 || c < 0 || c > 14) {
continue;
}
if (board[r][c] == player) {
count++;
} else {
count = 0;
}
if (count == 5) {
return true;
}
}
// check anti-diagonal
count = 0;
for (int i = -4; i <= 4; i++) {
int r = row - i;
int c = col + i;
if (r < 0 || r > 14 || c < 0 || c > 14) {
continue;
}
if (board[r][c] == player) {
count++;
} else {
count = 0;
}
if (count == 5) {
return true;
}
}
return false;
}
public boolean isDraw() {
for (int i = 0; i < 15; i++) {
for (int j = 0; j < 15; j++) {
if (board[i][j] == '-') {
return false;
}
}
}
return true;
}
public static void main(String[] args) {
FiveInRow game = new FiveInRow();
game.play();
}
}
```
该代码实现了一个简单的五子棋游戏,玩家可以交替落子,当有任意一方连成五个棋子时游戏结束。希望对您有所帮助。
阅读全文
相关推荐







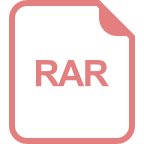








