python如何取resnet18前五层
时间: 2023-10-18 13:18:44 浏览: 115
Python 中可以使用 PyTorch 框架来访问 ResNet 模型中的层。以下是获取 ResNet18 模型前五层的示例代码:
```python
import torch
import torchvision.models as models
resnet18 = models.resnet18(pretrained=True)
layers = list(resnet18.children())[0:5]
print(layers)
```
该代码将输出第 1-5 层的内容。
相关问题
python resnet50 网络结构代码
resnet50是一种非常流行的深度学习网络结构,其在计算机视觉任务中取得了很好的效果。下面是使用Python编写的resnet50网络结构的代码示例:
```python
import tensorflow as tf
from tensorflow.keras import layers
def create_resnet50():
# 输入层
input_shape = (224, 224, 3)
inputs = tf.keras.Input(shape=input_shape)
# 第一段
x = layers.Conv2D(64, 7, strides=2, padding='same')(inputs)
x = layers.BatchNormalization()(x)
x = layers.Activation('relu')(x)
x = layers.MaxPooling2D(pool_size=3, strides=2, padding='same')(x)
# 第二段
x = conv_block(x, filters=[64, 64, 256], strides=1, block_name='2a')
x = identity_block(x, filters=[64, 64, 256], block_name='2b')
x = identity_block(x, filters=[64, 64, 256], block_name='2c')
# 第三段
x = conv_block(x, filters=[128, 128, 512], strides=2, block_name='3a')
x = identity_block(x, filters=[128, 128, 512], block_name='3b')
x = identity_block(x, filters=[128, 128, 512], block_name='3c')
x = identity_block(x, filters=[128, 128, 512], block_name='3d')
# 第四段
x = conv_block(x, filters=[256, 256, 1024], strides=2, block_name='4a')
x = identity_block(x, filters=[256, 256, 1024], block_name='4b')
x = identity_block(x, filters=[256, 256, 1024], block_name='4c')
x = identity_block(x, filters=[256, 256, 1024], block_name='4d')
x = identity_block(x, filters=[256, 256, 1024], block_name='4e')
x = identity_block(x, filters=[256, 256, 1024], block_name='4f')
# 第五段
x = conv_block(x, filters=[512, 512, 2048], strides=2, block_name='5a')
x = identity_block(x, filters=[512, 512, 2048], block_name='5b')
x = identity_block(x, filters=[512, 512, 2048], block_name='5c')
# 平均池化层
x = layers.GlobalAveragePooling2D()(x)
# 全连接层
x = layers.Dense(1000, activation='softmax')(x)
# 构建模型
model = tf.keras.Model(inputs=inputs, outputs=x)
return model
def conv_block(input_tensor, filters, strides, block_name):
filters1, filters2, filters3 = filters
x = layers.Conv2D(filters1, 1, strides=strides, name=block_name + '_conv1')(input_tensor)
x = layers.BatchNormalization(name=block_name + '_bn1')(x)
x = layers.Activation('relu')(x)
x = layers.Conv2D(filters2, 3, padding='same', name=block_name + '_conv2')(x)
x = layers.BatchNormalization(name=block_name + '_bn2')(x)
x = layers.Activation('relu')(x)
x = layers.Conv2D(filters3, 1, name=block_name + '_conv3')(x)
x = layers.BatchNormalization(name=block_name + '_bn3')(x)
shortcut = layers.Conv2D(filters3, 1, strides=strides, name=block_name + '_shortcut')(input_tensor)
shortcut = layers.BatchNormalization(name=block_name + '_bn_shortcut')(shortcut)
x = layers.add([x, shortcut])
x = layers.Activation('relu')(x)
return x
def identity_block(input_tensor, filters, block_name):
filters1, filters2, filters3 = filters
x = layers.Conv2D(filters1, 1, name=block_name + '_conv1')(input_tensor)
x = layers.BatchNormalization(name=block_name + '_bn1')(x)
x = layers.Activation('relu')(x)
x = layers.Conv2D(filters2, 3, padding='same', name=block_name + '_conv2')(x)
x = layers.BatchNormalization(name=block_name + '_bn2')(x)
x = layers.Activation('relu')(x)
x = layers.Conv2D(filters3, 1, name=block_name + '_conv3')(x)
x = layers.BatchNormalization(name=block_name + '_bn3')(x)
x = layers.add([x, input_tensor])
x = layers.Activation('relu')(x)
return x
# 创建ResNet50模型
model = create_resnet50()
```
以上是创建resnet50网络结构的代码示例,使用了tensorflow和keras库。这段代码定义了resnet50网络的每一层的连接方式和操作,包括卷积层、批归一化层、激活函数等。最后通过调用`create_resnet50()`函数创建一个resnet50的模型对象。
resnet101python
ResNet101是一个深度残差网络模型,用于图像分类任务。它由多个卷积层和池化层组成,以及一个全连接层。\[1\]在模型的构造函数中,定义了模型的结构,包括卷积层、批归一化层、ReLU激活函数和最大池化层等。在前向传播函数中,定义了模型的前向传播过程,包括卷积、批归一化、ReLU激活和池化等操作。\[1\]
在实例化模型时,可以使用ResNet101类来创建一个ResNet101的实例。\[1\]
在训练过程中,可以使用SoftmaxCrossEntropyWithLogits作为损失函数,该函数可以计算模型输出与标签之间的交叉熵损失。同时,可以使用动量优化器Momentum来更新模型的可训练参数,以最小化损失函数。\[2\]
ResNet101的网络结构主要由卷积和池化层组成。在101层的ResNet中,有五个卷积层和一个全连接层。在第一个卷积层后,还有一个池化层。整个网络的结构设计了残差连接,这是为了解决深度网络训练中的梯度消失问题。\[3\]
总结来说,ResNet101是一个深度残差网络模型,用于图像分类任务。它由多个卷积层和池化层组成,以及一个全连接层。在训练过程中,可以使用SoftmaxCrossEntropyWithLogits作为损失函数,使用Momentum优化器来更新模型参数。\[1\]\[2\]\[3\]
#### 引用[.reference_title]
- *1* *2* [在MindSpore中,可以使用ResNet类实现ResNet101模型。 你写一个示例代码,展示如何使用MindSp...](https://blog.csdn.net/weixin_42603332/article/details/129564301)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insertT0,239^v3^insert_chatgpt"}} ] [.reference_item]
- *3* [用Python手把手教你理解Resnet的运行过程(全代码注释版,基于paddle)](https://blog.csdn.net/qq_54504522/article/details/124415557)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insertT0,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
阅读全文
相关推荐
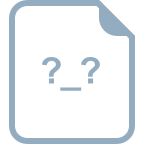
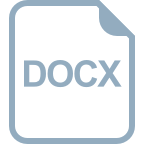
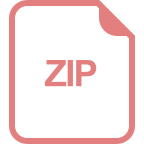
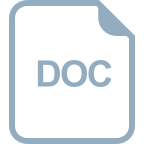
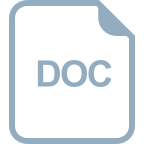
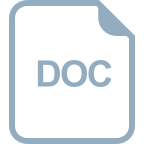
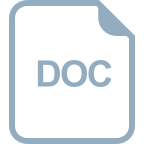
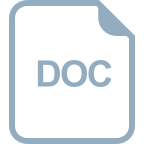
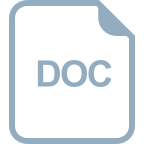
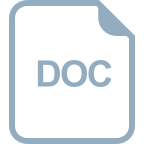
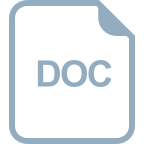
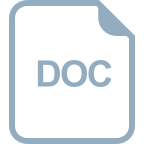




